I am trying to 1) double a number that is given by a user. 2) Take that number and divided it by 10 until it no longer is divisable by 10. 3)Push each individual modula onto a stack so that the doubled number saves into the Stack from right to left. eg.(User enters 123...console should double the value to 246, and then place 6**4**2** into a stack. 6 being the topmost number) The roadblock I am getting to is that when I try to push the numbers on the stack with a while loop, it seems like push only remembers the last number that was pushed.
I am trying to 1) double a number that is given by a user. 2) Take that number and divided it by 10 until it no longer is divisable by 10. 3)Push each individual modula onto a stack so that the doubled number saves into the Stack from right to left. eg.(User enters 123...console should double the value to 246, and then place 6**4**2** into a stack. 6 being the topmost number) The roadblock I am getting to is that when I try to push the numbers on the stack with a while loop, it seems like push only remembers the last number that was pushed.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
I am trying to 1) double a number that is given by a user.
2) Take that number and divided it by 10 until it no longer is divisable by 10.
3)Push each individual modula onto a stack so that the doubled number saves into the Stack from right to left.
eg.(User enters 123...console should double the value to 246, and then place 6**4**2** into a stack. 6 being the topmost number)
The roadblock I am getting to is that when I try to push the numbers on the stack with a while loop, it seems like push only remembers the last number that was pushed.
![1 package numbers;
2
3 import java.util.Scanner;
4
5 public class NumberReverse {
6° public static void main(String[]args) {
StackArray stackArray= new StackArray(20);
Scanner console=new Scanner(System.in);
System.out.println("Enter your 8-15 digit number");
long number=0;
number = console.nextLong();
//2468
long doubleNumber=number*2;
System.out.println(doubleNumber + " is your doubled number");
int five = 6;//2468
while(doubleNumber/10 > 0) //while the doubled # is greater than 0 when divided by 10
{
//doubleNumber = doubleNumber / 10;
System.out.println(((int)doubleNumber%10));//stackArray.push((int)doubleNumber%10); /
doubleNumber = (int)(doubleNumber / 10);
stackArray.push((int)doubleNumber);
//System.out.println(doubleNumber);
five--;
//stackArray.push(0);
}
System.out.println(doubleNumber+ " is in the array");
7
8
9.
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28 }
29 }
30
Console X
Problems D Debug Shell
<terminated> NumberReverse [Java Application] C:\Users\desir\.p2\pool\plugins\org.eclipse.justj.openjdk.hotspotjre.full.win32.x86_€
1234
2468 is your doubled number
8
2 is in the array
6 4](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe47c4bd6-568c-4770-a86e-93574933d968%2F1673f0c8-4b90-4a94-ba22-c736b743a7bb%2Fxtqhe0f_processed.png&w=3840&q=75)
Transcribed Image Text:1 package numbers;
2
3 import java.util.Scanner;
4
5 public class NumberReverse {
6° public static void main(String[]args) {
StackArray stackArray= new StackArray(20);
Scanner console=new Scanner(System.in);
System.out.println("Enter your 8-15 digit number");
long number=0;
number = console.nextLong();
//2468
long doubleNumber=number*2;
System.out.println(doubleNumber + " is your doubled number");
int five = 6;//2468
while(doubleNumber/10 > 0) //while the doubled # is greater than 0 when divided by 10
{
//doubleNumber = doubleNumber / 10;
System.out.println(((int)doubleNumber%10));//stackArray.push((int)doubleNumber%10); /
doubleNumber = (int)(doubleNumber / 10);
stackArray.push((int)doubleNumber);
//System.out.println(doubleNumber);
five--;
//stackArray.push(0);
}
System.out.println(doubleNumber+ " is in the array");
7
8
9.
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28 }
29 }
30
Console X
Problems D Debug Shell
<terminated> NumberReverse [Java Application] C:\Users\desir\.p2\pool\plugins\org.eclipse.justj.openjdk.hotspotjre.full.win32.x86_€
1234
2468 is your doubled number
8
2 is in the array
6 4
![1 package numbers;
2
3 public class StackArray
4 {
private int [] elements;
private int top;
private int size;
7
8.
9.
100
public StackArray (int size)
{
elements = new int[size];
top = -1;
this.size = size;
11
12
13
14
15
}
16
public boolean empty()
{
return top
}
170
18
19
== -1:
20
21
public boolean full()
{
return top == size - 1;
}
220
23
24
25
26
public boolean push(int el)
{
if (full())
return false;
270
28
29
30
31
else
{
top++;
elements[top] = el;
32
33
34
35
return true;
36
}
37
38
390
40
41
42
public int pop()
{
int el = elements[top];|
top--;
return el;
43
44
}
45
46
47 }
48](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe47c4bd6-568c-4770-a86e-93574933d968%2F1673f0c8-4b90-4a94-ba22-c736b743a7bb%2Fhk5a7pw_processed.png&w=3840&q=75)
Transcribed Image Text:1 package numbers;
2
3 public class StackArray
4 {
private int [] elements;
private int top;
private int size;
7
8.
9.
100
public StackArray (int size)
{
elements = new int[size];
top = -1;
this.size = size;
11
12
13
14
15
}
16
public boolean empty()
{
return top
}
170
18
19
== -1:
20
21
public boolean full()
{
return top == size - 1;
}
220
23
24
25
26
public boolean push(int el)
{
if (full())
return false;
270
28
29
30
31
else
{
top++;
elements[top] = el;
32
33
34
35
return true;
36
}
37
38
390
40
41
42
public int pop()
{
int el = elements[top];|
top--;
return el;
43
44
}
45
46
47 }
48
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

Recommended textbooks for you
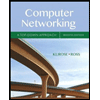
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
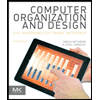
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
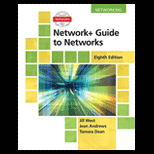
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
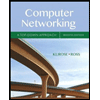
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
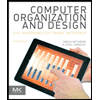
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
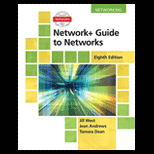
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
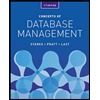
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
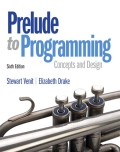
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
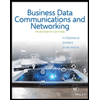
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY