PLEASE HELP ME! ? Maximize and use alternative method to this code! package com.btech.pf101; import java.io.bufferedreader; import java.io.inputstreamreader; import java.util.calendar; import java.util.date; public class pawnshopcode { private static final bufferedreader br = new bufferedreader(new inputstreamreader(system.in)); private static string name; private static int age; private static string address; private static string contactno; private static int itemtype; private static string itemtypename; private static final int itemtype_gagdet = 1; private static final int itemtype_jewelry = 2; private static final int itemtype_musicinstrument = 3; private static final int itemtype_homeequipment = 4; private static final int itemtype_landtitle = 5; private static string itemdescription; private static int periodtype; private static string periodtypename; private static final int periodtype_days = 1; private static final int periodtype_weeks = 2; private static final int periodtype_months = 3; private static final int periodtype_years = 4; private static int noofperiods; private static double pawnamount; private static double interestamount; private static date transactiondate; private static date redemptiondate; private static date terminationdate; private static boolean check; public static void main(string[] args) { try { //display name and short greeting. system.out.println("my pawnshop"); system.out.println("welcome client. if you wish to pawn an item, kindly input some of your information."); system.out.println(); //ask for client information check = false; while (!check) { system.out.println("please enter your name: "); name = br.readline(); if (name.equalsignorecase("")) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } check = false; while (!check) { system.out.println("please enter your age: "); try { age = integer.parseint(br.readline()); if (age < 18) { system.out.println("you are not yet in a legitimate age. please try again."); } else { check = true; } system.out.println(); } catch (exception e) { system.out.println("you have entered an invalid input. please try again."); } } check = false; while (!check) { system.out.println("please enter your address: "); address = br.readline(); if (address.equalsignorecase("")) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } check = false; while (!check) { system.out.println("please enter your contact number: "); contactno = br.readline(); if (contactno.equalsignorecase("")) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } //ask for item to be pawned check = false; while (!check) { system.out.println("please select type of item be pawned [1] gadget [2] jewelry [3] musical instrument [4] home equipment [5] land title :"); try { itemtype = integer.parseint(br.readline()); if (itemtype < 1 || itemtype > 5) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } catch (exception e) { system.out.println("you have entered an invalid input. please try again."); } } check = false; while (!check) { system.out.println("please enter short description of item to be pawned: "); itemdescription = br.readline(); if (itemdescription.equalsignorecase("")) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } //ask for pawn period information check = false; while (!check) { system.out.println("please select period type [1] days [2] weeks [3] months [4] years :"); try { periodtype = integer.parseint(br.readline()); if (periodtype < 1 || periodtype > 4) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } catch (exception e) { system.out.println("you have entered an invalid input. please try again."); } } check = false; while (!check) { system.out.println("please select number of periods to pay pawned item: "); try { noofperiods = integer.parseint(br.readline()); if (noofperiods < 1) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } catch (exception e) { system.out.println("you have entered an invalid input. please try again."); } } //ask for pawn amount from client //display minimums and maximums per item type based on chosen item type check = false; while (!check) { system.out.println("please select desired pawn amount: "); try { switch (itemtype) { case itemtype_gagdet: system.out.println("min: 5000 max: 10000"); pawnamount = double.parsedouble(br.readline()); if (pawnamount < 5000.00d || pawnamount > 10000.00d ) { system.out.println("you have entered an invalid input. please try again."); } else { itemtypename = "gadget"; check = true; } break; case itemtype_jewelry: system.out.println("min: 5000 max: 20000"); pawnamount = double.parsedouble(br.readline()); if (pawnamount < 5000.00d || pawnamount > 20000.00d ) { system.out.println("you have entered an invalid input. please try again."); } else { itemtypename = "jewelry"; check = true; } break; case itemtype_musicinstrument: system.out.println("min: 2000 max: 5000"); pawnamount = double.parsedouble(br.readline()); if (pawnamount < 2000.00d || pawnamount > 5000.00d ) { system.out.println("you have entered an invalid input. please try again."); } else { itemtypename = "musical instrument"; check = true; } break; case itemtype_homeequipment: system.out.println("min: 3000 max: 10000"); pawnamount = double.parsedouble(br.readline()); if (pawnamount < 3000.00d || pawnamount > 10000.00d ) { system.out.println("you have entered an invalid input. please try again."); } else { itemtypename = "home equipment"; check = true; } break; case itemtype_landtitle: system.out.println("min: 25000 max: unlimited"); pawnamount = double.parsedouble(br.readline()); if (pawnamount < 25000.00d) { system.out.println("you have entered an invalid input. please try again."); } else { itemtypename = "land title"; check = true; } break; default: system.out.println("you have entered an invalid input. please try again."); } system.out.println(); } catch (exception e) { system.out.println("you have entered an invalid input. please try again."); } } //compute for interest amount to be charged switch(periodtype){ case periodtype_days: periodtypename = "days"; interestamount = pawnamount * (0.01d * noofperiods); break; case periodtype_weeks: periodtypename = "weeks"; interestamount = pawnamount * (0.05d * noofperiods); break; case periodtype_months: periodtypename = "months"; interestamount = pawnamount * (0.10d * noofperiods); break; case periodtype_years: periodtypename = "years"; interestamount = pawnamount * (0.12d * noofperiods); break; } //set the dates calendar c = calendar.getinstance(); transactiondate = c.gettime(); switch(periodtype){ case periodtype_days: c.add(calendar.date, noofperiods); redemptiondate = c.gettime(); ; break; case periodtype_weeks: c.add(calendar.week_of_year, noofperiods); redemptiondate = c.gettime(); ; break; case periodtype_months: c.add(calendar.month, noofperiods); redemptiondate = c.gettime(); ; break; case periodtype_years: c.add(calendar.year, noofperiods); redemptiondate = c.gettime(); ; break; } c.add(calendar.week_of_year, 1); terminationdate = c.gettime(); //display transaction as receipt system.out.println(); system.out.println("my pawnshop"); system.out.println("sample transaction receipt"); system.out.println(); system.out.println("name: " + name); system.out.println("age: " + age); system.out.println("address: " + address); system.out.println("contact no: " + contactno); system.out.println("item type: " + itemtypename); system.out.println("item description: " + itemdescription); system.out.println("period type: " + periodtypename); system.out.println("number of periods: " + noofperiods); system.out.println("pawn amount: " + string.format("%.2f", pawnamount)); system.out.println("interest charged: " + string.format("%.2f", interestamount)); system.out.println("total amount due: " + string.format("%.2f", pawnamount + interestamount)); system.out.println("date of transaction: " + transactiondate); system.out.println("date of redemption: " + redemptiondate); system.out.println("date of termination: " + terminationdate); system.out.println(); system.out.println("thank you!!!"); system.out.println(); } catch (exception e) { system.out.println(e.tostring()); system.out.println("error found while running program."); } } }
PLEASE HELP ME! ? Maximize and use alternative method to this code! package com.btech.pf101; import java.io.bufferedreader; import java.io.inputstreamreader; import java.util.calendar; import java.util.date; public class pawnshopcode { private static final bufferedreader br = new bufferedreader(new inputstreamreader(system.in)); private static string name; private static int age; private static string address; private static string contactno; private static int itemtype; private static string itemtypename; private static final int itemtype_gagdet = 1; private static final int itemtype_jewelry = 2; private static final int itemtype_musicinstrument = 3; private static final int itemtype_homeequipment = 4; private static final int itemtype_landtitle = 5; private static string itemdescription; private static int periodtype; private static string periodtypename; private static final int periodtype_days = 1; private static final int periodtype_weeks = 2; private static final int periodtype_months = 3; private static final int periodtype_years = 4; private static int noofperiods; private static double pawnamount; private static double interestamount; private static date transactiondate; private static date redemptiondate; private static date terminationdate; private static boolean check; public static void main(string[] args) { try { //display name and short greeting. system.out.println("my pawnshop"); system.out.println("welcome client. if you wish to pawn an item, kindly input some of your information."); system.out.println(); //ask for client information check = false; while (!check) { system.out.println("please enter your name: "); name = br.readline(); if (name.equalsignorecase("")) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } check = false; while (!check) { system.out.println("please enter your age: "); try { age = integer.parseint(br.readline()); if (age < 18) { system.out.println("you are not yet in a legitimate age. please try again."); } else { check = true; } system.out.println(); } catch (exception e) { system.out.println("you have entered an invalid input. please try again."); } } check = false; while (!check) { system.out.println("please enter your address: "); address = br.readline(); if (address.equalsignorecase("")) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } check = false; while (!check) { system.out.println("please enter your contact number: "); contactno = br.readline(); if (contactno.equalsignorecase("")) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } //ask for item to be pawned check = false; while (!check) { system.out.println("please select type of item be pawned [1] gadget [2] jewelry [3] musical instrument [4] home equipment [5] land title :"); try { itemtype = integer.parseint(br.readline()); if (itemtype < 1 || itemtype > 5) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } catch (exception e) { system.out.println("you have entered an invalid input. please try again."); } } check = false; while (!check) { system.out.println("please enter short description of item to be pawned: "); itemdescription = br.readline(); if (itemdescription.equalsignorecase("")) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } //ask for pawn period information check = false; while (!check) { system.out.println("please select period type [1] days [2] weeks [3] months [4] years :"); try { periodtype = integer.parseint(br.readline()); if (periodtype < 1 || periodtype > 4) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } catch (exception e) { system.out.println("you have entered an invalid input. please try again."); } } check = false; while (!check) { system.out.println("please select number of periods to pay pawned item: "); try { noofperiods = integer.parseint(br.readline()); if (noofperiods < 1) { system.out.println("you have entered an invalid input. please try again."); } else { check = true; } system.out.println(); } catch (exception e) { system.out.println("you have entered an invalid input. please try again."); } } //ask for pawn amount from client //display minimums and maximums per item type based on chosen item type check = false; while (!check) { system.out.println("please select desired pawn amount: "); try { switch (itemtype) { case itemtype_gagdet: system.out.println("min: 5000 max: 10000"); pawnamount = double.parsedouble(br.readline()); if (pawnamount < 5000.00d || pawnamount > 10000.00d ) { system.out.println("you have entered an invalid input. please try again."); } else { itemtypename = "gadget"; check = true; } break; case itemtype_jewelry: system.out.println("min: 5000 max: 20000"); pawnamount = double.parsedouble(br.readline()); if (pawnamount < 5000.00d || pawnamount > 20000.00d ) { system.out.println("you have entered an invalid input. please try again."); } else { itemtypename = "jewelry"; check = true; } break; case itemtype_musicinstrument: system.out.println("min: 2000 max: 5000"); pawnamount = double.parsedouble(br.readline()); if (pawnamount < 2000.00d || pawnamount > 5000.00d ) { system.out.println("you have entered an invalid input. please try again."); } else { itemtypename = "musical instrument"; check = true; } break; case itemtype_homeequipment: system.out.println("min: 3000 max: 10000"); pawnamount = double.parsedouble(br.readline()); if (pawnamount < 3000.00d || pawnamount > 10000.00d ) { system.out.println("you have entered an invalid input. please try again."); } else { itemtypename = "home equipment"; check = true; } break; case itemtype_landtitle: system.out.println("min: 25000 max: unlimited"); pawnamount = double.parsedouble(br.readline()); if (pawnamount < 25000.00d) { system.out.println("you have entered an invalid input. please try again."); } else { itemtypename = "land title"; check = true; } break; default: system.out.println("you have entered an invalid input. please try again."); } system.out.println(); } catch (exception e) { system.out.println("you have entered an invalid input. please try again."); } } //compute for interest amount to be charged switch(periodtype){ case periodtype_days: periodtypename = "days"; interestamount = pawnamount * (0.01d * noofperiods); break; case periodtype_weeks: periodtypename = "weeks"; interestamount = pawnamount * (0.05d * noofperiods); break; case periodtype_months: periodtypename = "months"; interestamount = pawnamount * (0.10d * noofperiods); break; case periodtype_years: periodtypename = "years"; interestamount = pawnamount * (0.12d * noofperiods); break; } //set the dates calendar c = calendar.getinstance(); transactiondate = c.gettime(); switch(periodtype){ case periodtype_days: c.add(calendar.date, noofperiods); redemptiondate = c.gettime(); ; break; case periodtype_weeks: c.add(calendar.week_of_year, noofperiods); redemptiondate = c.gettime(); ; break; case periodtype_months: c.add(calendar.month, noofperiods); redemptiondate = c.gettime(); ; break; case periodtype_years: c.add(calendar.year, noofperiods); redemptiondate = c.gettime(); ; break; } c.add(calendar.week_of_year, 1); terminationdate = c.gettime(); //display transaction as receipt system.out.println(); system.out.println("my pawnshop"); system.out.println("sample transaction receipt"); system.out.println(); system.out.println("name: " + name); system.out.println("age: " + age); system.out.println("address: " + address); system.out.println("contact no: " + contactno); system.out.println("item type: " + itemtypename); system.out.println("item description: " + itemdescription); system.out.println("period type: " + periodtypename); system.out.println("number of periods: " + noofperiods); system.out.println("pawn amount: " + string.format("%.2f", pawnamount)); system.out.println("interest charged: " + string.format("%.2f", interestamount)); system.out.println("total amount due: " + string.format("%.2f", pawnamount + interestamount)); system.out.println("date of transaction: " + transactiondate); system.out.println("date of redemption: " + redemptiondate); system.out.println("date of termination: " + terminationdate); system.out.println(); system.out.println("thank you!!!"); system.out.println(); } catch (exception e) { system.out.println(e.tostring()); system.out.println("error found while running program."); } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
PLEASE HELP ME! ?
Maximize and use alternative method to this code!
package com.btech.pf101;
import java.io.bufferedreader;
import java.io.inputstreamreader;
import java.util.calendar;
import java.util.date;
public class pawnshopcode {
private static final bufferedreader br = new bufferedreader(new inputstreamreader(system.in));
private static string name;
private static int age;
private static string address;
private static string contactno;
private static int itemtype;
private static string itemtypename;
private static final int itemtype_gagdet = 1;
private static final int itemtype_jewelry = 2;
private static final int itemtype_musicinstrument = 3;
private static final int itemtype_homeequipment = 4;
private static final int itemtype_landtitle = 5;
private static string itemdescription;
private static int periodtype;
private static string periodtypename;
private static final int periodtype_days = 1;
private static final int periodtype_weeks = 2;
private static final int periodtype_months = 3;
private static final int periodtype_years = 4;
private static int noofperiods;
private static double pawnamount;
private static double interestamount;
private static date transactiondate;
private static date redemptiondate;
private static date terminationdate;
private static boolean check;
public static void main(string[] args) {
try {
//display name and short greeting.
