Write a checkbook balancing program in C++. The program will read in the following information for all checks that were not cashed as of the last time the user balanced their checkbook: the number of each check the amount of the check whether or not the check has yet been cashed Use a dynamic array with a class base type for the checks. The check class should have three member variables: check number check amount whether or not the check's been cashed And don't forget to provide accessors, mutators, and constructors as well as input and output methods. BTW, the amount member variable will be of type Money. Just save this link to the Money class definition — you do not have to design it yourself! (A closer look, however, reveals that it is just a class definition... The definitions for the methods seem to be missing. Bummer...) In addition to all of the checks since the last checkbook update, your program should also read all of the user's deposits, the last balance we reported to them, and the new balance the bank reported to them. You will most likely desire a second [dynamic] array to hold all of the deposits. (A deposit is, of course, a Money type value.) Your program should calculate and print the total for all checks, the total for all deposits, the calculated new balance, and how much this differs from the reported new balance. (Just in case: the new account balance should be the old balance plus all deposits and minus all cashed checks.) Then display two lists of checks: those already cashed and those still not cashed. Sort each list from lowest to highest check number. (Note that this will not require you to split the checks into two arrays...) To make the dynamic array management easier, you can ask the user how many checks and deposits they have to enter. The picture is the .h file for money
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Write a checkbook balancing
-
the number of each check
-
the amount of the check
-
whether or not the check has yet been cashed
Use a dynamic array with a class base type for the checks.
The check class should have three member variables:
-
check number
-
check amount
-
whether or not the check's been cashed
And don't forget to provide accessors, mutators, and constructors as well as input and output methods.
BTW, the amount member variable will be of type Money. Just save this link to the Money class definition — you do not have to design it yourself!
(A closer look, however, reveals that it is just a class definition... The definitions for the methods seem to be missing. Bummer...)
In addition to all of the checks since the last checkbook update, your program should also read all of the user's deposits, the last balance we reported to them, and the new balance the bank reported to them. You will most likely desire a second [dynamic] array to hold all of the deposits. (A deposit is, of course, a Money type value.)
Your program should calculate and print the total for all checks, the total for all deposits, the calculated new balance, and how much this differs from the reported new balance. (Just in case: the new account balance should be the old balance plus all deposits and minus all cashed checks.)
Then display two lists of checks: those already cashed and those still not cashed. Sort each list from lowest to highest check number. (Note that this will not require you to split the checks into two arrays...)
To make the dynamic array management easier, you can ask the user how many checks and deposits they have to enter.
The picture is the .h file for money.
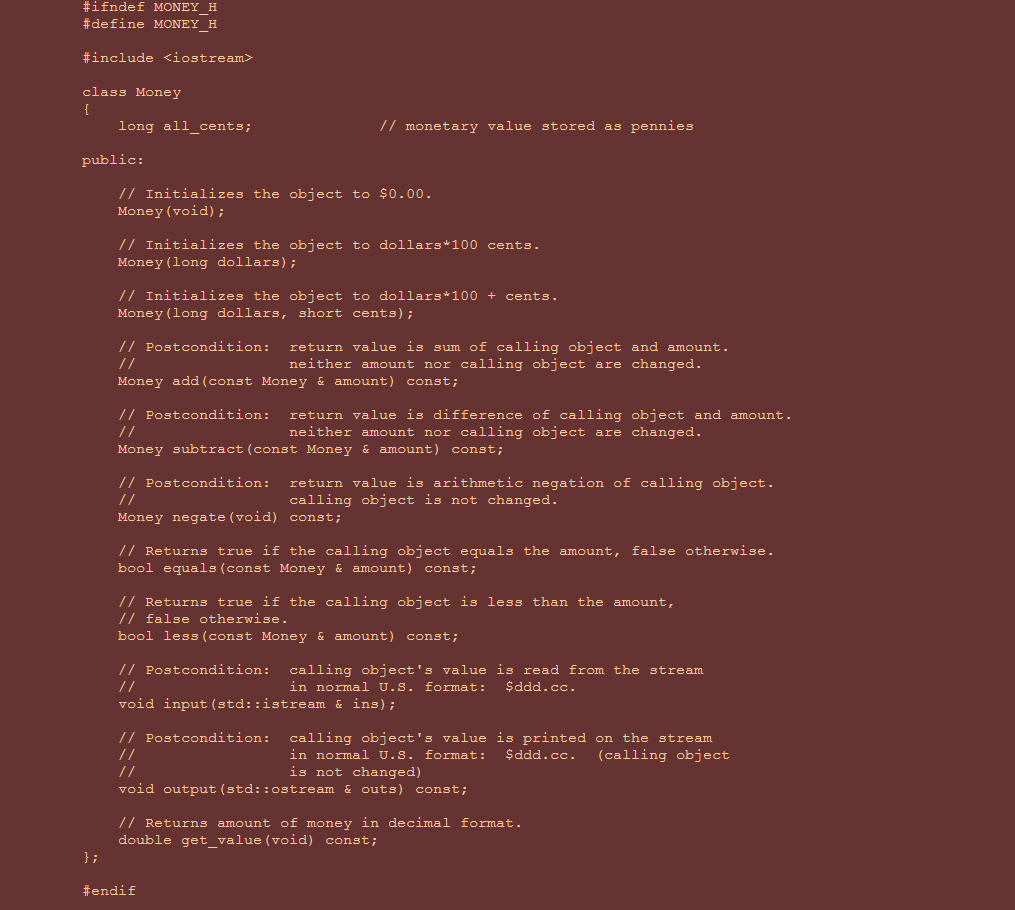

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

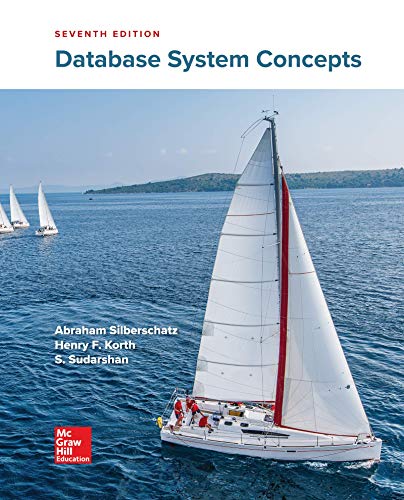
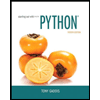
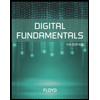
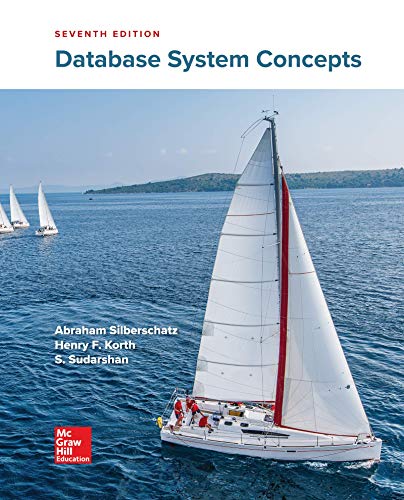
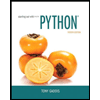
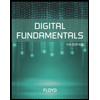
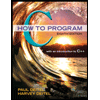
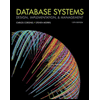
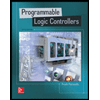