Solve this question using java language. you have to read the information from a file then print them in a file please read the instructour carefully, also look at the attach images to help you to solve it. This tips will help you What You Must Implement: You must define a class named MediaRental that implements the MediaRentalInt interface functionality (index A). You must define classes that support the functionality specified by the interface. The following specifications are associated with the project: 1. Define a class named MediaRental. Feel free to add any instance variables you understand are needed or any private methods. Do not add any public methods (beyond the ones specified in the MediaRentalInt interface). 2. The media rental system keeps track of customers and media (movies ,music albums and games). A customer has a name, address as string , a plan and two lists. One list represent the media the customer is interested in receiving and the second one represents the media already received (rented) by the customer. There are two plans a customer can have: UNLIMITED and LIMITED. UNLIMITED allows a customer to receive as many media as they want; LIMITED restricts the media to a default value of 2 (this value can be change via a media rental class method). A movie has a title, a number of copies available and a rating (e.g., "HR"). An album has a title, number of copies available, an artist and the songs that are part of the album. A game has title,number of copies,and the weight (in grams) is also stored. 3. You must define and use at least four classes (not including MediaRental) as part of your design. At least two of those classes must be in a superclass/subclass relationship (Inheritance Requirement). The other two can be defined as you wish. Feel free to define as many classes as you want. These classes must support the functionality of the system otherwise you will not receive any credit. 4. One of your classes must define an equals method that has as parameter an Object parameter. 5. The database for your system needs to be represented using two ArrayList objects. One ArrayList will represent the customers present in the database; the second will represent the media (movies, albums , and games). 6. Regarding the searchMedia method: the songs parameter represents a substring (fragment) or the full list of songs associated with the album. If the full list is provided you can assume commas will be part of the string. Hint: you may want to consider using the indexOf method of the String class. 7. Your program should store the results in a file between executions of the program, so that when the program is run again it will start up with the same inventory contents as when it last terminated. 8. Feel free to use Collections.sort to sort your data. 9. Write test driver. 10. Handle expectations where it’s needed. 11.Not all the details associated with the project can be fully specified in this description. The sooner you start working on the project the sooner you will be able to address any doubts you may have. Note: To understand the requirement, you might lock at the indices (A and B)
Solve this question using java language. you have to read the information from a file then print them in a file please read the instructour carefully, also look at the attach images to help you to solve it.
This tips will help you
What You Must Implement:
You must define a class named MediaRental that implements the MediaRentalInt interface functionality
(index A). You must define classes that support the functionality specified by the interface. The following
specifications are associated with the project:
1. Define a class named MediaRental. Feel free to add any instance variables you understand are needed or any
private methods. Do not add any public methods (beyond the ones specified in the MediaRentalInt interface).
2. The media rental system keeps track of customers and media (movies ,music albums and games). A customer
has a name, address as string , a plan and two lists. One list represent the media the customer is interested in
receiving and the second one represents the media already received (rented) by the customer. There are two
plans a customer can have: UNLIMITED and LIMITED. UNLIMITED allows a customer to receive as many media
as they want; LIMITED restricts the media to a default value of 2 (this value can be change via a media rental
class method).
A movie has a title, a number of copies available and a rating (e.g., "HR"). An album has a title, number of
copies available, an artist and the songs that are part of the album. A game has title,number of copies,and the
weight (in grams) is also stored.
3. You must define and use at least four classes (not including MediaRental) as part of your design. At least two of
those classes must be in a superclass/subclass relationship (Inheritance Requirement). The other two can be
defined as you wish. Feel free to define as many classes as you want. These classes must support the
functionality of the system otherwise you will not receive any credit.
4. One of your classes must define an equals method that has as parameter an Object parameter.
5. The
the customers present in the database; the second will represent the media (movies, albums , and games).
6. Regarding the searchMedia method: the songs parameter represents a substring (fragment) or the full list of
songs associated with the album. If the full list is provided you can assume commas will be part of the string.
Hint: you may want to consider using the indexOf method of the String class.
7. Your program should store the results in a file between executions of the program, so
that when the program is run again it will start up with the same inventory contents as
when it last terminated.
8. Feel free to use Collections.sort to sort your data.
9. Write test driver.
10. Handle expectations where it’s needed.
11.Not all the details associated with the project can be fully specified in this description. The sooner you start
working on the project the sooner you will be able to address any doubts you may have.
