Here's the C++ Programming Exercise: The population of town A is less than the population of town B. However, the population of town A is growing faster than the population of town B.Write a program that prompts the user to enter: 1. The population of town A 2. The population of town B 3. The growth rate of town A4. The growth rate of town B The program outputs: 1. After how many years the population of town A will be greater than or equal to the population of town B 2. The populations of both the towns at that time.(A sample input is: Population of town A = 5,000, growth rate of town A = 4%, population of town B = 8,000, and growth rate of town B = 2%.) Now here's my solution: #include <iostream>using namespace std;int main(){//declare variablesint pop_A;int pop_B;int year = 1;double growth_rate_A;double growth_rate_B;//Prompt the user to input the population and growth rate of Town A.cout <<"Enter the population and growth rate of Town A: ";cin >> pop_A >> growth_rate_A;cout << endl;//Prompt the user to input the population and growth rate of Town B.cout <<"Enter the population and growth rate of Town B: ";cin >> pop_B >> growth_rate_B;cout << endl;if (pop_A < pop_B && growth_rate_A > growth_rate_B){do {(pop_A = ((growth_rate_A /100) * pop_A) + pop_A); //calculates the population growth in one year.(pop_B = ((growth_rate_B / 100) * pop_B) + pop_B); year++;} while (pop_A < pop_B);int pop_Diff = pop_A - pop_B;int pop_Total = pop_A + pop_B;cout <<"Town A will surpass Town B in population after " << year << " years." << endl;cout << "The final population of Town A is: " << pop_A << endl;cout << "The final population of Town B is: " << pop_B << endl;cout << "In year " << year << ", Town A has " << pop_Diff << " more people than Town B." << endl;cout << "The total population of both Town A and Town B is " << pop_Total << endl;} else{cout << "The Values Doesn't Compute.";}return 0;} My class is conducted in Cengage. As a result, the test case's numbers are rather odd, and it's causing Cengage to reject my lab. The numbers used for test case #1 are: 44, 240, 44 and 30. The numbers for test case #2 are: 50000, 75000, 25 and 12. Both outputs are "The Values Doesn't Compute." Can someone help me to find out how to accomidate these crazy Cengage test values?
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
Here's the C++
The population of town A
is less than the population of town B.
However, the population of town A
is growing faster than the
population of town B.
Write a program that prompts the
user to enter:
1. The population of town A
2. The population of town B
3. The growth rate of town A
4. The growth rate of town B
The program outputs:
1. After how many years the
population of town A will be
greater than or equal to the
population of town B
2. The populations of both the
towns at that time.
(A sample input is: Population of
town A = 5,000, growth rate of town
A = 4%, population of town B =
8,000, and growth rate of town B =
2%.)
Now here's my solution:
#include <iostream>
using namespace std;
int main()
{
//declare variables
int pop_A;
int pop_B;
int year = 1;
double growth_rate_A;
double growth_rate_B;
//Prompt the user to input the population and growth rate of Town A.
cout <<"Enter the population and growth rate of Town A: ";
cin >> pop_A >> growth_rate_A;
cout << endl;
//Prompt the user to input the population and growth rate of Town B.
cout <<"Enter the population and growth rate of Town B: ";
cin >> pop_B >> growth_rate_B;
cout << endl;
if (pop_A < pop_B && growth_rate_A > growth_rate_B)
{
do {
(pop_A = ((growth_rate_A /100) * pop_A) + pop_A); //calculates the population growth in one year.
(pop_B = ((growth_rate_B / 100) * pop_B) + pop_B); year++;
} while (pop_A < pop_B);
int pop_Diff = pop_A - pop_B;
int pop_Total = pop_A + pop_B;
cout <<"Town A will surpass Town B in population after " << year << " years." << endl;
cout << "The final population of Town A is: " << pop_A << endl;
cout << "The final population of Town B is: " << pop_B << endl;
cout << "In year " << year << ", Town A has " << pop_Diff << " more people than Town B." << endl;
cout << "The total population of both Town A and Town B is " << pop_Total << endl;
} else
{
cout << "The Values Doesn't Compute.";
}
return 0;
}
My class is conducted in Cengage. As a result, the test case's numbers are rather odd, and it's causing Cengage to reject my lab. The numbers used for test case #1 are: 44, 240, 44 and 30. The numbers for test case #2 are: 50000, 75000, 25 and 12. Both outputs are "The Values Doesn't Compute." Can someone help me to find out how to accomidate these crazy Cengage test values?

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

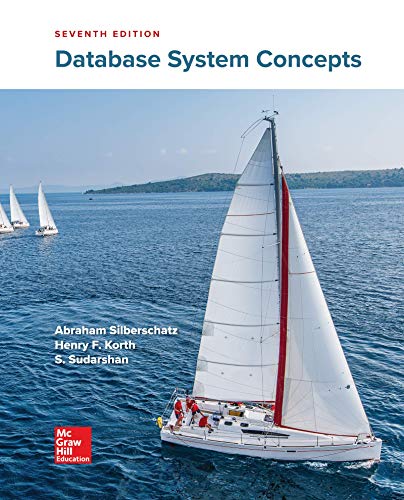
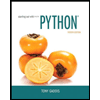
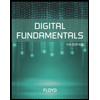
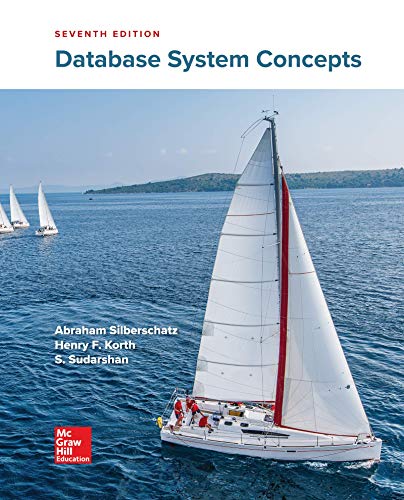
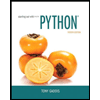
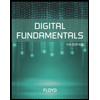
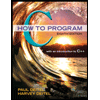
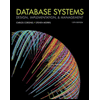
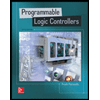