have some questions about java programming, im stuck on a couple things. I will attatch what I've got so far but here is what I have to do, Create a project named JavaReview. Use a package name of edu.seminolestate.review. You can substitute your domain name for edu.seminolestate if you wish. Create a single class named ReviewApplication. When the application starts, prompt the user to enter 5 floating point numbers. These are numbers that can contain a decimal. You do not need to edit these numbers. Your professor will only enter valid decimal numbers when testing your application. You must store these numbers in an array. Display a menu with five options. These options are add, subtract, multiply, divide, and quit. Below is a sample of the menu. Enter a to add. Enter s to subtract. Enter m to multiply. Enter d to divide. Enter q to quit. Add all the elements in the array when the add option is selected. Display the final sum using System.out. Subtract each element (elements 2 through 5) in the array from the first element when the subtract option is selected. Display the final result using System.out. Multiply each element in the array when the multiply option is selected. Display the final product using System.out. Divide each (elements 2 through 5) in the array into the first element when the divide option is specified. Display the final product using System.out. End the application when the quit option is selected. Display an error message if the user enters any character other than a, s, m, d, or q. Continue to display the menu and process user selections until the user enters the quit option. Display a closing message before ending the application. Let me know what i am missing. Also how do I display a message and exit when the user enters "Q"? Here is what i've got so far import java.util.Scanner; public class ReviewApplication { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); double sum = 0; double difference = 0; double product = 0; double divide = 0; boolean looper = true; double[] array = new double[5]; String choice = processMenu(); boolean continueToRun = true; System.out.print(" Enter 5 floating point numbers "); for (int i = 0; i < array.length; i++){ array[i] = keyboard.nextDouble(); sum = sum + array[i]; } System.out.println(sum); processMenu(); switch (choice) { case 1: for (int i = 0; i < array.length; i++){ array[i] = keyboard.nextDouble(); sum = sum + array[i]; } System.out.println(sum); break; case 2: for (int i = 0; i < array.length; i++){ array[i] = keyboard.nextDouble(); difference = difference - array[i];} System.out.println("the difference is" + difference); break; case 3: for (int i = 0; i < array.length; i++){ array[i] = keyboard.nextDouble(); product = product * array[i];} System.out.println("the product is" + product); break; case 4: double total4 = 0; for (int i = 0; i < array.length; i++){ total4 /= array[i];} System.out.println("the answer is" + total4); } break; case 5:System.out.println("enter q to exit"); keyboard.nextLine(); if input ='q'; System.exit; break; while(looper); System.out.println(); System.out.println("Thanks for playing!"); keyboard.close();} } // end of main public static String processMenu( ){ Scanner keyboard = new Scanner(System.in); String response = keyboard.nextLine(); do { System.out.println("Enter a to add numbers"); System.out.println("Enter s to subtract"); System.out.println("Enter m to multiply"); System.out.println("Enter d to divide"); System.out.println("Enter q to exit"); System.out.print(">>> "); response = keyboard.nextLine(); // keyboard.nextLine(); //clear enter key from keyboard buffer if (response != "a" || response != "s" || response != "m" || response != "d"|| response != "q") { System.out.println("Invalid menu slection. Try again."); } }while (response == "a" || response == "s" || response == "m" || response == "d"|| response == "q"); return response; } }
I have some questions about java programming, im stuck on a couple things. I will attatch what I've got so far but here is what I have to do,
- Create a project named JavaReview. Use a package name of edu.seminolestate.review. You can substitute your domain name for edu.seminolestate if you wish. Create a single class named ReviewApplication.
- When the application starts, prompt the user to enter 5 floating point numbers. These are numbers that can contain a decimal. You do not need to edit these numbers. Your professor will only enter valid decimal numbers when testing your application. You must store these numbers in an array.
- Display a menu with five options. These options are add, subtract, multiply, divide, and quit. Below is a sample of the menu.
Enter a to add. |
Enter s to subtract. |
Enter m to multiply. |
Enter d to divide. |
Enter q to quit. |
- Add all the elements in the array when the add option is selected. Display the final sum using System.out.
- Subtract each element (elements 2 through 5) in the array from the first element when the subtract option is selected. Display the final result using System.out.
- Multiply each element in the array when the multiply option is selected. Display the final product using System.out.
- Divide each (elements 2 through 5) in the array into the first element when the divide option is specified. Display the final product using System.out.
- End the application when the quit option is selected.
- Display an error message if the user enters any character other than a, s, m, d, or q.
- Continue to display the menu and process user selections until the user enters the quit option. Display a closing message before ending the application.
Let me know what i am missing. Also how do I display a message and exit when the user enters "Q"?
Here is what i've got so far
import java.util.Scanner;
public class ReviewApplication {
public static void main(String[] args) {
Scanner keyboard = new Scanner(System.in);
double sum = 0;
double difference = 0;
double product = 0;
double divide = 0;
boolean looper = true;
double[] array = new double[5];
String choice = processMenu();
boolean continueToRun = true;
System.out.print(" Enter 5 floating point numbers ");
for (int i = 0; i < array.length; i++){
array[i] = keyboard.nextDouble();
sum = sum + array[i];
}
System.out.println(sum);
processMenu();
switch (choice) {
case 1:
for (int i = 0; i < array.length; i++){
array[i] = keyboard.nextDouble();
sum = sum + array[i];
}
System.out.println(sum);
break;
case 2:
for (int i = 0; i < array.length; i++){
array[i] = keyboard.nextDouble();
difference = difference - array[i];}
System.out.println("the difference is" + difference);
break;
case 3:
for (int i = 0; i < array.length; i++){
array[i] = keyboard.nextDouble();
product = product * array[i];}
System.out.println("the product is" + product);
break;
case 4:
double total4 = 0;
for (int i = 0; i < array.length; i++){
total4 /= array[i];}
System.out.println("the answer is" + total4);
}
break;
case 5:System.out.println("enter q to exit");
keyboard.nextLine();
if input ='q'; System.exit;
break;
while(looper); System.out.println();
System.out.println("Thanks for playing!");
keyboard.close();}
} // end of main
public static String processMenu( ){
Scanner keyboard = new Scanner(System.in);
String response = keyboard.nextLine();
do {
System.out.println("Enter a to add numbers");
System.out.println("Enter s to subtract");
System.out.println("Enter m to multiply");
System.out.println("Enter d to divide");
System.out.println("Enter q to exit");
System.out.print(">>> ");
response = keyboard.nextLine();
// keyboard.nextLine(); //clear enter key from keyboard buffer
if (response != "a" || response != "s" || response != "m" ||
response != "d"|| response != "q") {
System.out.println("Invalid menu slection. Try again.");
}
}while (response == "a" || response == "s" || response == "m" ||
response == "d"|| response == "q");
return response;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

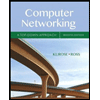
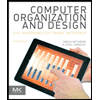
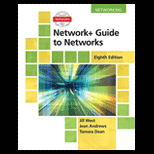
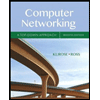
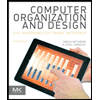
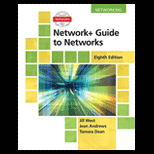
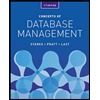
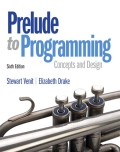
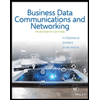