Note: To understand the requirement, you might lock at the indices (A and B)
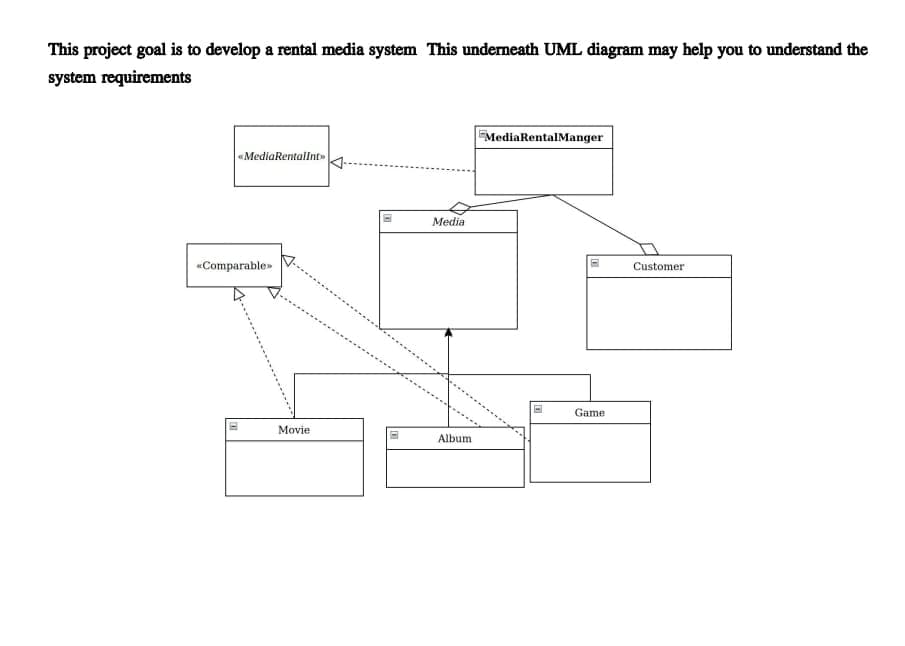
![Index A:
MediaRentallnt interfoce Includes:
Method Detail
addCustomer
void addCustomer (String name, String address, String plan)
Adds the specified custoner to the database. The address is a physical address (not e nail). The plan options avai lable
are: LIMITED and UNLIMITED. LIMITED defines a default naximum of two media that can be rented.
Parameters: name - , address -, plan -
addMovie
void addMovie (String title, int copiesAvailable, String rating)
Adda the specified aovie to the database, The possible values for rating are "DR", "HR", "AC".
Parameters:title - , copiesAvailnble , rating
addGame
void addGeme (String title, int copiesAvni lable, dotuble weight)
Adds the specified game to the database.
Parameters:title - , copiesAvailable -, weight
addalbum
void addAlbun (String title, int copiesAvai lable, String artist, String songs)
Adds the specified album to the database. The songs String includes a list of the title of songs in the album (song
titles are separated by comas).
Paraneters: title - , copiesávailable -, artist -, songa -
setlimitedPlaniinit
void setlinitedPlanLimit (int value)
This set the nunber of media ansociated vith the LIMITED plan.
Parameters: value
getallCuntomeralnfo
String getAllCustoneraInfo()
Returns information about the customers in the database. The infornation is presented sorted by custoner name.
Returna:
getAllMedialnfo
String getAllMediainfo 0
Returns information about all the nedia (novies, albums, and ganes) that are part of the database. The information
is presented sorted by media title.
Returna:
addToCart
boolean addToCart (String cust onerName, String mediaTitle)
Adds the specified nedia title to the cart associated with a custoner.
Paraneters:customer Nane - , mediaTitle -
Returna: false if the medialitle is already part of the cart (it will not be added)
renovePronCart
boolean renovePronCart (String custonerNane,
String mediaTitle)
Removes the specified media title from the customer's cart.
Parameters:customerNane - , mediaTitle
Returns: false if renoval failed for any reason (e. g., custOner Nane not found)
processkequests
String procesaRequesta 0
Processes the requests cart of each customer. The customers wi11 be processed in alphabetical order. For each customer,
the requests cart will be checked and media vill be added to the rented cart, if the plan associated vith the customer
allows it, and if there is a copy of the media avai lable. For UNLIMITED plans the media will be added to the rented
cart always, as long as there are copies associated vith the nedia available. For LIMITED plans, the number of entries
noved from the requests cart to the rented cart vill depend on the nunber of current ly rented media, and whether copies
associated with the nedia are available.
For each nedia that is rented, the following message will be generated:
"Sending ImedialTitlel to (customerNane]"
Returns:
returnledia
boolean returnMedia (String customer Name, String mediaTitle)
This is hov a customer returns a rented nedia. This nethod will renove the iten from the rented cart and adjust any
other values that are necessary (e. g.. copiendvai lable)
Paramoters:customerNane - , mediaTitle -
Returne:
senrchMedia
Arraylist<String searchledia (String title, String rating. String artist, String songs)
Returns a SORTED Arraylist with medin titles that satisfy the provided paraneter values. If mull is specified for a
paraneter, then that paraneter should be ignore in the search. Providing null for all paraneters will return all media
titles.
Paraneters:
title - ,rating - ,artist -, songs
Returns:
Index B:
Public Driver:
Method Detail
publie void testAddingCustomers 0
public void testAddingledia ()
public void testingAddingToCart 0
public void testingRemovingFroeCart 0
publie void testProcessingRequestsone 0
publie void testProcessingRequestsTvo 0
public void testReturnledia ()
publie void testSearchledia )](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffc03ea1b-3a89-4a24-aa91-9907626e940e%2Feaa569da-e5d1-49b0-8933-b2580c23cda6%2Fvhiblz_processed.jpeg&w=3840&q=75)

Step by step
Solved in 2 steps

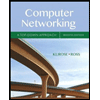
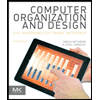
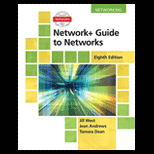
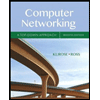
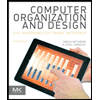
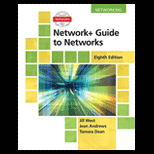
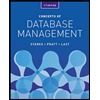
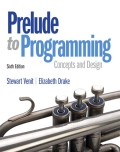
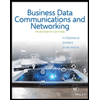