I am writing a stack code, where there are two stacks that reads an array of 40 numbers and puts them in ascending and descending order. I put my code on the bottom. The requirements of the code was to write the stack functions using arrays, but whenever i run the code it does not work. However, if use stack in built function the code works. Help me please. PS: I Attached the code requirements. struct Stack1 { int *arr; int NextIndex; int capacity; Stack1() { capacity = 20; arr = new int[capacity]; NextIndex = 0; } int size () { return NextIndex; } bool isEmpty() { if (NextIndex == 0) { return true; } else return false; } void push(int ele) { if (NextIndex == capacity) { int *newArr = new int[2*capacity]; for(int i = 0; i < capacity; i++) { newArr[i] = arr[i]; } delete []arr; arr = newArr; capacity = 2*capacity; } arr[NextIndex] = ele; NextIndex++; } void pop() { if (isEmpty()) { cout << "The stack is in underflow"<< endl; return; } NextIndex--; } int top() { if (isEmpty()) { cout << "The stack is in underflow"<< endl; return -1; } return arr[NextIndex - 1]; } void printStack() { if (isEmpty()) return; else { for (int i = 0; i < capacity; i ++) { cout << arr[i]; } } } }; struct Stack2 { int *arr; int NextIndex; int capacity; //using dynamic array here Stack2() { capacity = 20; arr = new int[capacity];// if the user does not define the size, the code will give a size of 4 automatically NextIndex = 0; } int size () { return NextIndex; } bool isEmpty() { if (NextIndex == 0) { return true; } else return false; } void push(int ele) { if (NextIndex == capacity) { int *newArr = new int[2*capacity]; for(int i = 0; i < capacity; i++) { newArr[i] = arr[i]; } delete []arr; arr = newArr; capacity = 2*capacity; } arr[NextIndex] = ele; NextIndex++; } void pop() { if (isEmpty()) { cout << "The stack is in underflow"<< endl; return; } NextIndex--; } int top() { if (isEmpty()) { cout << "The stack is in underflow"<< endl; return -1; } return arr[NextIndex - 1]; } }; void printsortedArray() { Stack1 s1; Stack2 s2; if(s1.isEmpty()) { cout << "\n Sorted values in ascending order:"; while (!s2.isEmpty()) { cout << s2.top() << " "; s1.push(s2.top()); s2.pop(); } cout << "\n Sorted values in descending order: "; while (!s1.isEmpty()) { cout << s1.top() << " "; s1.pop(); } } else { cout << "\n Sorted values in descending order: "; while (!s1.isEmpty()) { cout << s1.top()<< " "; s2.push(s1.top()); s1.pop(); } cout << "\n Sorted values in ascending order: "; while (!s2.isEmpty()) { cout << s2.top() << " "; s2.pop(); } } } void s1Add(int n) { Stack1 s1; Stack2 s2; cout << "\n Content of working stack: "; int flag = 0; while (!s2.isEmpty()) { int curr = s2.top(); cout << curr << " "; if (curr < n || flag == 1) s1.push(curr); else { flag = 1; s1.push(n); s1.push(curr); } s2.pop(); } if (flag == 0) s1.push(n); } void s2Add(int n) { Stack1 s1; Stack2 s2; cout << "\n Content of the non-working stack : "; int flag = 0; while (!s1.isEmpty()) { int curr = s1.top(); cout << curr << " "; if (curr > n || flag == 1) s2.push(curr); else { flag = 1; s2.push(n); s2.push(curr); } s1.pop(); } if (flag == 0) s2.push(n); } int main () { int A[40]; int i; Stack1 s1; Stack2 s2; ifstream numbers ("Data_FIle.txt"); for (i = 0; i < 40; i++) { numbers >> A[i]; } for (int i = 0; i < 40; i++) { int ele = A[i]; if (i == 0) s1.push(ele); else if (s2.isEmpty()) s2Add(ele); else s1Add(ele); } printsortedArray(); }
I am writing a stack code, where there are two stacks that reads an array of 40 numbers and puts them in ascending and descending order. I put my code on the bottom. The requirements of the code was to write the stack functions using arrays, but whenever i run the code it does not work. However, if use stack in built function the code works. Help me please.
