help me run this code import java.util.*; class Main{ static int max_kids; HashMap > sports ; public Main(int max){ max_kids = max; sports = new HashMap<>(); } static class Person{ String name; int age; public Person(String name , int age ) { this.name = name; this.age = age; } } static class Trainer extends Person{ int id; public Trainer(int id , String name , int age ) { super(name , age ); this.id = id; } } static class Kid extends Person{ int id; public Kid(int id , String name , int age ) { super(name , age ); this.id = id; } } static class Section { int section; String course ; Trainer instructor; int size ; HashMap Trainers; public Section(int section , String course , Trainer instructor) { this.section = section; this.course = course; instructor = this.instructor; Kids = new HashMap<>(); this.size = 0; } public void addKid(int id ,Kid s1) { if(Kids.containsKey(id) || size == max_Kids) { System.out.println("Adding student to Section is not succesful"); } else { Kids.put(id ,s1); size++; System.out.println("Adding student to Section is succesful"); } } public void removeStudent(int id ) { if(!Kids.containsKey(id) || size == 0) { System.out.println("Removing student from Section is not succesful"); } else { Kids.remove(id); size--; System.out.println("Removing student from Section is succesful"); } } private void removekids(int i) { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } } public void addSection(String course , Section s) { if(!sports.containsKey(course)) sports.put(course,new ArrayList()); sports.get(course).add(s); } public void DisplaySection() { for(String key : sports.keySet()) { System.out.print("Courses with sections : "); for(Section s : sports.get(key)) { System.out.print(s.section +" "); } System.out.println(); } } public static void main(String args[]) { Main cm = new Main (2); Kid s1 = new Kid(123,"John",15); Kid s2 = new Kid(456 , "Paul" ,16); Kid s3 = new Kid(789 , "Chris" ,15); Kid s4 = new Kid(901 , "Morris" ,17); Trainer t1 = new Trainer(1234 , "Sophie" , 28); Trainer t2 = new Trainer(1000 , "Alena" , 40); Section sec1 = new Section(11,"Football",t1); cm.addSection("M54", sec1); Section sec2 = new Section(22,"Cricket",t2); cm.addSection("M55", sec2); sec1.addKid(123, s1); sec1.addKid(456, s2); sec1.addKid(789, s3); sec1.removekids (123); sec2.addKid(901, s4); cm.DisplaySection(); } }
help me run this code
import java.util.*;
class Main{
static int max_kids;
HashMap<String,ArrayList<Section> > sports ;
public Main(int max){
max_kids = max;
sports = new HashMap<>();
}
static class Person{
String name;
int age;
public Person(String name , int age )
{
this.name = name;
this.age = age;
}
}
static class Trainer extends Person{
int id;
public Trainer(int id , String name , int age )
{
super(name , age );
this.id = id;
}
}
static class Kid extends Person{
int id;
public Kid(int id , String name , int age )
{
super(name , age );
this.id = id;
}
}
static class Section
{
int section;
String course ;
Trainer instructor;
int size ;
HashMap<Integer ,Trainer > Trainers;
public Section(int section , String course , Trainer instructor)
{
this.section = section;
this.course = course;
instructor = this.instructor;
Kids = new HashMap<>();
this.size = 0;
}
public void addKid(int id ,Kid s1)
{
if(Kids.containsKey(id) || size == max_Kids)
{
System.out.println("Adding student to Section is not succesful");
}
else
{
Kids.put(id ,s1);
size++;
System.out.println("Adding student to Section is succesful");
}
}
public void removeStudent(int id )
{
if(!Kids.containsKey(id) || size == 0)
{
System.out.println("Removing student from Section is not succesful");
}
else
{
Kids.remove(id);
size--;
System.out.println("Removing student from Section is succesful");
}
}
private void removekids(int i) {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
}
public void addSection(String course , Section s)
{
if(!sports.containsKey(course))
sports.put(course,new ArrayList<Section>());
sports.get(course).add(s);
}
public void DisplaySection()
{
for(String key : sports.keySet())
{
System.out.print("Courses with sections : ");
for(Section s : sports.get(key))
{
System.out.print(s.section +" ");
}
System.out.println();
}
}
public static void main(String args[])
{
Main cm = new Main (2);
Kid s1 = new Kid(123,"John",15);
Kid s2 = new Kid(456 , "Paul" ,16);
Kid s3 = new Kid(789 , "Chris" ,15);
Kid s4 = new Kid(901 , "Morris" ,17);
Trainer t1 = new Trainer(1234 , "Sophie" , 28);
Trainer t2 = new Trainer(1000 , "Alena" , 40);
Section sec1 = new Section(11,"Football",t1);
cm.addSection("M54", sec1);
Section sec2 = new Section(22,"Cricket",t2);
cm.addSection("M55", sec2);
sec1.addKid(123, s1);
sec1.addKid(456, s2);
sec1.addKid(789, s3);
sec1.removekids (123);
sec2.addKid(901, s4);
cm.DisplaySection();
}
}
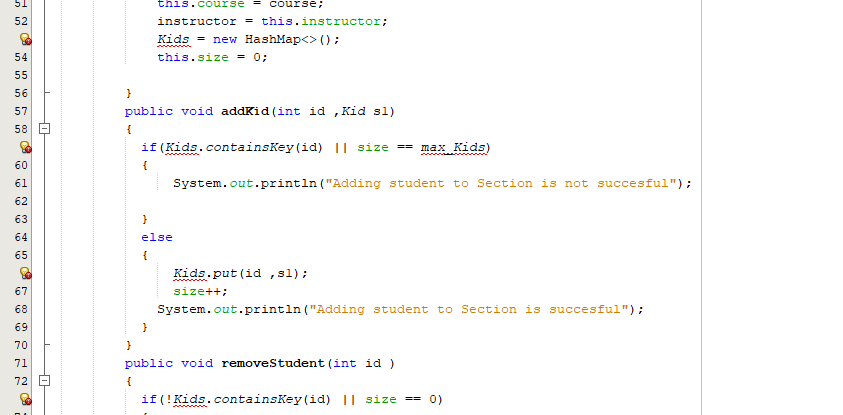

Step by step
Solved in 3 steps

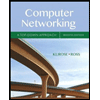
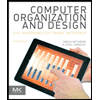
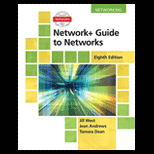
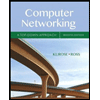
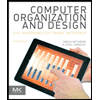
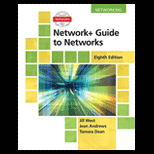
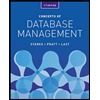
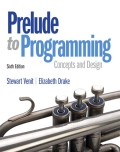
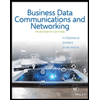