What is a Switch Statement?
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
Switch statements function similarly to the if statement in programming languages such as C/C++, C#, Visual Basic.NET, and Java, and are present in most high-level imperative programming languages such as Pascal, Ada, C/C++, C#, Visual Basic.NET, and Java.
What are the Conditional Statements?
Conditional statements allow you to make a decision based on a set of circumstances. These conditions are specified by a set of conditional statements that contain boolean expressions that are evaluated to a true or false boolean value. In programming languages such as C and C++, there are several types of conditional statements.
- If statement
- If-Else statement
- Nested If-else statement
- If-Else If ladder
- Switch statement
Switch Statement
A switch statement checks a variable for equality against a set of values. Each value is referred to as a case, and the variable being toggled is checked for each switch case. A switch statement is a selection statement that allows programme control to be transferred to a statement list with a switch label that corresponds to the value of the switch expression.
The Switch Statement is Subject to the Following Rules.
- The expression in a switch statement must be either an integral or boolean expression, or it must be of a class type with a single conversion function to an integral or boolean value. If no expression is supplied, the default value is true.
- A switch can contain an unlimited number of case statements. Each case is followed by a colon and the value to be compared to.
- A case's constant-expression must be of the same data type as the variable in the switch, and it must be either a constant or a literal.
- When the variable being turned on equals a case, the statements that follow that case will be executed. There is no need for a break in the case statement.
- A switch statement may include a default case, which must appear at the end of the switch. When none of the other cases are true, the default case can be used to complete a task. In the default case, no break is required.
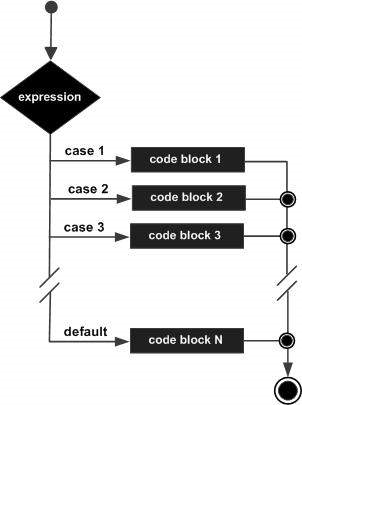
The JavaScript Switch Statement
Syntax:
switch(expression) {
case x:
// code block
break;
case y:
// code block
break;
default:
// code block
}
Example using a Switch Statement
The getDay() method returns the weekday as a number between 0 and 6.
(Sunday=0, Monday=1, Tuesday=2 ..)
This example uses the weekday number to calculate the weekday name:
switch (new Date().getDay()) {
case 0:
day = "Sunday";
break;
case 1:
day = "Monday";
break;
case 2:
day = "Tuesday";
break;
case 3:
day = "Wednesday";
break;
case 4:
day = "Thursday";
break;
case 5:
day = "Friday";
break;
case 6:
day = "Saturday";
}
The result of day will be:Thursday
Case
The switch statement in programing languages like java compares the value of a variable to multiple cases. When a case match is found, a set of statements associated with that case is executed. Each case in a switch block has a unique name/number, which is referred to as an identifier.
The word case is followed by an integral constant expression and a colon in a case label. Each integral constant expression's value must represent a unique value; duplicate case labels are not permitted. Multiple case labels can be placed anywhere a single case label can be placed.
The Break Keyword
A break statement is optional in each case statement. When control approaches the break statement, it jumps to the control expression following the switch expression. If no break statement is found, the next case is executed. A default label for the case value is optional.
The Default Keyword
The default keyword specifies the code that will be executed if no case match is found:In a switch statement, the default keyword refers to the default block of code. If there is no case match in the switch, the default keyword specifies some code to run. It is important to note that if the default keyword is used as the last statement in a switch block, no break is required.
Data Types & Switch
A switch can work with the primitive data types byte, short, char, and int. It also works with enumerated types (discussed in Enum Types), the String class, and a few special classes that wrap specific primitive types: Character, Byte, Short, and Integer (discussed in Numbers and Strings).
Fallthrough
In practise, fallthrough is usually prevented by inserting a break keyword at the end of the matching body, which exits the switch block's execution, but this can cause bugs due to unintentional fallthrough if the programmer forgets to insert the break statement.
Common Mistakes
- Use of repetative code while writing a computer program.
- Variable naming is essential in programing sometimes it is happened that general trams are used in variable declaration.
- Syntax error will not allow you to run the progam.
- Use of same constant in different cases.
- Use of relational expression & variable expression in case.
Context & Applications
This topic is significant in the professional exams for both undergraduate and graduate courses, especially for
- Bachelors in Technology (Computer Science)
- Masters in Technology (Computer Science)
- Bachelors in Science (Computer Science)
- Masters in Science (Computer Science)
Related Concepts
- Exception handling
- Boolean Expression
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Switch Statement Homework Questions from Fellow Students
Browse our recently answered Switch Statement homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.