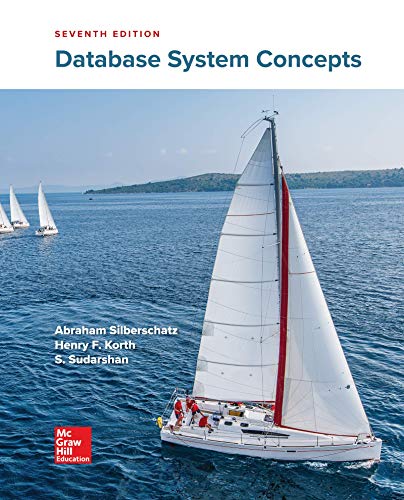
Matrix.h
#include <iostream>
#include <iomanip>
using namespace std;
#ifndef MATRIX_H
#define MATRIX_H
class Matrix
{
friend ostream& operator<<(ostream& out, const Matrix& srcMatrix);
public:
Matrix();
// initialize Matrix class object with rowN=1, colN=1, and zero value
Matrix(const int rN,const int cN );
// initialize Matrix class object with row number rN, col number cN and zero values
Matrix(const Matrix &srcMatrix );
// initialize Matrix class object with another Matrix class object
Matrix(const int rN, const int cN, const float *srcPtr);
// initialize Matrix class object with row number rN, col number cN and a pointer to an array
const float * getData()const;
// create a temp pointer and copy the array values which data pointer points,
// then returns temp.
int getRowN()const; // returns rowN
int getColN()const; // returns colN
void print()const; // prints the Matrix object in rowNxcolN form
Matrix transpose(); // takes the transpose of the matrix
Matrix operator+(const Matrix &rhsMatrix)const;
//+ operator which allows m1+m2 and returns a temp Matrix object
Matrix operator-(const Matrix &rhsMatrix)const;
//- operator which allows m1-m2 and returns a temp Matrix object
Matrix operator*(const Matrix &rhsMatrix)const;
//* operator which allows product of m1*m2
//(element-wise) and returns a temp Matrix object
float operator()(const int r,const int c)const;
//() operator which allows returning m1(r,c),
//m1(0,0) is on the first row, first col
Matrix& operator=(const Matrix &rhsMatrix);
//= operator which allows m1=m2 and returns this pointer of m1
Matrix& operator+=(const Matrix &rhsMatrix);
//+= operator which allows m1+=m2 and returns this pointer of m1
Matrix& operator-=(const Matrix &rhsMatrix);
//-= operator which allows m1-=m2 and returns this pointer of m1
Matrix& operator*=(const Matrix &rhsMatrix);
//*= operator which allows m1*=m2 (element-wise) and returns this pointer of m1
int operator==(const Matrix &rhsMatrix)const;
//== operator which returns 1 if (m1==m2)
int operator!=(const Matrix &rhsMatrix)const;
//== operator which returns 1 if (m1!=m2)
private:
//() operator which allows returning m1(r,c),
//m1(0,0) is on the first row,first col
int rowN, colN;
float* data;
// e.g: if pointer data points an array of [ 1, 2, 3, 4, 5, 6] and the rowN=3 and coln=2
// matrix is actually
// 1 2 3
// 4 5 6
};
#endif
testMatix.cpp
#include "Matrix.h"
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
float arr1[]={1,2,3,4,5, 11,12,13,14,15, 21,22,23,24,25};
float arr2[]={9,8,7,6,5, 19,18,17,16,15, 29,28,27,26,25};
Matrix m1(3,5);
Matrix m2(m1);
Matrix m3(3,5,arr1);
Matrix m4(3,5,arr2);
//-------------------
cout<<"m1(3,5)"<<endl;
m1.print();
cout<<"m2(m1)"<<endl;
m2.print();
cout<<"m3(3,5,arr1)"<<endl;
m3.print();
cout<<"m3.transpose()"<<endl;
m3.transpose().print();
cout<<"m4(3,5,arr2)"<<endl;
m4.print();
//------------------
cout<<"elements=m3.getData()"<<endl;
const float* const elements=m3.getData();
const int rN=m3.getRowN();
const int cN=m3.getColN();
for (int i=0; i<rN; i++)
{
for (int j=0; j<cN; j++)
cout<<setw(4)<<elements[i*cN+j];
cout<<"\n";
}
//-------------------
cout<<"m3+=m4"<<endl;
(m3+=m4).print();
cout<<"m3-=m4"<<endl;
(m3-=m4).print();
cout<<"m3+m4"<<endl;
(m3+m4).print();
cout<<"m3"<<endl;
m3.print();
cout<<"m3=m3+m4"<<endl;
(m3=m3+m4).print();
cout<<"m3"<<endl;
m3.print();
cout<<"m3(2,2)="<<m3(2,2)<<endl<<endl;
//---------------
(m1==m2)?cout<<"m1==m2\n"<<endl:cout<<"m1!=m2\n"<<endl;
(m3!=m4)?cout<<"m3!=m4\n"<<endl:cout<<"m3==m4\n"<<endl;
cout<<"(m4*m4)\n";
(m4*m4).print();
//---------------
//cout<<"(m4*m4)\n";
//cout << (m4*m4) << endl;
return 0;
}
![1. Using the header file Matrix.h and testMatrix.cpp (check the course webpage)
a) Type the implementation file Matrix.cpp, compile your files and get an application file.
Hint: the use of the float* data pointer for a matrix object can be understood with the help of the following empty
constructor function
2
Matrix::Matrix ()
// initialize Matrix class object with rowN=1, colN=1, and zero value
{
}
rowN=1;
colN=1;
data=new float [rowN*colN];
for (int i=0; i<rowN; i++)
for (int j = 0; j<colN; j++)
data[i*colN+j]=0;
If colN=3 and rowN
m (i, j) will be data[i*3+j]
m (0,0), data [0*3+0] m (0,1), data [0*3+1] m (0,2), data [0*3+2]
m (1,0), data [1*3+0] m (1,1), data [1*3+1] m (1,2), data [1*3+2]
m (2,0), data [2*3+0] m (2,1), data [2*3+1] m (2,2), data [2*3+2]
Compile your codes, run your application file, and save the screen image of the shell window.](https://content.bartleby.com/qna-images/question/70e43fc0-c98a-445d-bb4d-ca94b1343065/9f1c7ce5-463c-43c1-97f8-8416bcc57bc1/pbfqr7n_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 11 images

- Declare a student structure that contains : Student's first and last nameStudent IDCreate the following functions: getStudentInfo(void) Declares a single student, uses printf()/scanf() to get inputReturns the single student backprintStudentInfo(student_t *st_ptr) Takes the pointer to a student (to avoid making a copy)Prints out all of the student informationDeclare an array of five studentsUsing a for loop and getStudentInfo() function, get input of all the studentsUsing a for loop and printStudentInfo() function, print all the output of all students.arrow_forwardComputer Science csv file "/dsa/data/all_datasets/texas.csv" Task 6: Write a function "county_locator" that allows a user to enter in a name of a spatial data frame and a county name and have a map generated that shows the location of that county with respect to other counties (by using different colors and/or symbols). Hint: function(); $county ==; plot(); add=TRUE.arrow_forwardtypedef _people { int age; char name[ 32 ] ; } People_T ; People_T data [ 3 ]; Using string lib function, Assign 30 and Cathy to the first cell, Assign 40 and John to the second cell and Assign 50 and Tom to the third cell People_T *ptr ; Declare a pointer pointing to the structure data and print the age and name using the pointer. your output can be : 30 Cathy 40 John 50 Tomarrow_forward
- # dates and times with lubridateinstall.packages("nycflights13") library(tidyverse)library(lubridate)library(nycflights13) Qustion: Create a function called date_quarter that accepts any vector of dates as its input and then returns the corresponding quarter for each date Examples: “2019-01-01” should return “Q1” “2011-05-23” should return “Q2” “1978-09-30” should return “Q3” Etc. Use the flight's data set from the nycflights13 package to test your function by creating a new column called quarter using mutate()arrow_forwardAlert dont submit AI generated answer.arrow_forwardGame of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
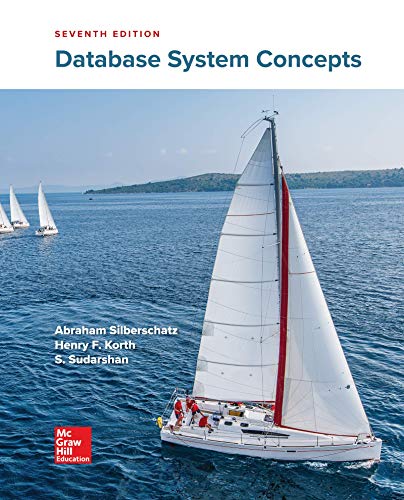
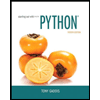
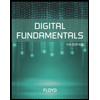
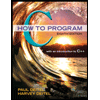
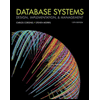
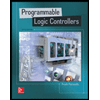