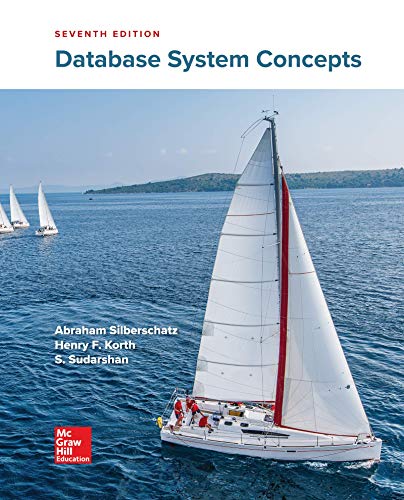
EXPLAIN THIS CODE EACH LINE
#include <stdio.h>
#include<string.h>
int main()
{
char name[20];
int meal,quantity;
int drink,quantity2;
char choose_meal[30] = "";
int meal_price;
float drink_price, orderPrice;
char choose_drink[30] = "";
float cash, change;
printf("--------------------Marry's Fast Food--------------------");
printf("\n");
printf("Enter your name: ");
scanf("%s", name);
printf("Hello %s!\n", name);
printf("\n\nWhat would you like to order?\n");
printf("------------------------MEALS------------------------\n\n");
printf("[1] 1 pc. Chicken Joy....................Php 76.00\n");
printf("[2] 1 pc. w/Jolly spaghetti..............Php 99.00\n");
printf("[3] pc. Spaghetti........................Php 55.00\n");
printf("[4] pc. Burger steak w/rice..............Php 85.00\n");
printf("[5] pc. Burger...........................Php 30.00\n\n");
printf("Choose a number for your meal: ");
scanf("%d", &meal);
printf("Quantity: ");
scanf("%d", &quantity);
printf("-----------------------DRINKS-----------------------\n\n");
printf("[1] Coke.................................Php 15.20\n");
printf("[2] Sprite...............................Php 15.25\n");
printf("[3] Royal................................Php 15.30\n");
printf("[4] Pineapple............................Php 20.25\n");
printf("[5] Iced Tea.............................Php 20.25\n\n");
printf("Choose a number for your drink: ");
scanf("%d", &drink);
printf("Quantity: ");
scanf("%d", &quantity2);
if(meal==1){
strcpy(choose_meal,"Chicken Joy");
meal_price = 76;
}
else if(meal==2){
strcpy(choose_meal,"w/Jolly spaghetti");
meal_price = 99;
}
else if(meal==3){
strcpy(choose_meal,"Spaghetti");
meal_price = 55;
}
else if(meal==4){
strcpy(choose_meal,"Burger steak w/rice");
meal_price = 85;
}
else{
strcpy(choose_meal, "Burger");
meal_price = 30;
}
if(drink==1){
strcpy(choose_drink, "Coke");
drink_price = 15.20;
}
else if(drink==2){
strcpy(choose_drink,"Sprite");
drink_price = 15.25;
}
else if(drink==3){
strcpy(choose_drink, "Royal");
drink_price = 15.30;
}
else if(drink==4){
strcpy(choose_drink, "Pineapple");
drink_price = 20.50;
}
else{
strcpy(choose_drink, "Iced Tea");
drink_price = 20.75;
}
printf("---------------------ITEM ORDERED---------------------\n\n");
printf("%s.....................Php %d x %d = %d",choose_meal,meal_price,quantity,meal_price*quantity);
printf("\n");
printf("%s.....................Php %f x %d = %f",choose_drink,drink_price,quantity2,drink_price*quantity2);
orderPrice = meal_price*quantity+drink_price*quantity2;
printf("\n");
printf("Total amount is %f",orderPrice );
printf("\nCash: " );
scanf("%f", &cash);
if (orderPrice <= cash )
{
printf("Change: %f", cash-meal_price*quantity+drink_price*quantity2);
printf("\n\n");
printf("---------------------------------------------------\n\n");
printf(" THANK YOU ENJOY THE MEAL! \n\n");
printf("---------------------------------------------------\n");
scanf("%f", &change);
}
else
{
printf("Please give enough money.");
}
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- /* Movie List Example --Showing how to use vectors and structures */ #include <iostream> #include <iomanip> #include <string> #include <vector> using namespace std; // define a struct for a Movie object struct Movie // It is common for the struct name to be capitalized { string title = ""; // First member of structure - and initialized int year = 0; // Second member of structure - and initialized }; int main() { cout << "The Movie List program\n\n" << "Enter a movie...\n\n"; // get vector of Movie objects vector<Movie> movie_list; char another = 'y'; while (tolower(another) == 'y') { Movie movie; // make temporary new (initialized) Movie object cout << "Title: "; getline(cin, movie.title); cout << "Year: "; cin >> movie.year; movie_list.push_back(movie);…arrow_forward#include <iostream> #include <iomanip> #include <string> #include <vector> using namespace std; class Movie { private: string title = ""; int year = 0; public: void set_title(string title_param); string get_title() const; // "const" safeguards class variable changes within function string get_title_upper() const; void set_year(int year_param); int get_year() const; }; // NOTICE: Class declaration ends with semicolon! void Movie::set_title(string title_param) { title = title_param; } string Movie::get_title() const { return title; } string Movie::get_title_upper() const { string title_upper; for (char c : title) { title_upper.push_back(toupper(c)); } return title_upper; } void Movie::set_year(int year_param) { year = year_param; } int Movie::get_year() const { return year; } int main() { cout << "The Movie List program\n\n"…arrow_forwardFill in the blanksarrow_forward
- C++ complete and create magical square #include <iostream> using namespace std; class Vec {public:Vec(){ }int size(){return this->sz;} int capacity(){return this->cap;} void reserve( int n ){// TODO:// (0) check the n should be > size, otherwise// ignore this action.if ( n > sz ){// (1) create a new int array which size is n// and get its addressint *newarr = new int[n];// (2) use for loop to copy the old array to the// new array // (3) update the variable to the new address // (4) delete old arraydelete[] oldarr; } } void push_back( int v ){// TODO: if ( sz == cap ){cap *= 2; reserve(cap);} // complete others } int at( int idx ){ } private:int *arr;int sz = 0;int cap = 0; }; int main(){Vec v;v.reserve(10);v.push_back(3);v.push_back(2);cout << v.size() << endl; // 2cout << v.capacity() << endl; // 10v.push_back(3);v.push_back(2);v.push_back(3);v.push_back(4);v.push_back(3);v.push_back(7);v.push_back(3);v.push_back(8);v.push_back(2);cout…arrow_forwardPart-2 Debugging Debug#0int main(){char* ptr = nullptr;int size; cout << "Enter the size of the sentence: "; cin >> size; ptr = new char[size];char* ptr2 = ptr; cout << static_cast<void*>(&ptr) << endl;cout << static_cast<void*>(&ptr2) << endl; cout << "\nEnter your sentence:" << endl;for (int i = 0; i < size; i++){cin >> *(ptr + i);} cout << *ptr << endl;cout << ptr[size - 1]; while (static_cast<void*>(&ptr) <= static_cast<void*>(&ptr[size -1])){cout << "HI" << endl;cout << static_cast<void*>(&ptr) << endl;static_cast<void*>(&ptr);ptr++;} }arrow_forwardWrite a function getNeighbors which will accept an integer array, size of the array and an index as parameters. This function will return a new array of size 2 which stores the neighbors of the value at index in the original array. If this function would result in returning garbage values the new array should be set to values {0,0} instead of values from the array.arrow_forward
- struct gameType { string title; int numPlayers; bool online; }; gameType Awesome[50]; Write the C++ statement that sets the first [0] title of Awesome to Best.arrow_forwardC++ complete magic Square #include <iostream> using namespace std; /*int f( int x, int y, int* p, int* q ){if ( y == 0 ){p = 0, q = 0;return 404; // status: Error 404}*p = x * y; // product*q = x / y; // quotient return 200; // status: OK 200} int main(){int p, q;int status = f(10, 2, &p, &q);if ( status == 404 ){cout << "[ERR] / by zero!" << endl;return 0;}cout << p << endl;cout << q << endl; return 0;}*/ /*struct F_Return{int p;int q;int status;}; F_Return f( int x, int y ){F_Return r;if ( y == 0 ){r.p = 0, r.q = 0;r.status = 404;return r;}r.p = x * y;r.q = x / y;r.status = 200;return r;} int main(){F_Return r = f(10, 0);if ( r.status == 404 ){cout << "[ERR] / by zero" << endl;return 0;}cout << r.p << endl;cout << r.q << endl;return 0;}*/ int sumByRow(int *m, int nrow, int ncol, int row){ int total = 0;for ( int j = 0; j < ncol; j++ ){total += m[row * ncol + j]; //m[row][j];}return total; } /*…arrow_forwardC++ program #include<iostream> #include<fstream> #include<string> using namespace std; const int NAME_SIZE = 20; const int STREET_SIZE = 30; const int CITY_SIZE = 20; const int STATE_CODE_SIZE = 3; struct Customers { long customerNumber; char name[NAME_SIZE]; char streetAddress_1[STREET_SIZE]; char streetAddress_2[STREET_SIZE]; char city[CITY_SIZE]; char state[STATE_CODE_SIZE]; int zipCode; char isDeleted; char newLine; }; void add_data(int no_of_records, ofstream& fout) { struct Customers c; cout << "Enter the Customer data:" << endl; cout << "Name:"; cin >> c.name; cout << "Street Address 1:"; cin >> c.streetAddress_1; cout << "Street Address 2:"; cin >> c.streetAddress_2; cout << "City:"; cin >> c.city; cout << "State:"; cin >> c.state; cout << "Zip Code:"; cin >> c.zipCode; cout << endl; c.customerNumber = no_of_records; c.isDeleted = 'N'; c.newLine = '\n'; cout…arrow_forward
- Please explain this question void main() {int a =300; char *ptr = (char*) &a ; ptr ++; *ptr =2; printf("%d", a); }arrow_forward#ifndef INT_SET_H#define INT_SET_H #include <iostream> class IntSet{public: static const int DEFAULT_CAPACITY = 1; IntSet(int initial_capacity = DEFAULT_CAPACITY); IntSet(const IntSet& src); ~IntSet(); IntSet& operator=(const IntSet& rhs); int size() const; bool isEmpty() const; bool contains(int anInt) const; bool isSubsetOf(const IntSet& otherIntSet) const; void DumpData(std::ostream& out) const; IntSet unionWith(const IntSet& otherIntSet) const; IntSet intersect(const IntSet& otherIntSet) const; IntSet subtract(const IntSet& otherIntSet) const; void reset(); bool add(int anInt); bool remove(int anInt); private: int* data; int capacity; int used; void resize(int new_capacity);}; bool operator==(const IntSet& is1, const IntSet& is2); #endifarrow_forwardint main(){ long long int total; long long int init; scanf("%lld %lld", &total, &init); getchar(); long long int max = init; long long int min = init; int i; for (i = 0; i < total; i++) { char op1 = '0'; char op2 = '0'; long long int num1 = 0; long long int num2 = 0; scanf("%c %lld %c %lld", &op1, &num1, &op2, &num2); getchar(); long long int maxr = max; long long int minr = min; if (op1 == '+') { long long int sum = max + num1; maxr = sum; minr = sum; long long int res = min + num1; if (res > maxr) { max = res; } if (res < minr) { minr = res; } } else { long long int sum = max * num1; maxr = sum; minr = sum; long long int res = min * num1;…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
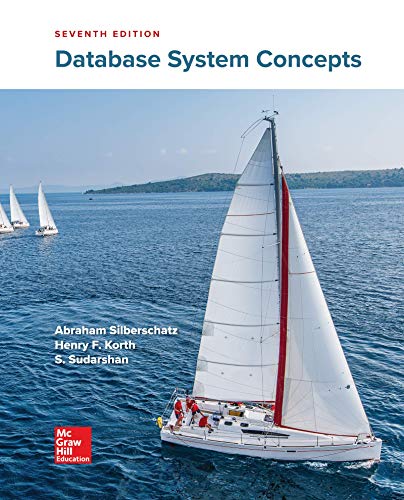
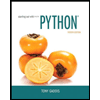
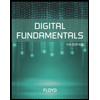
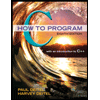
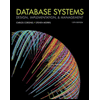
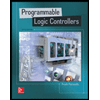