Here is my entire code(average is not working yet). The response I got from the professor is that I have to create a DRY solution replacing the five display_functions with a single get_array function you call five times like common_array = get_array(plant_xml, "COMMON") etc. from urllib.request import urlopen from xml.etree.ElementTree import parse def read_data(data_file): plant_url = urlopen(data_file) plant_xml = parse(plant_url) return plant_xml def display_common_items(plant_xml): plant_root = plant_xml.getroot() common_array = [] for item in plant_root.findall("PLANT"): common_array.append(item.find("COMMON").text) return common_array def display_botanical_items(plant_xml): plant_root = plant_xml.getroot() botanical_array = [] for item in plant_root.findall("PLANT"): botanical_array.append(item.find("BOTANICAL").text) return botanical_array def display_zone_items(plant_xml): plant_root = plant_xml.getroot() zone_array = [] for item in plant_root.findall("PLANT"): zone_array.append(item.find("ZONE").text) return zone_array def display_light_items(plant_xml): plant_root = plant_xml.getroot() light_array = [] for item in plant_root.findall("PLANT"): light_array.append(item.find("LIGHT").text) return light_array def display_price_items(plant_xml): plant_root = plant_xml.getroot() price_array = [] for item in plant_root.findall("PLANT"): price_array.append(item.find("PRICE").text) return price_array def display_output(common_array,botanical_array, zone_array, light_array, price_array): for count in range(0, len(common_array)): count in range(0,len(botanical_array)) count in range(0,len(zone_array)) count in range(0,len(light_array)) count in range(0,len(price_array)) print(common_array[count] + "(" + botanical_array[count] + ")" + " - " + zone_array[count] +" - " + light_array[count] + " - " + price_array[count]) def calculate_average(price_array): sum = 0 count = 0 for item in price_array[:1]: try: sum += price_array[:1] count += 1 except ValueError: pass average = sum/count print(average) def main(): data_file = "https://www.w3schools.com/xml/plant_catalog.xml" plant_xml = read_data(data_file) common_array = display_common_items(plant_xml) botanical_array = display_botanical_items(plant_xml) zone_array = display_zone_items(plant_xml) light_array = display_light_items(plant_xml) price_array = display_price_items(plant_xml) display_output(common_array,botanical_array,zone_array,light_array,price_array) calculate_average(price_array)
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Here is my entire code(average is not working yet). The response I got from the professor is that I have to create a DRY solution replacing the five display_functions with a single get_array function you call five times like common_array = get_array(plant_xml, "COMMON") etc.
from urllib.request import urlopen
from xml.etree.ElementTree import parse
def read_data(data_file):
plant_url = urlopen(data_file)
plant_xml = parse(plant_url)
return plant_xml
def display_common_items(plant_xml):
plant_root = plant_xml.getroot()
common_array = []
for item in plant_root.findall("PLANT"):
common_array.append(item.find("COMMON").text)
return common_array
def display_botanical_items(plant_xml):
plant_root = plant_xml.getroot()
botanical_array = []
for item in plant_root.findall("PLANT"):
botanical_array.append(item.find("BOTANICAL").text)
return botanical_array
def display_zone_items(plant_xml):
plant_root = plant_xml.getroot()
zone_array = []
for item in plant_root.findall("PLANT"):
zone_array.append(item.find("ZONE").text)
return zone_array
def display_light_items(plant_xml):
plant_root = plant_xml.getroot()
light_array = []
for item in plant_root.findall("PLANT"):
light_array.append(item.find("LIGHT").text)
return light_array
def display_price_items(plant_xml):
plant_root = plant_xml.getroot()
price_array = []
for item in plant_root.findall("PLANT"):
price_array.append(item.find("PRICE").text)
return price_array
def display_output(common_array,botanical_array, zone_array, light_array, price_array):
for count in range(0, len(common_array)):
count in range(0,len(botanical_array))
count in range(0,len(zone_array))
count in range(0,len(light_array))
count in range(0,len(price_array))
print(common_array[count] + "(" + botanical_array[count] + ")" + " - " + zone_array[count] +" - " + light_array[count] + " - " + price_array[count])
def calculate_average(price_array):
sum = 0
count = 0
for item in price_array[:1]:
try:
sum += price_array[:1]
count += 1
except ValueError:
pass
average = sum/count
print(average)
def main():
data_file = "https://www.w3schools.com/xml/plant_catalog.xml"
plant_xml = read_data(data_file)
common_array = display_common_items(plant_xml)
botanical_array = display_botanical_items(plant_xml)
zone_array = display_zone_items(plant_xml)
light_array = display_light_items(plant_xml)
price_array = display_price_items(plant_xml)
display_output(common_array,botanical_array,zone_array,light_array,price_array)
calculate_average(price_array)
main()

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

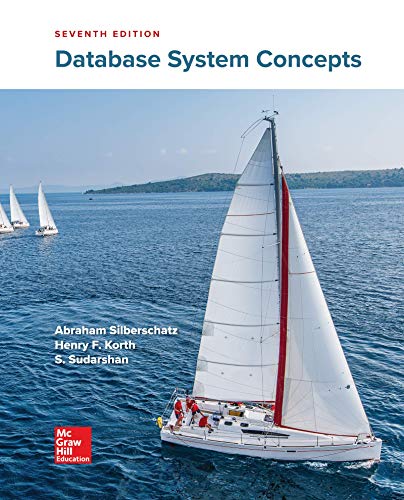
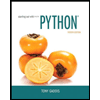
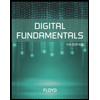
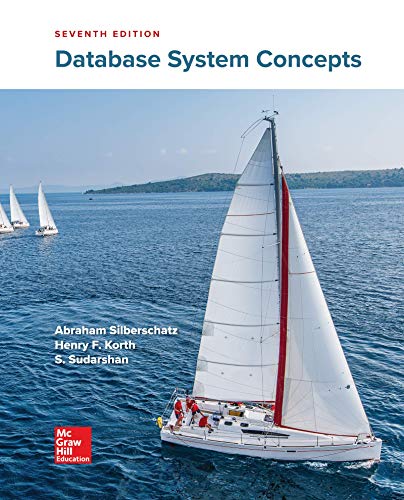
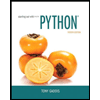
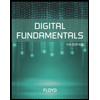
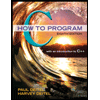
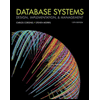
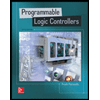