he menu at a lunch counter includes a variety of sandwiches, salads, and drinks. The menu also allows a customer to create a “trio,” which consists of three menu items: a sandwich, a salad, and a drink. The price of the trio is the sum of the two highest-priced menu items in the trio; one item with the lowest price is free. Each menu item has a name and a price. The four types of menu items are represented by the four classes Sandwich, Salad, Drink, and Trio.
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
Question 1. The menu at a lunch counter includes a variety of sandwiches, salads, and drinks. The menu also allows a customer to create a “trio,” which consists of three menu items: a sandwich, a salad, and a drink. The price of the trio is the sum of the two highest-priced menu items in the trio; one item with the lowest price is free. Each menu item has a name and a price. The four types of menu items are represented by the four classes Sandwich, Salad, Drink, and Trio.
import java.text.*;
import java.util.*;
class SimpleLunchItem
{
private String name;
private double price;
public SimpleLunchItem(String aName, double aPrice)
{
name = aName;
price = aPrice;
}
public String getName() { return name; }
public double getPrice() { return price; }
public String toString ()
{
DecimalFormat money = new DecimalFormat("0.00");
return getName() + " " + money.format(getPrice());
}
}
class Drink extends SimpleLunchItem
{
public Drink(String name, double price)
{ super(name, price); }
}
class Salad extends SimpleLunchItem
{
public Salad(String name, double price)
{ super(name, price); }
}
class Sandwich extends SimpleLunchItem
{
public Sandwich(String name, double price)
{ super(name, price); }
}
// Declare the Trio class
// declare the instance variables that you need for a trio object
// write a constructor that takes a Sandwich, Salad, and a Drink, in that order
// write the getName method it should return sandwich name/salad name/drink name
// write the getPrice method it should return the sum of price of the two highest price items in the trio.
public static void main(String[] args)
{
Sandwich burger = new Sandwich("Cheeseburger",2.75);
Sandwich club = new Sandwich("Club Sandwich", 2.75);
Salad spinachSalad = new Salad("Spinach Salad",1.25);
Salad coleslaw = new Salad("Coleslaw", 1.25);
Drink orange = new Drink("Orange Soda", 1.25);
Drink cap = new Drink("Cappuccino", 3.50);
Trio trio1 = new Trio(burger,spinachSalad, orange);
System.out.println("It should print Cheeseburger/Spinach Salad/Orange Soda and it prints: " + trio1.getName());
System.out.println("It should print 4.0 and it prints: " + trio1.getPrice());
Trio trio2 = new Trio(club,coleslaw,cap);
System.out.println("It should print Club Sandwich/Coleslaw/Capuccino and it prints: " + trio2.getName());
System.out.println("It should print 6.25 and it prints: " + trio2.getPrice());
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

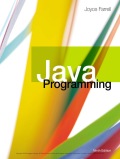
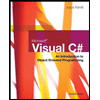
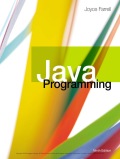
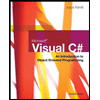