PS: I Attached the code requirements.
struct Stack1
{
int *arr;
int NextIndex;
int capacity;
Stack1()
{
capacity = 20;
arr = new int[capacity];
NextIndex = 0;
}
int size ()
{
return NextIndex;
}
bool isEmpty()
{
if (NextIndex == 0)
{
return true;
}
else
return false;
}
void push(int ele)
{
if (NextIndex == capacity)
{
int *newArr = new int[2*capacity];
for(int i = 0; i < capacity; i++)
{
newArr[i] = arr[i];
}
delete []arr;
arr = newArr;
capacity = 2*capacity;
}
arr[NextIndex] = ele;
NextIndex++;
}
void pop()
{
if (isEmpty())
{
cout << "The stack is in underflow"<< endl;
return;
}
NextIndex--;
}
int top()
{
if (isEmpty())
{
cout << "The stack is in underflow"<< endl;
return -1;
}
return arr[NextIndex - 1];
}
void printStack()
{
if (isEmpty())
return;
else
{
for (int i = 0; i < capacity; i ++)
{
cout << arr[i];
}
}
}
};
struct Stack2
{
int *arr;
int NextIndex;
int capacity;
//using dynamic array here
Stack2()
{
capacity = 20;
arr = new int[capacity];// if the user does not define the size, the code will give a size of 4 automatically
NextIndex = 0;
}
int size ()
{
return NextIndex;
}
bool isEmpty()
{
if (NextIndex == 0)
{
return true;
}
else
return false;
}
void push(int ele)
{
if (NextIndex == capacity)
{
int *newArr = new int[2*capacity];
for(int i = 0; i < capacity; i++)
{
newArr[i] = arr[i];
}
delete []arr;
arr = newArr;
capacity = 2*capacity;
}
arr[NextIndex] = ele;
NextIndex++;
}
void pop()
{
if (isEmpty())
{
cout << "The stack is in underflow"<< endl;
return;
}
NextIndex--;
}
int top()
{
if (isEmpty())
{
cout << "The stack is in underflow"<< endl;
return -1;
}
return arr[NextIndex - 1];
}
};
void printsortedArray()
{
Stack1 s1;
Stack2 s2;
if(s1.isEmpty())
{
cout << "\n Sorted values in ascending order:";
while (!s2.isEmpty())
{
