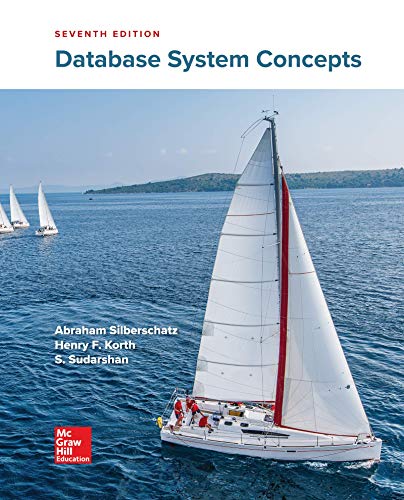
Suppose that you want to create the following program:
void copyStack(std::stack<int> &srcStack, std::stack<int> &dstStack);
This operation copies the elements of srcStack in the same order onto dstStack. Consider the following statements:
Int main() {
Std::stack<int> stack1;
Std::stack<int> stack2;
…
copyStack(stack1, stack2);
The function copyStack(stack1, stack2); copies the elements of stack1 onto stack2 in the same order. That is, the top element of stack1 is the top element of stack2, and so on. The old contents of stack2 are destroyed and stack1 is unchanged. Note that std::stack<int> is a C++ standard library stack object type.
Complete the following program
void copyStack(std::stack<int> &srcStack, std::stack<int> &dstStack)
{
// check if srcStack is empty -> error message is printed and return
// remove all the elements of dstStack
// create a temporary stack tempStack for temporary usage!
// move all the elements from srcStack to tempStack and to dstStack
// move all the elements from tempStack tosrcStack to restore
}

Step by stepSolved in 2 steps

- in c++arrow_forwardLook at this stack frame: Fill in the data types for the following procedure: SomeSub PROC, LOCAL val1: , LOCAL val2: , LOCAL val3: , LOCAL val4:arrow_forward54. What is the difference between the top and pop operations of a stack? Group of answer choices The top operation removes the top item of the stack. The pop operation returns a reference to the top item on the stack and remove it. The top operation removes the top item of the stack. The pop operation returns a reference to the top item on the stack without removing it. The pop operation removes the top item of the stack. The top operation returns a reference to the top item on the stack without removing it. The pop and top operations are doing the same thing which removes the top item of the stack. The pop operation removes the top item of the stack. The top operation returns a reference to the top item on the stack and remove it.arrow_forward
- Consider the following pseudocode: declare a stack of characterswhile ( there are more characters in the word to read ){read a characterpush the character on the stack}while ( the stack is not empty ){write the stack's top character to the screenpop a character off the stack}Call the output written to the screen for the input "carpets" out1. On the other hand;If the characters 'D', 'C', 'B', 'A' are placed in a queue (in that order), and then removed one at a time, call the output when they are removed out2? Correct answer for out1 + out2 is;A. carpets + DCBAB. carpets + ABCDC. steprac + DCBAD. steprac + ABCDarrow_forwardQuestion 11 Consider the following statements: (3) stackType stack(50); int num; Suppose that the input is: 31 47 86 39 62 71 15 63 Show what is output by the following segment of code: cin >> num; cin >> num; cout <« endl; while (cin) cout << "Stack Elements: "; { if (!stack.isFullStack ()) { if (num % 2 == 0 || num % 3 == 0) stack.push (num); else if (!stack.isEmptyStack ()) { while (!stack.isEmptyStack ()) { cout <« " " <« stack.top(); stack.pop () ; } cout « endl; cout « stack.top () <« " "; stack.pop (); } else stack.push (num + 3); 62 33 Stack Elements: 15 60 56 34 35 60 Stack Elements: 23 45 67 78 34 62 Stack Elements: 63 15 39 86 None of the abovearrow_forward1. A company wants to evaluate employee records in order to lay off some workers on the basis of service time (the most recently hired employees are laid off first). Only the employee ids are stored in stack. Write a program using stack and implement the following functions: Main program should ask the appropriate option from the user, until user selects an option for exiting the program. Enter_company (emp_id)- the accepted employee id(integer) is pushed into stack. Exit_company ()- The recently joined employee will be laid off from the company Show employee ()- display all the employees working in the company. count()- displays the number of employees working in the company. i) ii) iii) iv)arrow_forward
- Source Booksh... m HBO Max AAP CS A Java Cours... Assume you have a stack, called myStack, implemented with single-linked nodes, that looks like this: 573 -> 408 -> 142 -> 886 'top' is a reference variable that points to the node containing 573, and 'count' is an internal int that holds the count of nodes (currently 4). The following code is ran: Integer firstPop = myStack.pop(); Integer secondPop = myStack.pop(); myStack.push(firstPop); myStack.push(760); myStack.push(secondPop); myStack.push(738); Select all of the following that are true at the end of this code. The variable secondPop refers to 142. O The element 760 is contained by the third node in this stack (where 'top' is considered the first node). O The count is currently equal to 6. The variable first Pop refers to 573. O The Integer held by firstPop is now the element contained by the node referenced through the top pointer. 886 is the only Integer that didn't change position in the LinkedStack. O The Integer held by second…arrow_forwardQ2: Stack stores elements in an ordered list and allows insertions and deletions at one end.The elements in this stack are stored in an array. If the array is full, the bottom item is dropped from the stack. In practice, this would be equivalent to overwriting that entry in the array. And if top method is called then it should return the element that was entered recently Please do it in C++ and use stack class(header file), not premade functionarrow_forward5. fast please Convert the following infix expression into its prefix and postfix equivalents: a / b - (c + d * e) / f * g For any postfix, show the final transformed expression using the stack method for transformation as explained in class showing all the relevant steps. For the prefix, show any needed reversion and the final transformed expression using the stack method for transformation as explained in class showing all the relevant steps. Draw and sequence your diagrams clearlyarrow_forward
- stacks and queues. program must be able to handle all test cases without causing an exception Note that this problem does not require recursion to solve (though you can use that if you wish).arrow_forwardjavaarrow_forwardConsider the following statements: stackType<int> stack; int x; Suppose that the input is: 14 53 34 32 13 5 -999 Show what is output by the following segment of code: cin >> x; while (x != -999) { if (x % 2 == 0) { if (!stack.isFullStack()) stack.push(x); } else cout << "x = " << x << endl; cin >> x; } cout << "Stack Elements: "; while (!stack.isEmptyStack()) { cout << " " << stack.top(); stack.pop(); } cout << endl;arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
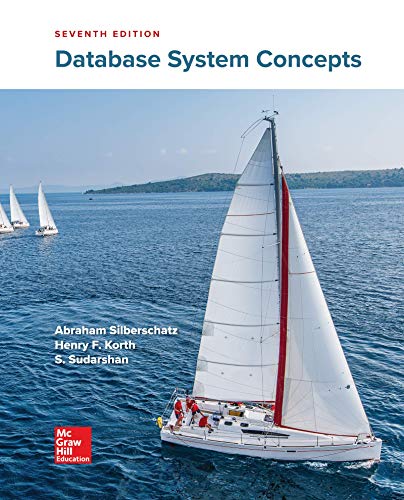
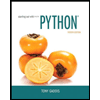
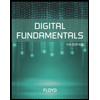
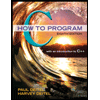
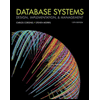
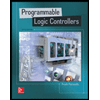