Hi can please help with the union bagsUnion methode i am trying to group duplicate values together. i know i need to use the itemOccurences and contains method to duplicate the two bags in one , but i dont know how to do that in c++. here is the program detail. I just need the union bag method to get fixed. this is the deatail Hint: Consider using class method itemOccurrences in your solution for method bagsUnion. Union Definition The union of two Bag objects, bag1 and bag2, is another, new Bag object that contains of all items of the two source objects. The new Bag object may contain more than one copy of an item if, 1) both objects contain the item once, and 2) if either object contains multiple copies of an item. For example, if bag1 contains 5 occurrences of item 2 and bag2 contains 3 copies of 2, the new Bag object will contain 8 occurrences of 2. and here is my code #include #include #include #include "Bag.h" template Bag::Bag() { arrayNumbers = 0; } template Bag::Bag(const Type& item) { arrayNumbers = 0; items[arrayNumbers++] = item; } template Bag::Bag(const Bag& bag) { arrayNumbers = bag.arrayNumbers; for(int value = 0;value < arrayNumbers; ++value){ items[value] = bag.items[value]; } } template Bag::~Bag() { arrayNumbers= 0; } template const Bag& Bag::operator=(const Bag& bag) { if (this != &bag) { arrayNumbers = bag.arrayNumbers; for (int i = 0; i < arrayNumbers; ++i) { items[i] = bag.items[i]; } } return *this; } template bool Bag::addItem(const Type& item) { if (isFull()) { return false; } items[arrayNumbers++] = item; return true; } template bool Bag::removeItem(const Type& item) { int index = -1; int value = 0; while(value < arrayNumbers) { if (items[value] == item) { index = value; break; } ++value; } if (index == -1) return false; --arrayNumbers; int number = index; while( number < arrayNumbers) { items[value] = items[number + 1]; ++number; } return true; } template int Bag::removeAllItems(const Type& item) { int cnt = 0; while (removeItem(item)) ++cnt; return cnt; } template void Bag:: empty(){ arrayNumbers = 0; } template int Bag::nmbrItems() { return arrayNumbers; } template int Bag::capacity() { return BAG_SIZE; } template bool Bag::isEmpty() { return arrayNumbers == 0; } template bool Bag::isFull() { return arrayNumbers == BAG_SIZE; } template bool Bag::contains(const Type& item) { int value = 0; while( value < arrayNumbers) { if (items[value] == item) { return true; } ++value; } return false; } template int Bag::itemOccurrences(const Type& item) { int count = 0; int value = 0; while( value < arrayNumbers) { if (items[value] == item) { ++count; } ++value; } return count; } template Bag Bag::bagsUnion(const Bag& bag ) { Bag unionBag; unionBag.arrayNumbers = bag.arrayNumbers; for (int i = 0; i < unionBag.arrayNumbers; ++i) { unionBag.items[i] = bag.items[i]; unionBag.contains(bag.items[i]); } return unionBag; } template string Bag::toString() { if (isEmpty()) return "[]"; string number = "[" + std::to_string(items[0]); int value = 1; while( value < arrayNumbers) { number += ", " + std::to_string(items[value]); ++value; } number += "]"; return number; }
Hi can please help with the union
bagsUnion methode i am trying to group duplicate values together. i know i need to use the itemOccurences and contains method to duplicate the two bags in one , but i dont know how to do that in c++.
here is the program detail. I just need the union bag method to get fixed.
this is the deatail
-
Hint:
-
Consider using class method itemOccurrences in your solution for method bagsUnion. Union Definition
The union of two Bag objects, bag1 and bag2, is another, new Bag object that contains of all items of the two source objects. The new Bag object may contain more than one copy of an item if, 1) both objects contain the item once, and 2) if either object contains multiple copies of an item. For example, if bag1 contains 5 occurrences of item 2 and bag2 contains 3 copies of 2, the new Bag object will contain 8 occurrences of 2.
and here is my code#include <map>
#include <vector>
#include <string>
#include "Bag.h"
template<typename Type>
Bag<Type>::Bag() {
arrayNumbers = 0;
}
template<typename Type>
Bag<Type>::Bag(const Type& item) {
arrayNumbers = 0;
items[arrayNumbers++] = item;
}
template<typename Type>
Bag<Type>::Bag(const Bag& bag) {
arrayNumbers = bag.arrayNumbers;
for(int value = 0;value < arrayNumbers; ++value){
items[value] = bag.items[value];
}
}
template<typename Type>
Bag<Type>::~Bag() {
arrayNumbers= 0;
}
template<typename Type>
const Bag<Type>& Bag<Type>::operator=(const Bag<Type>& bag) {
if (this != &bag)
{
arrayNumbers = bag.arrayNumbers;
for (int i = 0; i < arrayNumbers; ++i) {
items[i] = bag.items[i];
}
}
return *this;
}
template<typename Type>
bool Bag<Type>::addItem(const Type& item) {
if (isFull()) {
return false;
}
items[arrayNumbers++] = item;
return true;
}
template<typename Type>
bool Bag<Type>::removeItem(const Type& item) {
int index = -1;
int value = 0;
while(value < arrayNumbers) {
if (items[value] == item) {
index = value;
break;
}
++value;
}
if (index == -1) return false;
--arrayNumbers;
int number = index;
while( number < arrayNumbers) {
items[value] = items[number + 1];
++number;
}
return true;
}
template<typename Type>
int Bag<Type>::removeAllItems(const Type& item) {
int cnt = 0;
while (removeItem(item)) ++cnt;
return cnt;
}
template<typename Type>
void Bag<Type>:: empty(){
arrayNumbers = 0;
}
template<typename Type>
int Bag<Type>::nmbrItems() {
return arrayNumbers;
}
template<typename Type>
int Bag<Type>::capacity() {
return BAG_SIZE;
}
template<typename Type>
bool Bag<Type>::isEmpty() {
return arrayNumbers == 0;
}
template<typename Type>
bool Bag<Type>::isFull() {
return arrayNumbers == BAG_SIZE;
}
template<typename Type>
bool Bag<Type>::contains(const Type& item) {
int value = 0;
while( value < arrayNumbers) {
if (items[value] == item) {
return true;
}
++value;
}
return false;
}
template<typename Type>
int Bag<Type>::itemOccurrences(const Type& item) {
int count = 0;
int value = 0;
while( value < arrayNumbers) {
if (items[value] == item) {
++count;
}
++value;
}
return count;
}
template<typename Type>
Bag<Type> Bag<Type>::bagsUnion(const Bag& bag ) {
Bag<Type> unionBag;
unionBag.arrayNumbers = bag.arrayNumbers;
for (int i = 0; i < unionBag.arrayNumbers; ++i) {
unionBag.items[i] = bag.items[i];
unionBag.contains(bag.items[i]);
}
return unionBag;
}
template<typename Type>
string Bag<Type>::toString() {
if (isEmpty()) return "[]";
string number = "[" + std::to_string(items[0]);
int value = 1;
while( value < arrayNumbers) {
number += ", " + std::to_string(items[value]);
++value;
}
number += "]";
return number;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

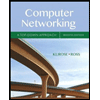
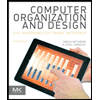
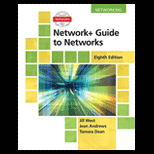
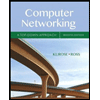
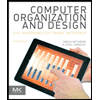
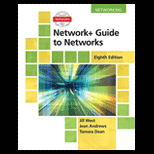
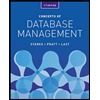
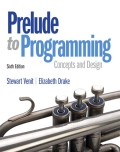
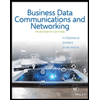