cout << s2.top() << " ";
s1.push(s2.top());
s2.pop();
}
cout << "\n Sorted values in descending order: ";
while (!s1.isEmpty())
{
cout << s1.top() << " ";
s1.pop();
}
}
else
{
cout << "\n Sorted values in descending order: ";
while (!s1.isEmpty())
{
cout << s1.top()<< " ";
s2.push(s1.top());
s1.pop();
}
cout << "\n Sorted values in ascending order: ";
while (!s2.isEmpty())
{
cout << s2.top() << " ";
s2.pop();
}
}
}
void s1Add(int n)
{
Stack1 s1;
Stack2 s2;
cout << "\n Content of working stack: ";
int flag = 0;
while (!s2.isEmpty())
{
int curr = s2.top();
cout << curr << " ";
if (curr < n || flag == 1)
s1.push(curr);
else
{
flag = 1;
s1.push(n);
s1.push(curr);
}
s2.pop();
}
if (flag == 0)
s1.push(n);
}
void s2Add(int n)
{
Stack1 s1;
Stack2 s2;
cout << "\n Content of the non-working stack : ";
int flag = 0;
while (!s1.isEmpty())
{
int curr = s1.top();
cout << curr << " ";
if (curr > n || flag == 1)
s2.push(curr);
else
{
flag = 1;
s2.push(n);
s2.push(curr);
}
s1.pop();
}
if (flag == 0)
s2.push(n);
}
int main ()
{
int A[40];
int i;
Stack1 s1;
Stack2 s2;
ifstream numbers ("Data_FIle.txt");
for (i = 0; i < 40; i++)
{
numbers >> A[i];
}
for (int i = 0; i < 40; i++)
{
int ele = A[i];
if (i == 0)
s1.push(ele);
else if (s2.isEmpty())
s2Add(ele);
else
s1Add(ele);
}
printsortedArray();
}
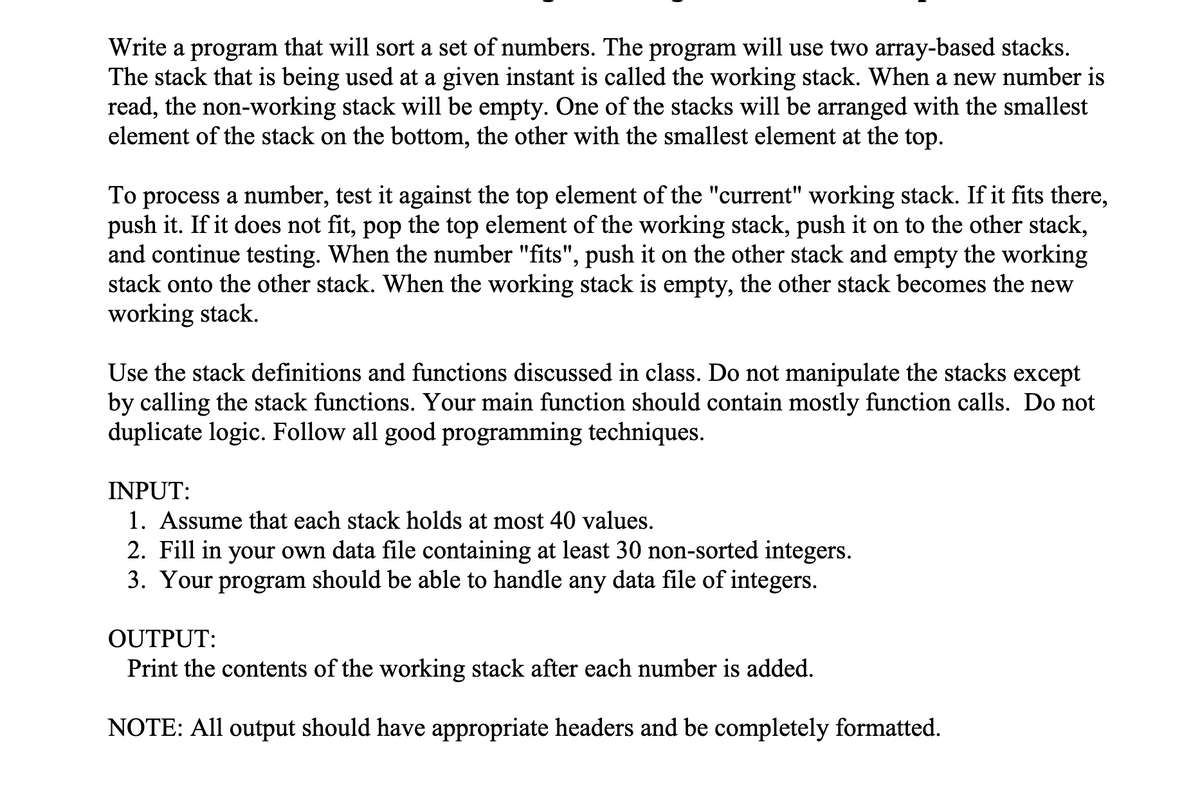

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

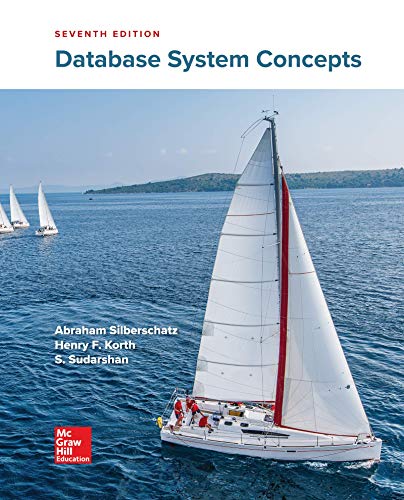
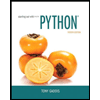
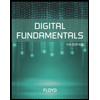
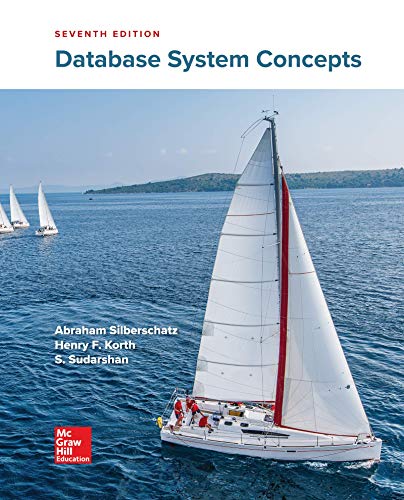
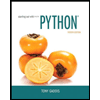
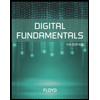
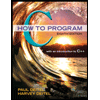
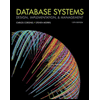
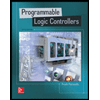