How do I fix this code in order for it to run? BusFareHandler.java package BusFare; public interface BusFareHandler { /** * Initializes your available change. * * * create a MaxHeap with a 1,5,10,25,50,100 coin randomly inserted */ public void grabChange(); /** * get the largest ( in this case the 100 cent ) coin from your maxHeap * * @return max */ public int getMaxFromHeap(); /** * (1) The param for this method should be the result returned from getMaxFromHeap() * * (2) RECURSIVSELY build up the bus fare. * * * (3) Constraint: Bus only accepts 5,25,50,100 cent coins, but you should have other coins in your collection. * * * @param coin */ public int payBusFare(int coin); //week1 test recursive call by selecting next element out of a sorted array instead of calling getMaxFromHeap() } BusFareHandlerImpl.java package BusFare; import MaxHeap.MaxHeap; import MaxHeap.MaxHeapImpl; import java.util.Random; public class BusFareHandlerImpl implements BusFareHandler { private MaxHeap changeHeap; //this method loads the maxheap with random coins - nickel thru silver dollar public void grabChange() { changeHeap = new MaxHeapImpl(); int[] coins = new int[] {1, 5, 10, 25, 50, 100}; Random rand = new Random(); for (int coin : coins) { int randNum = rand.nextInt(2); for (int i = 0; i < randNum; i++) { changeHeap.insert(coin); } } // TODO Auto-generated method stub } //this method uses my MaxHeap instance to get the largest coin public int getMaxFromHeap() { return changeHeap.extractMax(); } // this method (recursive method) retrieves the next coin public int payBusFare(int coin) { if (coin == 0) { return 0; } else if (coin == 100 || coin == 50 || coin == 25 || coin == 5) { changeHeap.delete(coin); return coin + payBusFare(getMaxFromHeap() - coin); } else { return payBusFare(getMaxFromHeap() - coin); } } } BusFareTester.java package BusFare; public class BusFareTester { public static void main(String[] args) { BusFareHandler busFare = new BusFareHandlerImpl(); testBusFareHandler(busFare); } private static void testBusFareHandler (BusFareHandler fare) { fare.grabChange(); int initialCoin = fare.getMaxFromHeap(); System.out.println(" Exact Fare paid: " + fare.payBusFare(initialCoin) + " cents "); } } MaxHeap.java package MaxHeap; public interface MaxHeap { public void insert(int element); public void display(); public int extractMax(); public void delete(int coin); } MaxHeapImpl.java package MaxHeap; public class MaxHeapImpl implements MaxHeap{ private int[] Heap; private int size; private int maxsize; public MaxHeapImpl(int maxsize) { this.maxsize = maxsize; this.size = size; Heap = new int[this.maxsize + 1]; Heap[0] = Integer.MAX_VALUE; } private int parent(int pos) { return pos/2;} private int leftChild(int pos) {return pos*2;} private int rightChild(int pos) {return (pos*2) + 1;} private void swap(int fpos, int spos) { int tmp; tmp = Heap[fpos]; Heap[fpos] = Heap[spos]; Heap[spos] = tmp; } private void downheapify(int pos) { if (pos >= (size / 2) && pos <= size) return; if (Heap[pos] < Heap[leftChild(pos)] || Heap[pos] < Heap[rightChild(pos)]) { if (Heap[leftChild(pos)] > Heap[rightChild(pos)]) { swap(pos, leftChild(pos)); downheapify(leftChild(pos)); } else { swap(pos, rightChild(pos)); downheapify(rightChild(pos)); } } } private void heapifyUp(int pos) { int tmp = Heap[pos]; while(pos>0 && tmp > Heap[parent(pos)]) { Heap[pos] = Heap[parent(pos)]; pos = parent(pos); } Heap[pos] = tmp; } public void insert(int element) { Heap[++size] = element; int tmp = size; while (Heap[tmp] > Heap[parent(tmp)]) { swap(tmp, parent(tmp)); tmp = parent(tmp); } } public void display() { for (int i = 1; i <= size / 2; i++) { System.out.println("Parent: " + Heap[i] + " Left Child: " + Heap[2 * i] + " Right Child: " + Heap[2 * i + 1]); System.out.println(); } } public int extractMax() { int max = Heap[1]; Heap[1] = Heap[size--]; Heap[size+1] = 0; downheapify(1); return max; } public void delete(int coin) { } } MaxHeapTester.java package MaxHeap; public class MaxHeapTester { public static void main(String[] args) { // MaxHeap theHeap = new MaxHeapImpl(15); // runTests(theHeap); } public static void runTests(MaxHeap theHeap) { } }
How do I fix this code in order for it to run?
BusFareHandler.java
package BusFare;
public interface BusFareHandler {
/**
* Initializes your available change.
*
*
* create a MaxHeap with a 1,5,10,25,50,100 coin randomly inserted
*/
public void grabChange();
/**
* get the largest ( in this case the 100 cent ) coin from your maxHeap
*
* @return max
*/
public int getMaxFromHeap();
/**
* (1) The param for this method should be the result returned from getMaxFromHeap()
*
* (2) RECURSIVSELY build up the bus fare.
*
*
* (3) Constraint: Bus only accepts 5,25,50,100 cent coins, but you should have other coins in your collection.
