Hello, can you please help me with this Java homework? Here are the indications and I also attached two pictures with the notes (slides13-17 are in those two pictures) Create a Java program for the definition for the class Student Slides 13 - 16 are for class definition Create a Java application program to use this class Slide 17 is for the application Name your application program as useStudent_YourInitial.java Submit the bot
Hello, can you please help me with this Java homework? Here are the indications and I also attached two pictures with the notes (slides13-17 are in those two pictures) Create a Java program for the definition for the class Student Slides 13 - 16 are for class definition Create a Java application program to use this class Slide 17 is for the application Name your application program as useStudent_YourInitial.java Submit the bot
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 6PE
Related questions
Question
100%
Hello, can you please help me with this Java homework? Here are the indications and I also attached two pictures with the notes (slides13-17 are in those two pictures)
- Create a Java
program for the definition for the class Student- Slides 13 - 16 are for class definition
- Create a Java application program to use this class
- Slide 17 is for the application
- Name your application program as useStudent_YourInitial.java
- Submit the both programs (the class definition & the application) and the screenshot of your compiler window.
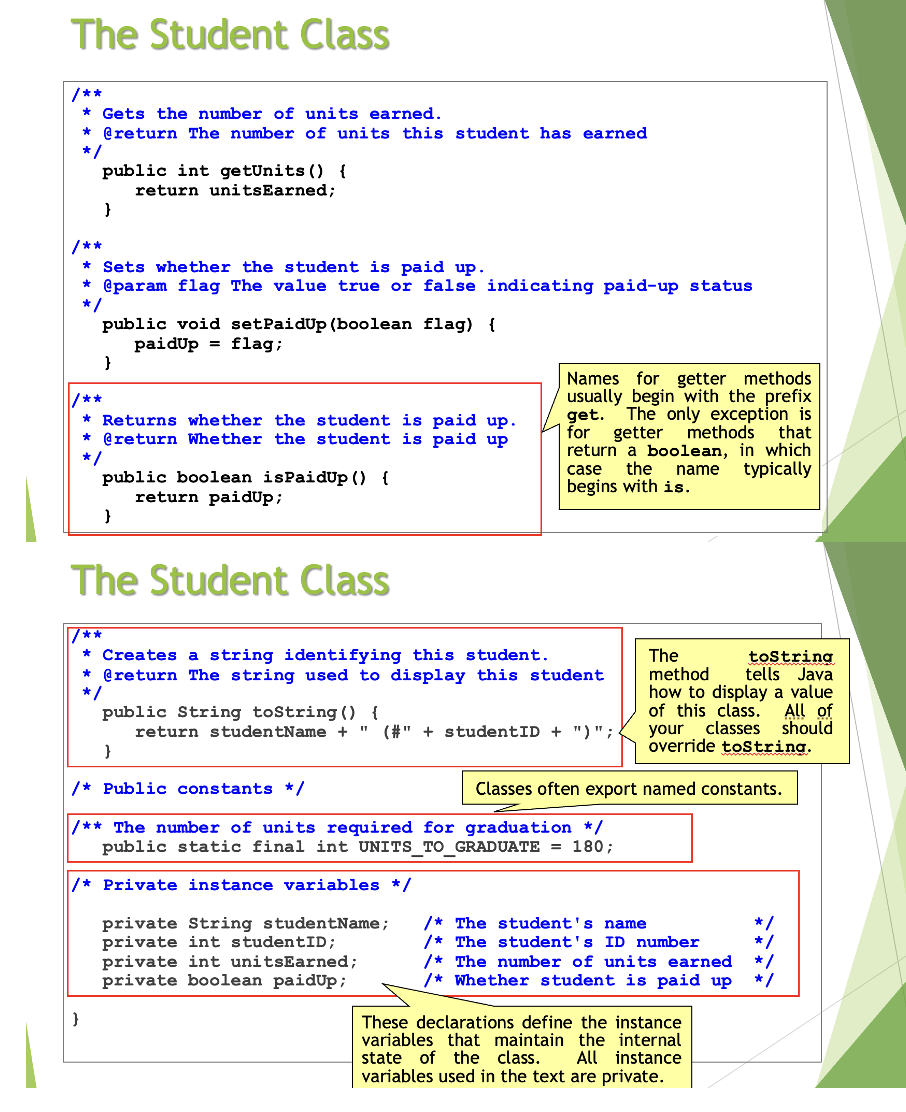
Transcribed Image Text:The Student Class
/**
Gets the number of units earned.
* @return The number of units this student has earned
*/
public int getUnits () {
return unitsEarned;
/**
* Sets whether the student is paid up.
/**
}
* @param flag The value true or false indicating paid-up status
*/
}
public void setPaidUp (boolean flag) {
paidUp = flag;
}
*Returns whether the student is paid up.
* @return Whether the student is paid up
*/
public boolean isPaidUp () {
return paidUp;
}
The Student Class
/**
* Creates a string identifying this student.
