list, row_no: int, Please write a function named block_correct(sudoku: column_no: int), which takes a two-dimensional array representing a sudoku grid, and two integers referring to the row and column indexes of a single square, as its arguments. Rows and columns are indexed from 0. The function should return True or False depending on whether the 3 by 3 block to the right and down from the given indexes is filled in correctly. That is, whether the block contains each of the numbers 1 to 9 at most once. Notice that this function does not strictly follow the rules of sudoku. In a real game of sudoku there are only 9 blocks to check, and these are located at indexes (0, 0), (0, 3), (0, 6), (3, 0), (3, 3), (3, 6), (6, 0), (6, 3) and (6, 6). Such restrictions on indexes should not be implemented here. sudoku [9, 0, 0, 0, 8, 0, 3, 0, 0], [2, 0, 0, 2, 5, 0, 7, 0, 0], [0, 2, 0, 3, 0, 0, 0, 0, 4], [2, 9, 4, 0, 0, 0, 0, 0, 0], [0, 0, 0, 7, 3, 0, 5, 6, 0], [7, 8, 5, 8, 6, 0, 4, 0, 0], [0, 0, 7, 8, 0, 3, 9, 0, 0], [0, 0, 1, 0, 0, 0, 0, 0, 3], [3, 0, 0, 0, 0, 0, 0, 0, 2] ] = [ print (block_correct (sudoku, 0, 0)) print (block_correct (sudoku, 1, 2))
list, row_no: int, Please write a function named block_correct(sudoku: column_no: int), which takes a two-dimensional array representing a sudoku grid, and two integers referring to the row and column indexes of a single square, as its arguments. Rows and columns are indexed from 0. The function should return True or False depending on whether the 3 by 3 block to the right and down from the given indexes is filled in correctly. That is, whether the block contains each of the numbers 1 to 9 at most once. Notice that this function does not strictly follow the rules of sudoku. In a real game of sudoku there are only 9 blocks to check, and these are located at indexes (0, 0), (0, 3), (0, 6), (3, 0), (3, 3), (3, 6), (6, 0), (6, 3) and (6, 6). Such restrictions on indexes should not be implemented here. sudoku [9, 0, 0, 0, 8, 0, 3, 0, 0], [2, 0, 0, 2, 5, 0, 7, 0, 0], [0, 2, 0, 3, 0, 0, 0, 0, 4], [2, 9, 4, 0, 0, 0, 0, 0, 0], [0, 0, 0, 7, 3, 0, 5, 6, 0], [7, 8, 5, 8, 6, 0, 4, 0, 0], [0, 0, 7, 8, 0, 3, 9, 0, 0], [0, 0, 1, 0, 0, 0, 0, 0, 3], [3, 0, 0, 0, 0, 0, 0, 0, 2] ] = [ print (block_correct (sudoku, 0, 0)) print (block_correct (sudoku, 1, 2))
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.1: Function And Parameter Declarations
Problem 10E
Related questions
Question
Can you use Python
Thanks
![Please write a function named block_correct (sudoku: list, row_no: int,
column_no: int), which takes a two-dimensional array representing a sudoku grid, and
two integers referring to the row and column indexes of a single square, as its arguments.
Rows and columns are indexed from 0.
The function should return True or False depending on whether the 3 by 3 block to the
right and down from the given indexes is filled in correctly. That is, whether the block
contains each of the numbers 1 to 9 at most once.
Notice that this function does not strictly follow the rules of sudoku. In a real game of
sudoku there are only 9 blocks to check, and these are located at indexes (0, 0), (0, 3), (0,
6), (3, 0), (3, 3), (3, 6), (6, 0), (6, 3) and (6, 6). Such restrictions on indexes should not be
implemented here.
sudoku = [
[9, 0, 0, 0, 8, 0, 3, 0, 0],
[2, 0, 0, 2, 5, 0, 7, 0, 0],
[0, 2, 0, 3, 0, 0, 0, 0, 4],
[2, 9, 4, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 7, 3, 0, 5, 6, 0],
[7, 0, 5, 0, 6, 0, 4, 0, 0],
[0, 0, 7, 8, 0, 3, 9, 0, 0],
[0, 0, 1, 0, 0, 0, 0, 0, 3],
[3, 0, 0, 0, 0, 0, 0, 0, 21
]
print (block_correct (sudoku, 0, 0))
print (block_correct (sudoku, 1, 2))
False
True
The first function call should check the 3 by 3 block beginning with the square at indexes
0, 0:
900
200
020
Sample output
The second function call should check the 3 by 3 block beginning with the square at row 1,
column 2:
025
0 30
400
This second block would not be checked in an actual game of sudoku, but your function
should allow for it to be checked.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1e81da3a-685f-4a1c-b0d8-bdbcceea4042%2F78f685c7-a4a7-469c-b903-eea720e9a195%2Ft3uporw_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Please write a function named block_correct (sudoku: list, row_no: int,
column_no: int), which takes a two-dimensional array representing a sudoku grid, and
two integers referring to the row and column indexes of a single square, as its arguments.
Rows and columns are indexed from 0.
The function should return True or False depending on whether the 3 by 3 block to the
right and down from the given indexes is filled in correctly. That is, whether the block
contains each of the numbers 1 to 9 at most once.
Notice that this function does not strictly follow the rules of sudoku. In a real game of
sudoku there are only 9 blocks to check, and these are located at indexes (0, 0), (0, 3), (0,
6), (3, 0), (3, 3), (3, 6), (6, 0), (6, 3) and (6, 6). Such restrictions on indexes should not be
implemented here.
sudoku = [
[9, 0, 0, 0, 8, 0, 3, 0, 0],
[2, 0, 0, 2, 5, 0, 7, 0, 0],
[0, 2, 0, 3, 0, 0, 0, 0, 4],
[2, 9, 4, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 7, 3, 0, 5, 6, 0],
[7, 0, 5, 0, 6, 0, 4, 0, 0],
[0, 0, 7, 8, 0, 3, 9, 0, 0],
[0, 0, 1, 0, 0, 0, 0, 0, 3],
[3, 0, 0, 0, 0, 0, 0, 0, 21
]
print (block_correct (sudoku, 0, 0))
print (block_correct (sudoku, 1, 2))
False
True
The first function call should check the 3 by 3 block beginning with the square at indexes
0, 0:
900
200
020
Sample output
The second function call should check the 3 by 3 block beginning with the square at row 1,
column 2:
025
0 30
400
This second block would not be checked in an actual game of sudoku, but your function
should allow for it to be checked.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
Hi! I get all these error messages with this code. Can you help me?
![FAIL:
SudokuBlockTest: test_2_type_of_return_value
[[9, 0, 0, 0, 8, 0, 3, 0, 0], [2, 0, 0, 2, 5, 0, 7, 0,
0], [0, 2, 0, 3, 0, 0, 0, 0, 4], [2, 9, 4, 0, 0, 0, 0,
0, 0], [0, 0, 0, 7, 3, 0, 5, 6, 0], [7, 0, 5, 0, 6, 0,
4, 0, 0], [0, 0, 7, 8, 0, 3, 9, 0, 0], [0, 0, 1, 0, 0,
0, 0, 0, 3], [3, 0, 0, 0, 0, 0, 0, 0, 2]]
FAIL:
SudokuBlockTest: test_3_functionality
[[9, 0, 0, 0, 8, 0, 3, 0, 0], [2, 0, 0, 2, 5, 0, 7, 0,
0], [0, 2, 0, 3, 0, 0, 0, 0, 4], [2, 9, 4, 0, 0, 0, 4,
0, 0], [0, 0, 0, 7, 3, 0, 5, 6, 0], [7, 0, 5, 0, 6, 0,
4, 0, 0], [0, 0, 7, 8, 0, 3, 9, 0, 0], [0, 0, 1, 0, 0,
0, 0, 0, 3], [3, 0, 1, 0, 0, 8, 0, 0, 2]]](https://content.bartleby.com/qna-images/question/dca59d3b-f065-4790-9f9b-8546b85214d3/b664f75e-9c4e-4d97-a1c1-4238d41131b2/fbp5s7k_thumbnail.png)
Transcribed Image Text:FAIL:
SudokuBlockTest: test_2_type_of_return_value
[[9, 0, 0, 0, 8, 0, 3, 0, 0], [2, 0, 0, 2, 5, 0, 7, 0,
0], [0, 2, 0, 3, 0, 0, 0, 0, 4], [2, 9, 4, 0, 0, 0, 0,
0, 0], [0, 0, 0, 7, 3, 0, 5, 6, 0], [7, 0, 5, 0, 6, 0,
4, 0, 0], [0, 0, 7, 8, 0, 3, 9, 0, 0], [0, 0, 1, 0, 0,
0, 0, 0, 3], [3, 0, 0, 0, 0, 0, 0, 0, 2]]
FAIL:
SudokuBlockTest: test_3_functionality
[[9, 0, 0, 0, 8, 0, 3, 0, 0], [2, 0, 0, 2, 5, 0, 7, 0,
0], [0, 2, 0, 3, 0, 0, 0, 0, 4], [2, 9, 4, 0, 0, 0, 4,
0, 0], [0, 0, 0, 7, 3, 0, 5, 6, 0], [7, 0, 5, 0, 6, 0,
4, 0, 0], [0, 0, 7, 8, 0, 3, 9, 0, 0], [0, 0, 1, 0, 0,
0, 0, 0, 3], [3, 0, 1, 0, 0, 8, 0, 0, 2]]
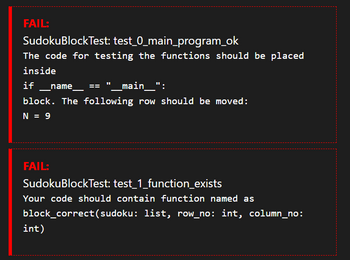
Transcribed Image Text:FAIL:
SudokuBlockTest: test_0_main_program_ok
The code for testing the functions should be placed
inside
if _name
_main___"
block. The following row should be moved:
N = 9
FAIL:
SudokuBlock Test: test_1_function_exists
Your code should contain function named as
block_correct (sudoku: list, row_no: int, column_no:
int)
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
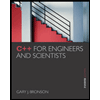
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
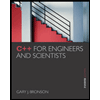
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr