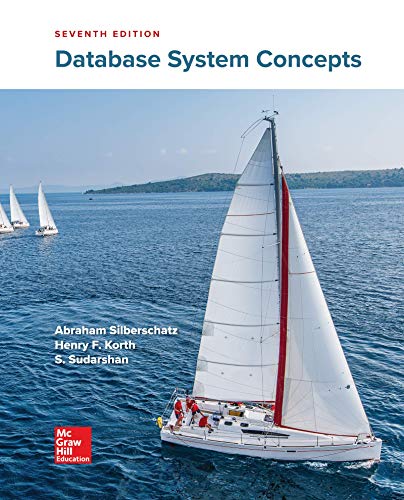
Concept explainers
Please written by computer source
const int ROW_SIZE = 10; const int COLUMN_SIZE = 10; int myMatrix[ROW_SIZE][COLUMN_SIZE];
Note that these constants will be in main and not in the scope of any other function. That is, you must design your functions to have parameters to receive the dimensions.
Write a function called initialize that receives a 2D array and initializes the array with random values between 0 and 99. Add a message that confirms the successful creation of the matrix. Call this function in main to initialize myMatrix.
Write a function called display that receives a 2D array and displays the elements of the array in a tabular format. Arrange the method to display row and column numbers. Row indices displayed on the left and column indices displayed at the top. Call this function in main to print myMatrix.
Write a function called sumEven that receives a 2D array and returns the sum of all the even elements. Call this function in main to calculate and display this value for myMatrix.
Write a function called sum2Diagonal that receives a 2D array and sums all the values in the secondary diagonal (Top right to bottom left). Call this function in main to calculate this value for myMatrix.
Write a function called sumColumn that receives a 2D array and a column number. The function returns the sum of all the elements in that column. Call this function in main to calculate and display this value for the last column of myMatrix.
Write a function called largestSum that receives a 2D array and returns the number of the row with the largest sum of elements. Call this function in main to calculate and display this value for myMatrix.
Write a function called transpose that receives a 2D array and transposes the array. The transposed matrix will be stored in another 2D array. For comparison print both matrices. Call this function in main to transpose myMatrix.

Step by stepSolved in 3 steps with 7 images

- In C++ language Write a function that takes a 2 Demensional array and returns the position of the first row with an odd sum. Assume that the column size is fixed at 4. If no sum is odd, return -1.arrow_forwardThe code should be in C and not hard-coded. It should use 2D arrays.arrow_forwardMatrix Addition• Write an addition function that accepts two 2D numpy arrays, andreturns the sum of the two (if they are the same size). This functionshould test that the arrays are the same size before performing theaddition. If the arrays are not the same size, the function shouldreturn -1. solve in pythonarrow_forward
- Matrix Multiplication• Write a multiplication function that accepts two 2D numpy arrays,and returns the product of the two. This function should test that thearrays are compatible (recall that the number of columns in the firstmatrix must equal the number of rows in the second matrix). Thisfunction should test the array sizes before attempting themultiplication. If the arrays are not compatible, the function shouldreturn -1. solve in pythonarrow_forwardCreate a function named "onlyOdd".The function should accept a parameter named "numberArray".Use the higher order function filter() to create a new array that only containsthe odd numbers. Return the new array.// Use this array to test your function:const testingArray = [1, 2, 4, 17, 19, 20, 21];arrow_forwarduse c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forward
- javascript only: This quarter, your team manager is awarding commission to anyone who closes a sale worth $15,000 or more! The commission amount will be 10% of the value of their largest sale. Anyone who does not make this large of a sale will receive the base commission of $500. Write a function `salesCommission(arr)` that takes an array of objects containing salesperson names and their largest sale, and returns the total amount of commission money that needs to be paid out to the team. Example: salespeople = [ { name: "Susan", largestSale: 3000 }, { name: "Brianna", largestSale: 20000 }, { name: "Dwayne", largestSale: 13000 }, { name: "Delilah", largestSale: 26000 }, { name: "Fernando", largestSale: 8000 }, ]; console.log(salesCommission(salespeople)); // 6100arrow_forwardYou are designing a function that will manipulate an array of integers. It needs to work for arrays of any length. What is the minimum number of parameters your function will have?arrow_forwardwrite in html and scriptarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
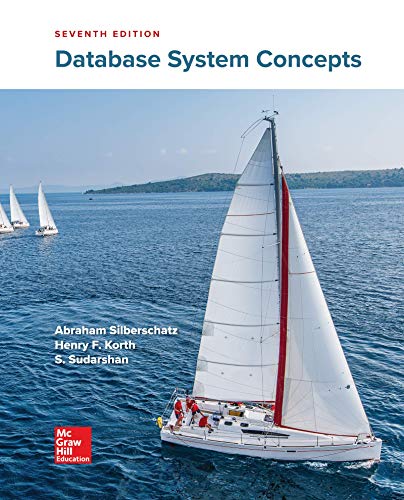
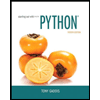
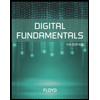
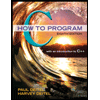
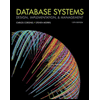
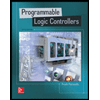