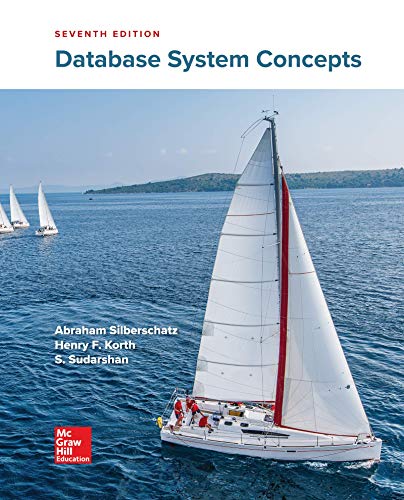
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Please fill in all the code gaps if possible: (java)
public class LinkedList {
privateLinkedListNode head;
**Creates a new instance of LinkedList**
public LinkedList () {
}
public LinkedListNode getHead()
public void setHead (LinkedListNode head)
**Add item at the front of the linked list**
public void insertFront (Object item)
**Remove item at the front of linked list and return the object variable**
public Object removeFront()
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Redesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forward1.Assume some Node class with info link fields . Complete this method in class List that returns a reference to the node containing the data item in the argument findThis, Assume that findThis is in the list public class list { protected Node head ; Protected int size ; Public Node find (char find this) { Node curr = head; while(curr != null) { if(curr.info == findThis) return curr; curr = curr.link; } return -1; } }arrow_forwardLinked List, create your own code. (Do not use the build in function or classes of Java or from the textbook). Create a LinkedList class: Call the class MyLinkedList, (hint) Create a second class called Node.java and use it, remember in the class I put the Node class inside the LinkedList Class, but you should do it outside. This class should haveo Variables you may need for a Node,o (optional) Constructor Your linked list is of an int type. (you may do it as General type as <E>) For this Linked List you need to have the following methods: add, addAfter, remove, size, contain, toString, compare, addInOrder. This is just a suggestion, if you use Generic type, you must modify this Write a main function or Main class to test all the methods,o Create a 2 linked list and test all your methods. (Including the compare)arrow_forward
- Send me your own work not AI generated response or plagiarised response. If I see these things, I'll give multiple downvotes and will definitely report.arrow_forwardGiven the following definition for a LinkedList: // LinkedList.h class LinkedList { public: LinkedList(); // TODO: Implement me void printEveryOther() const; private: struct Node { int data; Node* next; }; Node * head; }; // LinkedList.cpp #include "LinkedList.h" LinkedList::LinkedList() { head = nullptr; } Implement the function printEveryOther, which prints every other data value (i.e. those at the odd indices assuming 0-based indexing).arrow_forwardStarter code for ShoppingList.java import java.util.*;import java.util.LinkedList; public class ShoppingList{ public static void main(String[] args) { Scanner scnr=new Scanner(System.in); LinkedList<ListItem>shoppingList=new LinkedList<ListItem>();//declare LinkedList String item; int i=0,n=0;//declare variables item=scnr.nextLine();//get input from user while(item.equals("-1")!=true)//get inputuntil user not enter -1 { shoppingList.add(new ListItem(item));//add into shoppingList LinkedList n++;//increment n item=scnr.nextLine();//get item from user } for(i=0;i<n;i++) { shoppingList.get(i).printNodeData();//call printNodeData()for each object } }} class ListItem{ String item; //constructor ListItem(String item) { this.item=item; } void printNodeData() { System.out.println(item); }}arrow_forward
- package circularlinkedlist;import java.util.Iterator; public class CircularLinkedList<E> implements Iterable<E> { // Your variablesNode<E> head;Node<E> tail;int size; // BE SURE TO KEEP TRACK OF THE SIZE // implement this constructorpublic CircularLinkedList() {} // I highly recommend using this helper method// Return Node<E> found at the specified index// be sure to handle out of bounds casesprivate Node<E> getNode(int index ) { return null;} // attach a node to the end of the listpublic boolean add(E item) {this.add(size,item);return false; } // Cases to handle// out of bounds// adding to empty list// adding to front// adding to "end"// adding anywhere else// REMEMBER TO INCREMENT THE SIZEpublic void add(int index, E item){ } // remove must handle the following cases// out of bounds// removing the only thing in the list// removing the first thing in the list (need to adjust the last thing in the list to point to the beginning)// removing the last…arrow_forwardWrite the following method in Java: public void reverse() The method will reverse the linked list. Head will point to the last node of the linked list and all the pointers (addresses) will be reversed. The last node (a new head) will point to the second last node and so on. The old head node will become the tail node in the reversed LinkedList. Use the LinkedList class discussed in the video lecture and write the reverse() method in the same class. Test your method in the main method. Following picture depicts the reversal of the LinkedList. NULL Head I V R I ↑ Head > NULLarrow_forwardSimple linkedlist implementation please help (looks like a lot but actually isnt, everything is already set up for you below to make it EVEN easier) help in any part you can be clear Given an interface for LinkedList- Without using the java collections interface (ie do not import java.util.List,LinkedList, Stack, Queue...)- Create an implementation of LinkedList interface provided- For the implementation create a tester to verify the implementation of thatdata structure performs as expected Build Bus Route – Linked List- You’ll be provided a java package containing an interface, data structure,implementation shell, test harness shell below.- Your task is to:o Implement the LinkedList interface (fill out the implementation shell)o Put your implementation through its paces by exercising each of themethods in the test harnesso Create a client (a class with a main) ‘BusClient’ which builds a busroute by performing the following operations on your linked list:o§ Create (insert) 4 stations§…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
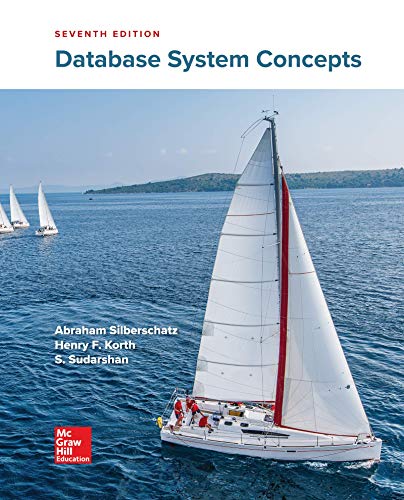
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
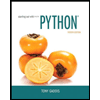
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
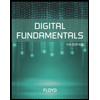
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
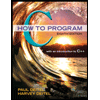
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
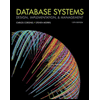
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
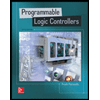
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education