*
*
* @param coin
*/
public int payBusFare(int coin);
//week1 test recursive call by selecting next element out of a sorted array instead of calling getMaxFromHeap()
}
BusFareHandlerImpl.java
package BusFare;
import MaxHeap.MaxHeap;
import MaxHeap.MaxHeapImpl;
import java.util.Random;
public class BusFareHandlerImpl implements BusFareHandler {
private MaxHeap changeHeap;
//this method loads the maxheap with random coins - nickel thru silver dollar
public void grabChange() {
changeHeap = new MaxHeapImpl();
int[] coins = new int[] {1, 5, 10, 25, 50, 100};
Random rand = new Random();
for (int coin : coins) {
int randNum = rand.nextInt(2);
for (int i = 0; i < randNum; i++) {
changeHeap.insert(coin);
}
} // TODO Auto-generated method stub
}
//this method uses my MaxHeap instance to get the largest coin
public int getMaxFromHeap()
{
return changeHeap.extractMax();
}
// this method (recursive method) retrieves the next coin
public int payBusFare(int coin)
{
if (coin == 0) {
return 0;
} else if (coin == 100 || coin == 50 || coin == 25 || coin == 5) {
changeHeap.delete(coin);
return coin + payBusFare(getMaxFromHeap() - coin);
} else {
return payBusFare(getMaxFromHeap() - coin);
}
}
}
BusFareTester.java
package BusFare;
public class BusFareTester {
public static void main(String[] args) {
BusFareHandler busFare = new BusFareHandlerImpl();
testBusFareHandler(busFare);
}
private static void testBusFareHandler (BusFareHandler fare) {
fare.grabChange();
int initialCoin = fare.getMaxFromHeap();
System.out.println(" Exact Fare paid: " + fare.payBusFare(initialCoin) + " cents ");
}
}
MaxHeap.java
package MaxHeap;
public interface MaxHeap {
public void insert(int element);
public void display();
public int extractMax();
public void delete(int coin);
}
MaxHeapImpl.java
package MaxHeap;
public class MaxHeapImpl implements MaxHeap{
private int[] Heap;
private int size;
private int maxsize;
public MaxHeapImpl(int maxsize) {
this.maxsize = maxsize;
this.size = size;
Heap = new int[this.maxsize + 1];
Heap[0] = Integer.MAX_VALUE;
}
private int parent(int pos) { return pos/2;}
private int leftChild(int pos) {return pos*2;}
private int rightChild(int pos) {return (pos*2) + 1;}
private void swap(int fpos, int spos)
{
int tmp;
tmp = Heap[fpos];
Heap[fpos] = Heap[spos];
Heap[spos] = tmp;
}
private void downheapify(int pos)
{
if (pos >= (size / 2) && pos <= size)
return;
if (Heap[pos] < Heap[leftChild(pos)] || Heap[pos] < Heap[rightChild(pos)])
{
if (Heap[leftChild(pos)] > Heap[rightChild(pos)])
{
swap(pos, leftChild(pos));
downheapify(leftChild(pos));
}
else
{
swap(pos, rightChild(pos));
downheapify(rightChild(pos));
}
}
}
private void heapifyUp(int pos)
{
int tmp = Heap[pos];
while(pos>0 && tmp > Heap[parent(pos)])
{
Heap[pos] = Heap[parent(pos)];
pos = parent(pos);
}
Heap[pos] = tmp;
}
public void insert(int element)
{
Heap[++size] = element;
int tmp = size;
while (Heap[tmp] > Heap[parent(tmp)])
{
swap(tmp, parent(tmp));
tmp = parent(tmp);
}
}
public void display()
{
for (int i = 1; i <= size / 2; i++) {
System.out.println("Parent: " + Heap[i] + " Left Child: " + Heap[2 * i] + " Right Child: " + Heap[2 * i + 1]);
System.out.println();
}
}
public int extractMax()
{
int max = Heap[1];
Heap[1] = Heap[size--];
Heap[size+1] = 0;
downheapify(1);
return max;
}
public void delete(int coin)
{
}
}
MaxHeapTester.java
package MaxHeap;
public class MaxHeapTester {
public static void main(String[] args) {
// MaxHeap theHeap = new MaxHeapImpl(15);
// runTests(theHeap);
}
public static void runTests(MaxHeap theHeap) {
}
}

Step by step
Solved in 5 steps

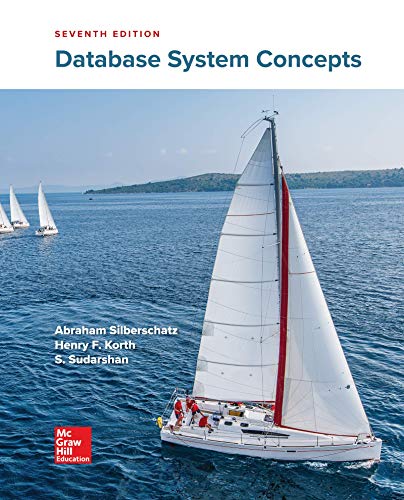
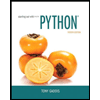
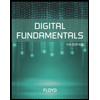
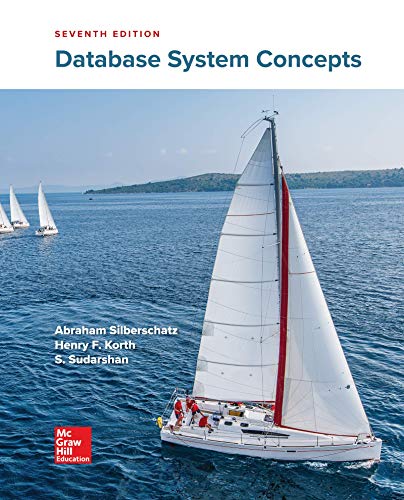
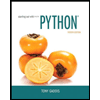
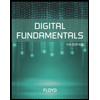
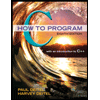
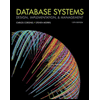
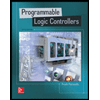