Hi l would appreciate the help of this project in java . Part 1 The zip file my_zoo_p1.zip is on BB under the Resit folder in Programming 2. The file contains two Java classes, MyZoo and Animal, and two text files import001.txt and import002.txt containing "animal" data. Download these into your filespace. Although the MyZoo and Animal classes are similar to the classes in the original Zoo project, there are some important differences. For example, the Animal objects in the zoo are stored in a TreeMap rather than an ArrayList. Animals are added to the TreeMap using the put() method which requires a unique key value to be specified (similar to the HashMap discussed in Chapter 6). You can examine the documentation for TreeMap if you wish, but no detailed knowledge of this class is required for this project. Another difference is that the MyZoo class has a three letter identifier, provided to the constructor, and a method allocateId() for creating unique identifiers for animals of the zoo. Part 1 of this project is basically concerned with completing methods printAllAnimals() and readDataFromFile(). The readDataFromFile() method uses a FileDialog object so that you can easily select a file to read data from, but the code for reading the data is missing. However, if you create a MyZoo object and execute the readDataFromFile() then you should see a message stating that 10 animals have been read from the file (but remember that a common problem is that the "open file" dialog gets hidden behind other windows). It doesn't matter which input file you select as no data is read from the file, instead some "temporary" code will "populate" the zoo. After executing readDataFromFile(), if you next execute the printAllAnimals() method then only a "header" will be printed -- it will not actually show any animals as this method is also incomplete. Proceed by completing the following stages. Note that no changes should be needed for class Animal. Step 1: Complete the method printAllAnimals() using the Collection object c, provided in the code, so that animals stored in the zoo can easily be checked. Note that when you complete this method, the animals are listed in alphabetical order by name. This is because the values() method will return a collection with the animals in alphabetical order by name since the key values for the TreeMap are the names of the Animal objects: see method addAnimal(). Step 2: Start the development of the method readDataFromFile() by first deleting or "commenting out" the temporary code. You should proceed in the following two steps. (i) You will need a loop and you should first concentrate on successfully reading the data from the file. At this step do not attempt to create an Animal object from the data. Here is the basic structure needed: read an initial line of text into a string while there is something in the file print out the string using System.out.println() read the next line You might also need to consult slides SM5 on how to handle exceptions. Get this working, reading from import001.txt and showing the original data, before proceeding to the next step. (ii) The data in the text file is "tab separated" and looks like this: Bird Golden Eagle Eddie Spaces at the beginning or end of the line should be ignored. You should extract the three substrings and then create and add an Animal object to the zoo. You should ignore the first substring ("Bird" in the above example) as it is not required for this part of this project. Also note that you should introduce an extra parameter - representing the owner of the collection – for the constructor of your Animal object. Its value in this case will be "this". On successful completion of this step you will have a "basic" working version of readDataFromFile(). Step 3: A refinement of method readDataFromFile(). In these next stages, the data file you should use is import002.txt where the first line of data in the text file provides some information about the animals e.g. import shipment of June 30, 2023 So, amend your code so that this first line is not treated as "animal" data but is printed out to the terminal window. Also, subsequent lines of text may include "comment lines" that start with the # symbol e.g. # this line is a comment Such lines should be ignored when reading data from the file. Step 4: If you now "test" your project by reading from the file import002.txt then you might notice that although two animals named Eddie are read from the file, only one appears in the listing if you execute printAllAnimals(). This is because we have used a Map object TreeMap and the call to put() in addAnimal() uses the name field and consequently the second Eddie "replaces" the first one. Solve this problem without losing the alphabetical ordering by name. This is a very simple task once you see what is needed, but you should first re-read Part 1 from the beginning. Hint: currently the "key" used for each animal, in the put() method, is its name -- look at the code for the Animal class and think about how a unique key for each animal could be obtained; just one line of code (in the MyZoo class) needs modifying !

