What are control structures?
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Control structures are the building blocks of the program. Control structures helps in writing complex logic and enables writing code for solving real world problems.
Purpose of control structures
- Control structure changes the flow of execution in a program.
- Allows the code to select an alternative path for execution.
- Allows repetition of certain sections of code.
- Complex logic can be carried out using a simple block.
Basic control structures
Any program code must contain any or all of the following control structures.
- Sequence.
- Conditional (Selection).
- Loops (Iteration).
Sequence
The default control structure is the sequence, where the instruction will be executed one after another. The program is executed from first line till the last line one after the other. There is no change is flow of control in this case.
Example
Code
include <stdio.h>
int main()
{
int num1 = 5, num2; //declaration and initialization of variable
num2 = num1 * num1; //calculate square
printf("n The square of 5 is:");
printf("%d",num2); //display statement
return 0;
}
Output
The square of 5 is 25.
Explanation
In the above program, the instruction gets executed one after the other in linear order. First, the variables num1 and num2 are declared. The variable num1 is initialized to 5. The square of num1 is calculated and the result is stored in num2. The printf statement displays the value of num2.
Conditionals
Conditionals help in changing the flow of execution based on the outcome of the condition. The conditional statement consists of relational operators/logical operators and it returns either true or false. If the outcome is true, one set of statements will be executed. If it is false, another set of statements get executed. It can be implemented using if statement, if-else statement, if-else if-else statement or switch-case statement.
If statement
Condition in the if-clause is executed. If it evaluates to true, then the statements in the if-block are executed. Otherwise, they are skipped.
Syntax
If(condition)
{
//statement
}
Flow chart
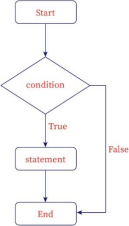
Example
Code
/* Check whether the value of y is positive or not. */
#include<stdio.h>
int main()
{
int y=2; //variable initialization
if(y >= 0) //if condition
{
printf("n y is a positive number "); //display statement
}
return 0;
}
Output
y is a positive number.
Explanation
First, the variable y is initialized to 2. If the value of the variable y is greater than or equal to 0, then it displays the message that y is a positive number.
If-else statement
It provides a dual alternative execution path. If the condition is true, then the statements in the IF block gets executed. Otherwise, statements in the ELSE block gets executed.
Syntax
If (condition)
{
//Statement
}
else
{
//Statement
}
Flow chart
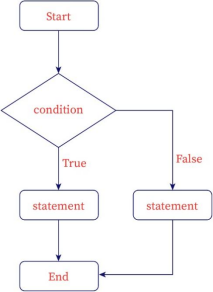
Example
Code
/* Check whether the value of y is positive or not. */
#include<stdio.h>
int main()
{
int y=-2; //variable initialization
if ( y >= 0 ) // if condition
{
printf("y is a positive number"); //display statement
}
else
{
printf("y is a negative number"); //display statement
}
return 0;
}
Output
"y is a negative number".
Explanation
In the given example, the variable y is initialized to -2. The value of y is checked whether it is greater than 0. If it is true then the message "y is a positive number" is displayed. Otherwise, the message "y is a negative number" is displayed.
Loops
It involves repeated execution of a set of statements as long as the specified condition is true.
Types of loops
- For loop: It is a counter-controlled loop which involves execution of set of statements a predefined number of times.
- While loop: It is an entry-controlled loop where the condition is evaluated before entering the loop body. The loop body is executed only when the condition evaluates to true.
- Do-while loop: It is an exit-controlled loop where the body of the loop is executed at least once. The condition is checked after the execution of body once. If the condition evaluates to true, the body of the loop is executed again. Otherwise, the loop is terminated.
For loop
The loop header consists of three parts separated by semi-colons. They are initialization, condition and increment or decrement operation.
Syntax
for (initial statement; test expression; iteration statement)
{
//statement
}
Flow chart
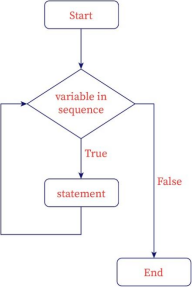
Example
Code
/* Printing numbers from 1 to 7 with for loop*/
#include<stdio.h>
int main() {
int i; //initialization
for(i=1;i<8;++i) //loop
{
printf("%d t" ,I); //display statement
}
return 0;
}
Output
1 2 3 4 5 6 7
Explanation
The for loop iterates from i=1 to 7 and displays the value of i.
While loop
In this loop, condition is evaluated first. If the condition evaluates to true, the loop body is executed. Otherwise, it is skipped.
Syntax
While (condition)
{
//statement
}
Flow chart
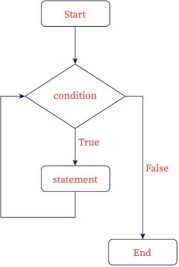
Example
Code
/* Printing numbers from 1 to 7 with while loop*/
#include <stdio.h>
int main() {
int i=1; //initialization
While(i<=7) { //loop
printf(“%dt”,I); //display statement
++i; //update
}
return 0;
}
Output
1 2 3 4 5 6 7
Explanation
The variable i is initialized to 1. The loop body is repeated until i is less than or equal to 7. The value of i is displayed and then it is incremented inside the loop body.
Do-while loop
Body of the loop is executed first and then the condition is evaluated. If the condition is true, the loop body is executed again. Otherwise, the loop is terminated.
Syntax
do{
//statement
} while (condition);
Flow chart
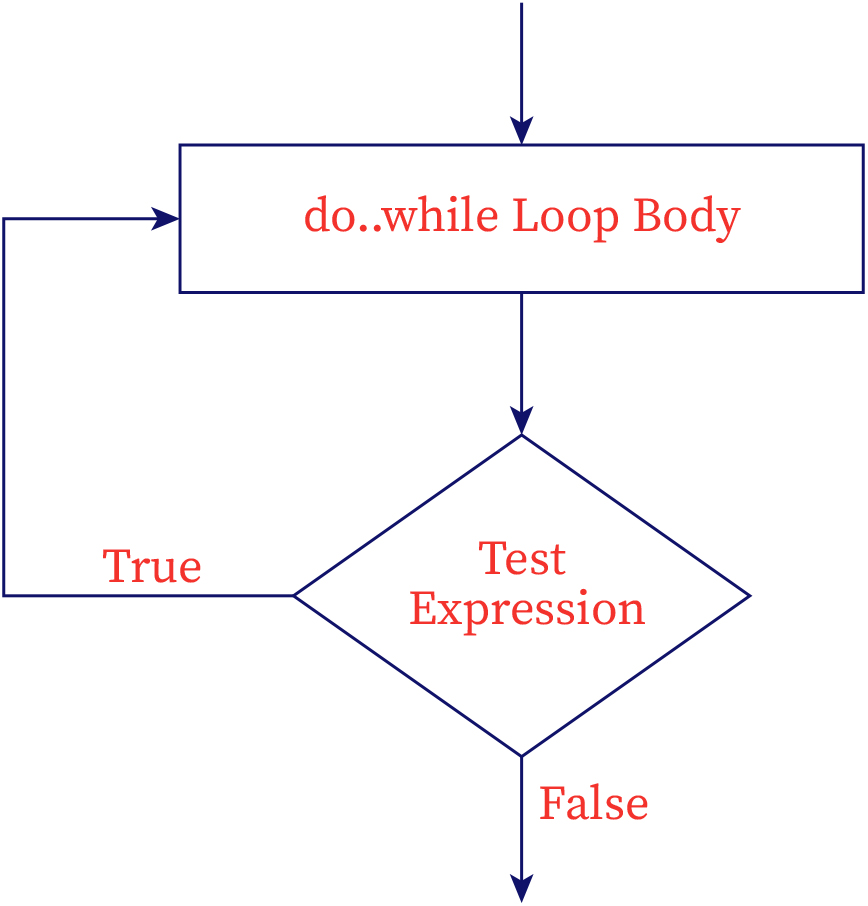
Example
/* Printing numbers from 1 to 7 with do-while loop */
#include <stdio.h>
int main()
{
int i = 1; //initialization
do{ //loop
printf("%d t", I); //display statement
++i; // update
}while(i<=7); //test expression
return 0;
}
Output
1 2 3 4 5 6 7
Explanation
The value of i is initialized to 1. It is displayed, incremented and then the condition is evaluated. If the condition is true, the loop body is repeated. In this case, it remains true as long as i is less or equal to 7.
Context and Applications
This topic is important for postgraduate and undergraduate courses, particularly for, Bachelor's in Computer Science Engineering, and Associate of Science in Computer Science.
Practice Problems
Question 1 : ________ statement is used to exit the loop.
- Break condition
- If condition
- Else condition
- Return condition
Answer: Option A is correct.
Explanation: Break statement is used to terminate the loop execution.
Question 2: Which of the following is not a type of control structure?
- Loop
- Sequence
- Selection
- Function
Answer: Option D is correct.
Explanation: Control structures are classified into three types namely sequence, conditional and loops.
Question 3: ____ is the default control structure.
- Selection
- Loop
- Sequence
- None of the above
Answer: Option C is correct.
Explanation: The default control structure is the sequence, where the instruction will get executed one after another. It is also called an overriding structure. These are defined by the programming languages themselves.
Question 4: ___ is an exit-controlled loop.
- Do-while loop
- For loop
- While loop
- All the above
Answer: Option A is correct.
Explanation: Do-while loop an exit-controlled loop in which the condition is checked only after execution of body once.
Question 5: Which statement corresponds to dual alternative decision structure?
- For statement
- While statement
- If-else statement
- If statement
Answer: Option C is correct.
Explanation: If-else statement allows the program to select an alternative route. It is used to take either of the two different paths of execution depending upon the outcome of the condition.
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Control structures Homework Questions from Fellow Students
Browse our recently answered Control structures homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.