system.out.println("my pawnshop");
system.out.println("welcome client. if you wish to pawn an item, kindly input some of your information.");
system.out.println();
//ask for client information
check = false;
while (!check) {
system.out.println("please enter your name: ");
name = br.readline();
if (name.equalsignorecase("")) {
system.out.println("you have entered an invalid input. please try again.");
} else {
check = true;
}
system.out.println();
}
check = false;
while (!check) {
system.out.println("please enter your age: ");
try {
age = integer.parseint(br.readline());
if (age < 18) {
system.out.println("you are not yet in a legitimate age. please try again.");
} else {
check = true;
}
system.out.println();
} catch (exception e) {
system.out.println("you have entered an invalid input. please try again.");
}
}
check = false;
while (!check) {
system.out.println("please enter your address: ");
address = br.readline();
if (address.equalsignorecase("")) {
system.out.println("you have entered an invalid input. please try again.");
} else {
check = true;
}
system.out.println();
}
check = false;
while (!check) {
system.out.println("please enter your contact number: ");
contactno = br.readline();
if (contactno.equalsignorecase("")) {
system.out.println("you have entered an invalid input. please try again.");
} else {
check = true;
}
system.out.println();
}
//ask for item to be pawned
check = false;
while (!check) {
system.out.println("please select type of item be pawned [1] gadget [2] jewelry [3] musical instrument [4] home equipment [5] land title :");
try {
itemtype = integer.parseint(br.readline());
if (itemtype < 1 || itemtype > 5) {
system.out.println("you have entered an invalid input. please try again.");
} else {
check = true;
}
system.out.println();
} catch (exception e) {
system.out.println("you have entered an invalid input. please try again.");
}
}
check = false;
while (!check) {
system.out.println("please enter short description of item to be pawned: ");
itemdescription = br.readline();
if (itemdescription.equalsignorecase("")) {
system.out.println("you have entered an invalid input. please try again.");
} else {
check = true;
}
system.out.println();
}
//ask for pawn period information
check = false;
while (!check) {
system.out.println("please select period type [1] days [2] weeks [3] months [4] years :");
try {
periodtype = integer.parseint(br.readline());
if (periodtype < 1 || periodtype > 4) {
system.out.println("you have entered an invalid input. please try again.");
} else {
check = true;
}
system.out.println();
} catch (exception e) {
system.out.println("you have entered an invalid input. please try again.");
}
}
check = false;
while (!check) {
system.out.println("please select number of periods to pay pawned item: ");
try {
noofperiods = integer.parseint(br.readline());
if (noofperiods < 1) {
system.out.println("you have entered an invalid input. please try again.");
} else {
check = true;
}
system.out.println();
} catch (exception e) {
system.out.println("you have entered an invalid input. please try again.");
}
}
//ask for pawn amount from client
//display minimums and maximums per item type based on chosen item type
check = false;
while (!check) {
system.out.println("please select desired pawn amount: ");
try {
switch (itemtype) {
case itemtype_gagdet:
system.out.println("min: 5000 max: 10000");
pawnamount = double.parsedouble(br.readline());
if (pawnamount < 5000.00d || pawnamount > 10000.00d ) {
system.out.println("you have entered an invalid input. please try again.");
} else {
itemtypename = "gadget";
check = true;
}
break;
case itemtype_jewelry:
system.out.println("min: 5000 max: 20000");
pawnamount = double.parsedouble(br.readline());
if (pawnamount < 5000.00d || pawnamount > 20000.00d ) {
system.out.println("you have entered an invalid input. please try again.");
} else {
itemtypename = "jewelry";
check = true;
}
break;
case itemtype_musicinstrument:
system.out.println("min: 2000 max: 5000");
pawnamount = double.parsedouble(br.readline());
if (pawnamount < 2000.00d || pawnamount > 5000.00d ) {
