How would I create this in java using this skeleton code? Implement a simple weekly payroll program. The input will consist of the employee’s full name, employee’s ID (String), the number of hours worked per week (double), the hourly rate (double), the income tax is 6%. Your program should calculate the total gross pay, and the net pay and display a paycheck like: Employee’s name: John Smith Employee’s number: js1200 Hourly rate of pay: 10.50 Hours worked: 36.00 Total Gross Pay: $378.00 Deductions Tax (6 %): $22.68 Net Pay: 355.32 Dollars Your program must include the class Employee whose private attributes are: - fullName: String - employeeNumber: String - payRate: double - hoursWorked: double (Don’t add any variables to the class Employee) In addition to that, equip the class Employee with these methods: - Employee (String fullName, String employeeNumber, double payRate, double hoursWorked) This constructor assigns the parametres fullName, employeeNumber, payrate, hoursWorked to the data members. - For each of the private data members above, add the Setter and the Getter - Override the toString() method to return the String of the form: [Employee Number/Full Name, x Hours @ y per hour] where x is thenumber of hours worked and y is the pay rate. As an example, [js1200/John Smith, 36 Hours @ 10.5 per hour] - double netPay (), the private method that returns the net pay of the employee. The net pay is calculated by deducting 6% from the gross pay. See sample check above. - void printCheck (), the method that prints the paycheck of the employee as shown above. The printCheck method calls the private netPay method to get the net pay of the employee. So do not recalculate net pay in this printCheck method. public class HW { public static void main(String[] args) { String fullName = "Erika T. Jones"; String employeeNumber = "ej789"; double payRate = 100.0, hoursWorked = 1.0; Employee e; e = new Employee(fullName, employeeNumber, payRate, hoursWorked); System.out.println(e); // To Test your toString method e.printCheck(); // This prints the check of Erika T. Jones Company company = new Company(); company.hire ( new Employee ("Saeed Happy", "sh895" , 2 , 200) ); company.hire (e); Company.printCompanyInfo(); company.hire( new Employee("Enrico Torres" , "et897" , 3 , 150) ); //Make sure that each employee of company has a unique employeeNumber company.printCheck("ab784"); company.deleteEmployeesBySalary(256.36); company.reverseEmployees(); System.out.println( company.SearchByName("WaLiD WiLLiAms") ); Company.printEmployees(); System.out.println("Bye!"); } } //____________________________ class Employee { //Add the private attributes and the methods as mentioned above... } //____________________________ class Company { private ArrayList employeeList; private static String companyName; private static String companyTaxId; //Add static Setters and Getters for companyName and companyTaxId //No need to add a Setter and Getter for employeeList public Company() { employeeList = new ArrayList<>(); companyName = "People's Place"; companyTaxId = "v1rtua7C0mpan1"; } public boolean hire ( Employee employee ) { //Add empoyee to employeeList //Note well that we can't add an employee whose employeeNumber already //assigned to another employee. In that case, this method returns false. //This method returns true otherwise } public static void printCompanyInfo() { //This method prints the compay name, its tax id and the current number of employees //You may choose to print that any way you like! } public void printEmployees() { //This methods prints all employees (One employee per line) //Note that you already have toString in Employee } public int countEmployees( double maxSalary ) { //This method returns the number of employees paid less than maxSalary } public boolean SearchByName (String fullName ) { //This method returns true if fullName exists as an employee. //It returns false otherwise //this is a not a case sensitive search. } public void reverseEmployees () { //This method reverses the order in which the employees were added to //the list. The last employee is swapped with the first employee, the second last with the second and so on.. } public void deleteEmployeesBySalary (double targetSalary ) { //This method deletes all employees who are paid targetSalary as a net //salary } public void printCheck ( String employeeNumber) { //This method prints the check of the employee whose employee number is //employeeNumber. It prints NO SUCH EMPLOYEE EXISTS if employeeNumber is //not a registered employee number. } }//end of class Company