* @return The string used to display this student
Names for getter methods
usually begin with the prefix
get. The only exception is
for getter methods that
return a boolean, in which
case the name typically
begins with is.
public String toString() {
return studentName + 17 (#" + studentID + ")";
}
/* Public constants */
/** The number of units required for graduation */
public static final int UNITS_TO_GRADUATE = 180;
/* Private instance variables */
private String studentName; /* The student's name
private int studentID;
private int unitsEarned;
private boolean paidUp;
The
toString
method tells Java
how to display a value
of this class. All of
your classes should
override toString.
Classes often export named constants.
/* The student's ID number
/* The number of units earned
/* Whether student is paid up
These declarations define the instance
variables that maintain the internal
state of the class. All instance
variables used in the text are private.
*/
*/
*/
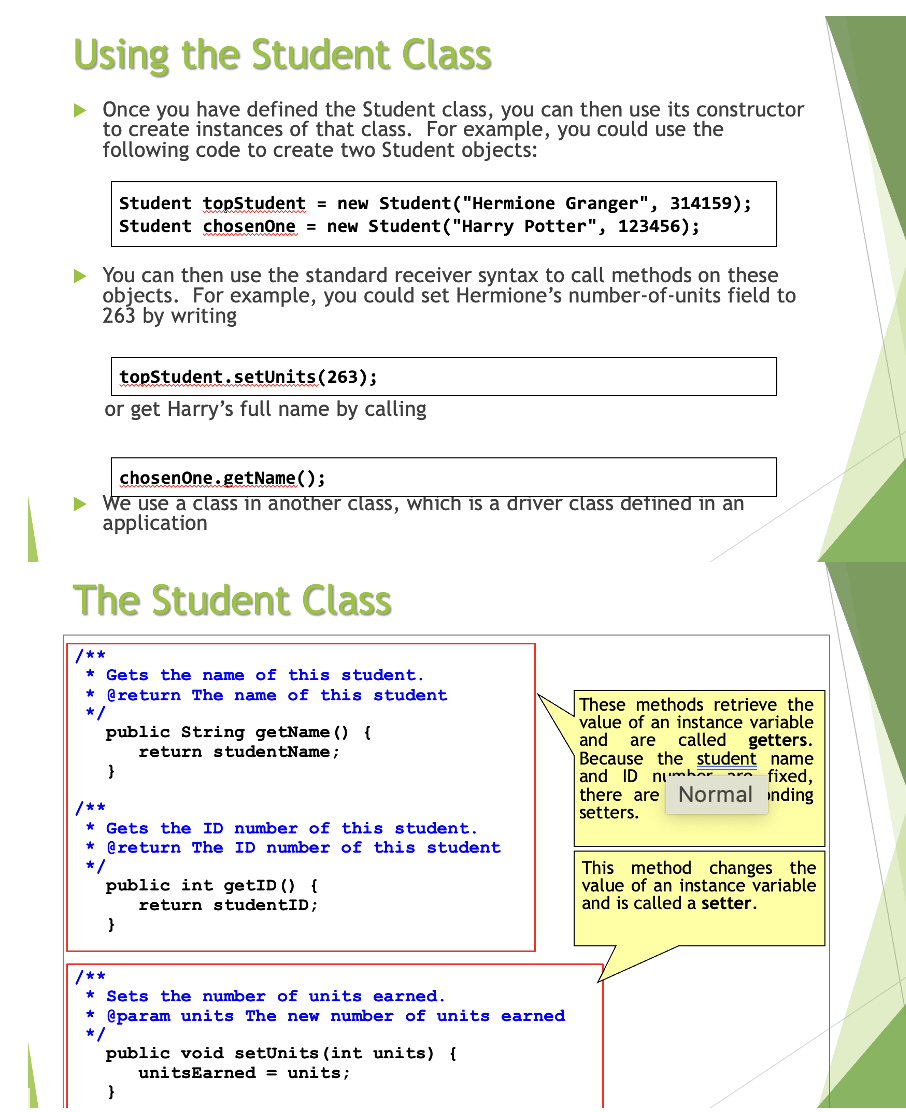
Transcribed Image Text:Using the Student Class
▸ Once you have defined the Student class, you can then use its constructor
to create instances of that class. For example, you could use the
following code to create two Student objects:
► You can then use the standard receiver syntax to call methods on these
objects. For example, you could set Hermione's number-of-units field to
263 by writing
topStudent.setUnits (263);
or get Harry's full name by calling
Student topStudent = new Student ("Hermione Granger", 314159);
Student chosenOne = new Student ("Harry Potter", 123456);
chosenOne.getName();
►We use a class in another class, which is a driver class defined in an
application
The Student Class
/**
* Gets the name of this student.
* @return The name of this student
public String getName() {
return studentName;
}
/**
* Gets the ID number of this student.
* @return The ID number of this student
*/
public int getID () {
return studentID;
}
/**
* Sets the number of units earned.
* @param units The new number of units earned
public void setUnits (int units) {
unitsEarned = units;
}
These methods retrieve the
value of an instance variable
and
are called getters.
Because the student name
and ID number fixed,
there are Normal nding
setters.
AVA
This method changes the
value of an instance variable
and is called a setter.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
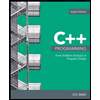
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
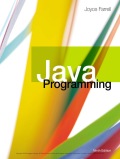
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
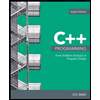
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
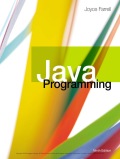
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT