How to I code without using the instanceof (see in bold) in my toString method? Please show work if possible! /** * This is the WordNode class for the Sentence interface. */ public class WordNode implements Sentence { private final String word; private final Sentence rest; /** * This is the constructor for the Sentence class. * @param word is a string format * @param rest is a sentence */ public WordNode(String word, Sentence rest) { this.word = word; this.rest = rest; } /** * This calculates the total number of words in a sentence. * @return the total number of words from teh current */ @Override public int getNumberOfWords() { return 1 + this.rest.getNumberOfWords(); } /** * This method determines the longest word in the sentence. * @return a string of the longest word in teh sentence */ @Override public String longestWord() { if (word.length() >= this.rest.longestWord().length()) { return this.word; } return this.rest.longestWord(); } /** * This method adds a period to the end of a string if there is one. * If no string, there is no period. * @return a period to the string */ @Override public String toString() { if (this.rest.toString().equals("")) { return this.word + "."; } if (rest instanceof PunctuationNode) { return this.word + this.rest.toString(); } return this.word + " " + this.rest.toString(); } /** * This method clones a sentence; duplicates it. * @return a string of a clone sentence */ @Override public Sentence clone() { return new WordNode(this.word, this.rest.clone()); } /** * This method merges two sentences * @param secondSentence to merge with the first * @return a merge of two sentences to create a new one */ @Override public Sentence merge(Sentence secondSentence) { return new WordNode(this.word, this.rest.merge(secondSentence)); } }
How to I code without using the instanceof (see in bold) in my toString method? Please show work if possible!
/**
* This is the WordNode class for the Sentence interface.
*/
public class WordNode implements Sentence {
private final String word;
private final Sentence rest;
/**
* This is the constructor for the Sentence class.
* @param word is a string format
* @param rest is a sentence
*/
public WordNode(String word, Sentence rest) {
this.word = word;
this.rest = rest;
}
/**
* This calculates the total number of words in a sentence.
* @return the total number of words from teh current
*/
@Override
public int getNumberOfWords() {
return 1 + this.rest.getNumberOfWords();
}
/**
* This method determines the longest word in the sentence.
* @return a string of the longest word in teh sentence
*/
@Override
public String longestWord() {
if (word.length() >= this.rest.longestWord().length()) {
return this.word;
}
return this.rest.longestWord();
}
/**
* This method adds a period to the end of a string if there is one.
* If no string, there is no period.
* @return a period to the string
*/
@Override
public String toString() {
if (this.rest.toString().equals("")) {
return this.word + ".";
}
if (rest instanceof PunctuationNode) {
return this.word + this.rest.toString();
}
return this.word + " " + this.rest.toString();
}
/**
* This method clones a sentence; duplicates it.
* @return a string of a clone sentence
*/
@Override
public Sentence clone() {
return new WordNode(this.word, this.rest.clone());
}
/**
* This method merges two sentences
* @param secondSentence to merge with the first
* @return a merge of two sentences to create a new one
*/
@Override
public Sentence merge(Sentence secondSentence) {
return new WordNode(this.word, this.rest.merge(secondSentence));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

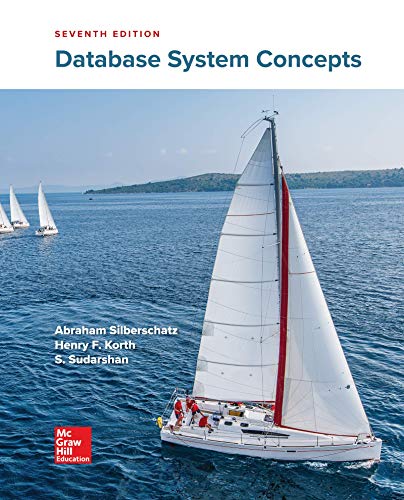
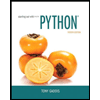
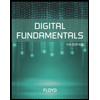
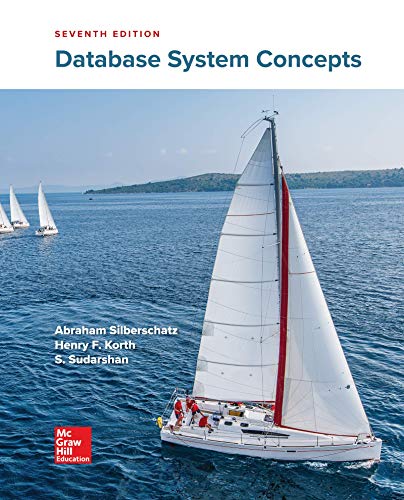
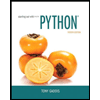
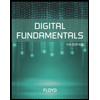
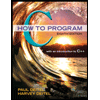
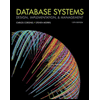
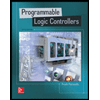