How would I create this in java using this skeleton code? Implement a simple weekly payroll program. The input will consist of the employee’s full name, employee’s ID (String), the number of hours worked per week (double), the hourly rate (double), the income tax is 6%. Your program should calculate the total gross pay, and the net pay and display a paycheck like: Employee’s name: John Smith Employee’s number: js1200 Hourly rate of pay: 10.50 Hours worked: 36.00 Total Gross Pay: $378.00 Deductions Tax (6 %): $22.68 Net Pay: 355.32 Dollars Your program must include the class Employee whose private attributes are: - fullName: String - employeeNumber: String - payRate: double - hoursWorked: double (Don’t add any variables to the class Employee) In addition to that, equip the class Employee with these methods: - Employee (String fullName, String employeeNumber, double payRate, double hoursWorked) This constructor assigns the parametres fullName, employeeNumber, payrate, hoursWorked to the data members. - For each of the private data members above, add the Setter and the Getter - Override the toString() method to return the String of the form: [Employee Number/Full Name, x Hours @ y per hour] where x is thenumber of hours worked and y is the pay rate. As an example, [js1200/John Smith, 36 Hours @ 10.5 per hour] - double netPay (), the private method that returns the net pay of the employee. The net pay is calculated by deducting 6% from the gross pay. See sample check above. - void printCheck (), the method that prints the paycheck of the employee as shown above. The printCheck method calls the private netPay method to get the net pay of the employee. So do not recalculate net pay in this printCheck method. public class HW { public static void main(String[] args) { String fullName = "Erika T. Jones"; String employeeNumber = "ej789"; double payRate = 100.0, hoursWorked = 1.0; Employee e; e = new Employee(fullName, employeeNumber, payRate, hoursWorked); System.out.println(e); // To Test your toString method e.printCheck(); // This prints the check of Erika T. Jones Company company = new Company(); company.hire ( new Employee ("Saeed Happy", "sh895" , 2 , 200) ); company.hire (e); Company.printCompanyInfo(); company.hire( new Employee("Enrico Torres" , "et897" , 3 , 150) ); //Make sure that each employee of company has a unique employeeNumber company.printCheck("ab784"); company.deleteEmployeesBySalary(256.36); company.reverseEmployees(); System.out.println( company.SearchByName("WaLiD WiLLiAms") ); Company.printEmployees(); System.out.println("Bye!"); } } //____________________________ class Employee { //Add the private attributes and the methods as mentioned above... } //____________________________ class Company { private ArrayList employeeList; private static String companyName; private static String companyTaxId; //Add static Setters and Getters for companyName and companyTaxId //No need to add a Setter and Getter for employeeList public Company() { employeeList = new ArrayList<>(); companyName = "People's Place"; companyTaxId = "v1rtua7C0mpan1"; } public boolean hire ( Employee employee ) { //Add empoyee to employeeList //Note well that we can't add an employee whose employeeNumber already //assigned to another employee. In that case, this method returns false. //This method returns true otherwise } public static void printCompanyInfo() { //This method prints the compay name, its tax id and the current number of employees //You may choose to print that any way you like! } public void printEmployees() { //This methods prints all employees (One employee per line) //Note that you already have toString in Employee } public int countEmployees( double maxSalary ) { //This method returns the number of employees paid less than maxSalary } public boolean SearchByName (String fullName ) { //This method returns true if fullName exists as an employee. //It returns false otherwise //this is a not a case sensitive search. } public void reverseEmployees () { //This method reverses the order in which the employees were added to //the list. The last employee is swapped with the first employee, the second last with the second and so on.. } public void deleteEmployeesBySalary (double targetSalary ) { //This method deletes all employees who are paid targetSalary as a net //salary } public void printCheck ( String employeeNumber) { //This method prints the check of the employee whose employee number is //employeeNumber. It prints NO SUCH EMPLOYEE EXISTS if employeeNumber is //not a registered employee number. } }//end of class Company
Chapter2: Using Data
Section: Chapter Questions
Problem 19RQ
Related questions
Question
How would I create this in java using this skeleton code?
Implement a simple weekly payroll program. The input will consist of the employee’s full name, employee’s ID (String), the number of hours worked per week (double), the hourly rate (double), the income tax is 6%. Your program should calculate the total gross pay, and the net pay and display a paycheck like:
Employee’s name: John Smith
Employee’s number: js1200
Hourly rate of pay: 10.50
Hours worked: 36.00
Total Gross Pay: $378.00
Employee’s number: js1200
Hourly rate of pay: 10.50
Hours worked: 36.00
Total Gross Pay: $378.00
Deductions
Tax (6 %): $22.68
Net Pay: 355.32 Dollars
Tax (6 %): $22.68
Net Pay: 355.32 Dollars
Your program must include the class Employee whose private attributes
are:
- fullName: String
- employeeNumber: String
- payRate: double
- hoursWorked: double
are:
- fullName: String
- employeeNumber: String
- payRate: double
- hoursWorked: double
(Don’t add any variables to the class Employee)
In addition to that, equip the class Employee with these methods:
- Employee (String fullName, String employeeNumber, double payRate,
double hoursWorked)
This constructor assigns the parametres fullName, employeeNumber,
payrate, hoursWorked to the data members.
- For each of the private data members above, add the Setter and the
Getter
- Override the toString() method to return the String of the form:
[Employee Number/Full Name, x Hours @ y per hour] where x is thenumber of hours worked and y is the pay rate.
In addition to that, equip the class Employee with these methods:
- Employee (String fullName, String employeeNumber, double payRate,
double hoursWorked)
This constructor assigns the parametres fullName, employeeNumber,
payrate, hoursWorked to the data members.
- For each of the private data members above, add the Setter and the
Getter
- Override the toString() method to return the String of the form:
[Employee Number/Full Name, x Hours @ y per hour] where x is thenumber of hours worked and y is the pay rate.
As an example,
[js1200/John Smith, 36 Hours @ 10.5 per hour]
[js1200/John Smith, 36 Hours @ 10.5 per hour]
- double netPay (), the private method that returns the net pay of the employee. The net pay is calculated by deducting 6% from the gross pay. See sample check above.
- void printCheck (), the method that prints the paycheck of the employee as shown above. The printCheck method calls the private netPay method to get the net pay of the employee. So do not recalculate net pay in this printCheck method.
- void printCheck (), the method that prints the paycheck of the employee as shown above. The printCheck method calls the private netPay method to get the net pay of the employee. So do not recalculate net pay in this printCheck method.
public class HW {
public static void main(String[] args) {
String fullName = "Erika T. Jones";
String employeeNumber = "ej789";
double payRate = 100.0, hoursWorked = 1.0;
Employee e;
e = new Employee(fullName, employeeNumber, payRate, hoursWorked);
System.out.println(e); // To Test your toString method
e.printCheck(); // This prints the check of Erika T. Jones
Company company = new Company();
company.hire ( new Employee ("Saeed Happy", "sh895" , 2 , 200) );
company.hire (e);
public static void main(String[] args) {
String fullName = "Erika T. Jones";
String employeeNumber = "ej789";
double payRate = 100.0, hoursWorked = 1.0;
Employee e;
e = new Employee(fullName, employeeNumber, payRate, hoursWorked);
System.out.println(e); // To Test your toString method
e.printCheck(); // This prints the check of Erika T. Jones
Company company = new Company();
company.hire ( new Employee ("Saeed Happy", "sh895" , 2 , 200) );
company.hire (e);
Company.printCompanyInfo();
company.hire( new Employee("Enrico Torres" , "et897" , 3 , 150) );
//Make sure that each employee of company has a unique employeeNumber
company.printCheck("ab784");
company.deleteEmployeesBySalary(256.36);
company.reverseEmployees();
System.out.println( company.SearchByName("WaLiD WiLLiAms") );
Company.printEmployees();
System.out.println("Bye!");
}
}
//____________________________
class Employee {
//Add the private attributes and the methods as mentioned above...