Step by step
Solved in 3 steps

Hi can I get help for java code for this project
Part 1 of this project is basically concerned with completing methods printAllAnimals() and readDataFromFile(). The readDataFromFile() method uses a FileDialog object so that you can easily select a file to read data from, but the code for reading the data is missing. However, if you create a MyZoo object and execute the readDataFromFile() then you should see a message stating that 10 animals have been read from the file (but remember that a common problem is that the "open file" dialog gets hidden behind other windows). It doesn't matter which input file you select as no data is read from the file, instead some "temporary" code will "populate" the zoo. After executing readDataFromFile(), if you next execute the printAllAnimals() method then only a "header" will be printed -- it will not actually show any animals as this method is also incomplete. Proceed by completing the following stages. Note that no changes should be needed for class Animal. Step 1: Complete the method printAllAnimals() using the Collection object c, provided in the code, so that animals stored in the zoo can easily be checked. Note that when you complete this method, the animals are listed in alphabetical order by name. This is because the values() method will return a collection with the animals in alphabetical order by name since the key values for the TreeMap are the names of the Animal objects: see method addAnimal(). Step 2: Start the development of the method readDataFromFile() by first deleting or "commenting out" the temporary code. You should proceed in the following two steps. (i) You will need a loop and you should first concentrate on successfully reading the data from the file. At this step do not attempt to create an Animal object from the data. Here is the basic structure needed: read an initial line of text into a string while there is something in the file print out the string using System.out.println() read the next line You might also need to consult slides SM5 on how to handle exceptions. Get this working, reading from import001.txt and showing the original data, before proceeding to the next step. (ii) The data in the text file is "tab separated" and looks like this: Bird Golden Eagle Eddie Spaces at the beginning or end of the line should be ignored. You should extract the three substrings and then create and add an Animal object to the zoo. You should ignore the first substring ("Bird" in the above example) as it is not required for this part of this project. Also note that you should introduce an extra parameter - representing the owner of the collection – for the constructor of your Animal object. Its value in this case will be "this". On successful completion of this step you will have a "basic" working version of readDataFromFile(). Step 3: A refinement of method readDataFromFile(). In these next stages, the data file you should use is import002.txt where the first line of data in the text file provides some information about the animals e.g. import shipment of June 30, 2023 So, amend your code so that this first line is not treated as "animal" data but is printed out to the terminal window. Also, subsequent lines of text may include "comment lines" that start with the # symbol e.g. # this line is a comment Such lines should be ignored when reading data from the file. Step 4: If you now "test" your project by reading from the file import002.txt then you might notice that although two animals named Eddie are read from the file, only one appears in the listing if you execute printAllAnimals(). This is because we have used a Map object TreeMap and the call to put() in addAnimal() uses the name field and consequently the second Eddie "replaces" the first one. Solve this problem without losing the alphabetical ordering by name.
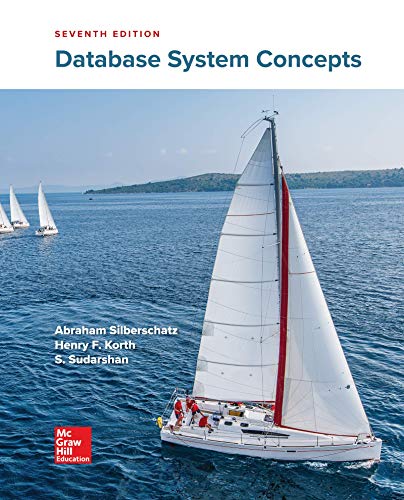
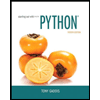
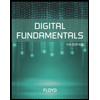
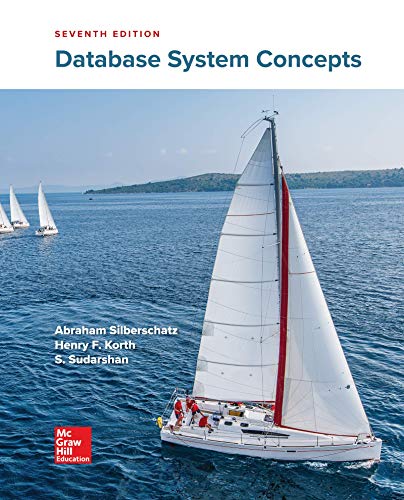
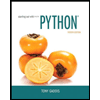
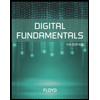
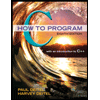
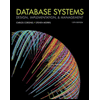
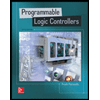