system.out.println("you have entered an invalid input. please try again.");
} else {
itemtypename = "musical instrument";
check = true;
}
break;
case itemtype_homeequipment:
system.out.println("min: 3000 max: 10000");
pawnamount = double.parsedouble(br.readline());
if (pawnamount < 3000.00d || pawnamount > 10000.00d ) {
system.out.println("you have entered an invalid input. please try again.");
} else {
itemtypename = "home equipment";
check = true;
}
break;
case itemtype_landtitle:
system.out.println("min: 25000 max: unlimited");
pawnamount = double.parsedouble(br.readline());
if (pawnamount < 25000.00d) {
system.out.println("you have entered an invalid input. please try again.");
} else {
itemtypename = "land title";
check = true;
}
break;
default:
system.out.println("you have entered an invalid input. please try again.");
}
system.out.println();
} catch (exception e) {
system.out.println("you have entered an invalid input. please try again.");
}
}
//compute for interest amount to be charged
switch(periodtype){
case periodtype_days:
periodtypename = "days";
interestamount = pawnamount * (0.01d * noofperiods);
break;
case periodtype_weeks:
periodtypename = "weeks";
interestamount = pawnamount * (0.05d * noofperiods);
break;
case periodtype_months:
periodtypename = "months";
interestamount = pawnamount * (0.10d * noofperiods);
break;
case periodtype_years:
periodtypename = "years";
interestamount = pawnamount * (0.12d * noofperiods);
break;
}
//set the dates
calendar c = calendar.getinstance();
transactiondate = c.gettime();
switch(periodtype){
case periodtype_days:
c.add(calendar.date, noofperiods);
redemptiondate = c.gettime(); ;
break;
case periodtype_weeks:
c.add(calendar.week_of_year, noofperiods);
redemptiondate = c.gettime(); ;
break;
case periodtype_months:
c.add(calendar.month, noofperiods);
redemptiondate = c.gettime(); ;
break;
case periodtype_years:
c.add(calendar.year, noofperiods);
redemptiondate = c.gettime(); ;
break;
}
c.add(calendar.week_of_year, 1);
terminationdate = c.gettime();
//display transaction as receipt
system.out.println();
system.out.println("my pawnshop");
system.out.println("sample transaction receipt");
system.out.println();
system.out.println("name: " + name);
system.out.println("age: " + age);
system.out.println("address: " + address);
system.out.println("contact no: " + contactno);
system.out.println("item type: " + itemtypename);
system.out.println("item description: " + itemdescription);
system.out.println("period type: " + periodtypename);
system.out.println("number of periods: " + noofperiods);
system.out.println("pawn amount: " + string.format("%.2f", pawnamount));
system.out.println("interest charged: " + string.format("%.2f", interestamount));
system.out.println("total amount due: " + string.format("%.2f", pawnamount + interestamount));
system.out.println("date of transaction: " + transactiondate);
system.out.println("date of redemption: " + redemptiondate);
system.out.println("date of termination: " + terminationdate);
system.out.println();
system.out.println("thank you!!!");
system.out.println();
} catch (exception e) {
system.out.println(e.tostring());
system.out.println("error found while running program.");
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
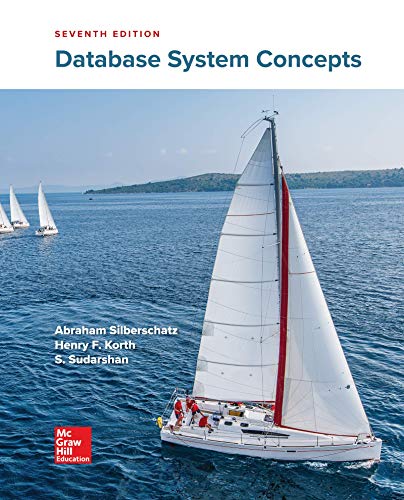
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
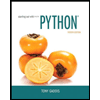
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
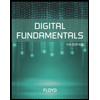
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
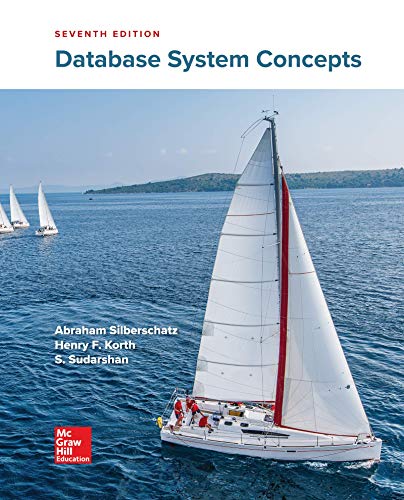
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
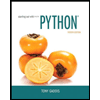
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
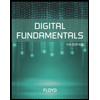
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
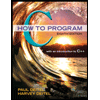
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
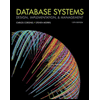
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
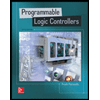
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education