}
//____________________________
class Company {
company.hire( new Employee("Enrico Torres" , "et897" , 3 , 150) );
//Make sure that each employee of company has a unique employeeNumber
company.printCheck("ab784");
company.deleteEmployeesBySalary(256.36);
company.reverseEmployees();
System.out.println( company.SearchByName("WaLiD WiLLiAms") );
Company.printEmployees();
System.out.println("Bye!");
}
}
//____________________________
class Employee {
//Add the private attributes and the methods as mentioned above...
}
//____________________________
class Company {
private ArrayList<Employee> employeeList;
private static String companyName;
private static String companyTaxId;
//Add static Setters and Getters for companyName and companyTaxId
//No need to add a Setter and Getter for employeeList
private static String companyName;
private static String companyTaxId;
//Add static Setters and Getters for companyName and companyTaxId
//No need to add a Setter and Getter for employeeList
public Company() {
employeeList = new ArrayList<>();
companyName = "People's Place";
companyTaxId = "v1rtua7C0mpan1";
}
public boolean hire ( Employee employee ) {
//Add empoyee to employeeList
//Note well that we can't add an employee whose employeeNumber already
employeeList = new ArrayList<>();
companyName = "People's Place";
companyTaxId = "v1rtua7C0mpan1";
}
public boolean hire ( Employee employee ) {
//Add empoyee to employeeList
//Note well that we can't add an employee whose employeeNumber already
//assigned to another employee. In that case, this method returns false.
//This method returns true otherwise
}
public static void printCompanyInfo() {
//This method prints the compay name, its tax id and the current number of employees
//You may choose to print that any way you like!
}
public void printEmployees() {
//This methods prints all employees (One employee per line)
//Note that you already have toString in Employee
}
public int countEmployees( double maxSalary ) {
//This method returns the number of employees paid less than maxSalary
}
//This method returns true otherwise
}
public static void printCompanyInfo() {
//This method prints the compay name, its tax id and the current number of employees
//You may choose to print that any way you like!
}
public void printEmployees() {
//This methods prints all employees (One employee per line)
//Note that you already have toString in Employee
}
public int countEmployees( double maxSalary ) {
//This method returns the number of employees paid less than maxSalary
}
public boolean SearchByName (String fullName ) {
//This method returns true if fullName exists as an employee.
//It returns false otherwise
//this is a not a case sensitive search.
}
public void reverseEmployees () {
//This method reverses the order in which the employees were added to //the list.
The last employee is swapped with the first employee, the second last with the second
and so on..
}
public void deleteEmployeesBySalary (double targetSalary ) {
//This method deletes all employees who are paid targetSalary as a net //salary
}
public void printCheck ( String employeeNumber) {
//This method prints the check of the employee whose employee number is
//employeeNumber. It prints NO SUCH EMPLOYEE EXISTS if employeeNumber is //not
a registered employee number.
}
}//end of class Company
//This method returns true if fullName exists as an employee.
//It returns false otherwise
//this is a not a case sensitive search.
}
public void reverseEmployees () {
//This method reverses the order in which the employees were added to //the list.
The last employee is swapped with the first employee, the second last with the second
and so on..
}
public void deleteEmployeesBySalary (double targetSalary ) {
//This method deletes all employees who are paid targetSalary as a net //salary
}
public void printCheck ( String employeeNumber) {
//This method prints the check of the employee whose employee number is
//employeeNumber. It prints NO SUCH EMPLOYEE EXISTS if employeeNumber is //not
a registered employee number.
}
}//end of class Company
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
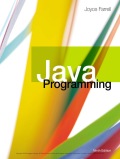
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
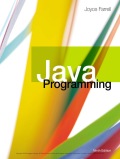
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT