Quadratic equations The form of a quadratic equation is ax2 + bx + c = 0. Write a program that solves a quadratic equation in all cases, including when both roots are complex numbers. Remember that the solutions are x = (-b + sqrt(b2 -4ac))/2a and (-b - sqrt(b2 -4ac))/2a For this you will need to set up the following classes: Complex: Encapsulates a complex number. ComplexPair: Encapsulates a pair of complex numbers. Quadratic: Encapsulates a quadratic equation. – Assume the coefficients are all of type int for this example. SolveEquation: Contains the main method. (I give this to you it is shown below) Along with the usual constructors, accessors and mutators you will need the following additional methods: In the Complex class a method called isReal to determine whether a complex object is real or not returning a boolean value. In the ComplexPair class a method bothIdentical that determines if both complex numbers are identical, returning a boolean value. In the Quadratic class a method that solves the quadratic equation and returns a ComplexPair object. Please use the solve SolveEquation class found below. The three parameters in each case are for the a, b and c coefficients respectively. SolveEquation includes the main program. It includes all the testing you need. Output should make comments as to which type of roots we get (double, real real root, distinct roots, complex roots). All coefficients in output should be to 2 decimal places. See below for the sample output.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
- Quadratic equations
The form of a quadratic equation is ax2 + bx + c = 0.
Write a program that solves a quadratic equation in all cases, including when both roots are complex numbers. Remember that the solutions are
x = (-b + sqrt(b2 -4ac))/2a and (-b - sqrt(b2 -4ac))/2a
For this you will need to set up the following classes:
Complex: Encapsulates a complex number.
ComplexPair: Encapsulates a pair of complex numbers.
Quadratic: Encapsulates a quadratic equation. – Assume the coefficients are all of type int for this example.
SolveEquation: Contains the main method. (I give this to you it is shown below)
Along with the usual constructors, accessors and mutators you will need the following additional methods:
In the Complex class a method called isReal to determine whether a complex object is real or not returning a boolean value.
In the ComplexPair class a method bothIdentical that determines if both complex numbers are identical, returning a boolean value.
In the Quadratic class a method that solves the quadratic equation and returns a ComplexPair object.
Please use the solve SolveEquation class found below. The three parameters in each case are for the a, b and c coefficients respectively. SolveEquation includes the main program. It includes all the testing you need.
Output should make comments as to which type of roots we get (double, real real root, distinct roots, complex roots). All coefficients in output should be to 2 decimal places. See below for the sample output.
SolveEquation class:
public class SolveEquation
{
public static void main( String [] args )
{
Quadratic q1 = new Quadratic( 0, -2, 6 );
System.out.printf( "Quadratic equation #1: %s\n", q1 );
ComplexPair c1 = q1.solveQuadratic( );
System.out.printf( "%s\n", q1.getComment( ) );
System.out.printf( "Solutions: %s\n\n", c1 );
Quadratic q2 = new Quadratic( -2, 4, -2 );
System.out.printf( "Quadratic equation #2: %s\n", q2 );
ComplexPair c2 = q2.solveQuadratic( );
System.out.printf( "%s\n", q2.getComment( ) );
System.out.printf( "Solutions: %s\n\n", c2);
Quadratic q3 = new Quadratic( 1, 4, 3 );
System.out.printf( "Quadratic equation #3: %s\n", q3);
ComplexPair c3 = q3.solveQuadratic( );
System.out.printf( "%s\n", q3.getComment( ) );
System.out.printf( "Solutions: %s\n\n", c3);
Quadratic q4 = new Quadratic( -1, 2, -5 );
System.out.printf( "Quadratic equation #4: %s\n", q4);
ComplexPair c4 = q4.solveQuadratic( );
System.out.printf( "%s\n", q4.getComment( ) );
System.out.printf( "Solutions: %s\n\n", c4);
}
}
Sample Output:
Quadratic equation #1: - 2x + 6 = 0
Linear equation: one real root
Solutions: first: 3.00; second: 3.00
Quadratic equation #2: -2x^2 + 4x - 2 = 0
Double real root
Solutions: first: 1.00; second: 1.00
Quadratic equation #3: x^2 + 4x + 3 = 0
Two distinct real roots
Solutions: first: -1.00; second: -3.00
Quadratic equation #4: -x^2 + 2x - 5 = 0
Two distinct complex roots
Solutions: first: 1.00 - 2.00i; second: 1.00 + 2.00i

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

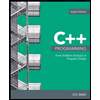
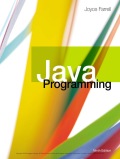
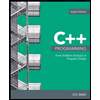
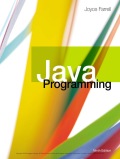