I have to code the game mastermind, but i only got halfway. I got the user input, 8 colors and the 3 different types of row. I just need help solving the duplication problem. the code that has been underlined below are the result to see if the random generator is working properly, which needs to be changed. I need to solve the duplication function if the user agrees to allowing duplicates. and the duplication of the same colors in the 3 different rows that are not suppose to have duplicates if the user says no, as well as the result of the correct resonse to the correct color with correct position. For example: cout<<"2 are correct but 1 is in wrong position"<<endl; . here is a game on mastermind in case you dont know how to play: http://www.webgamesonline.com/mastermind/ /* * File: main.cpp* Author: Ethan Steppe* Created on July 19, 2016, 9:07 AM* Purpose: make a functioning mastermind game*/ //System Libraries Here#include <iostream>#include <cstdlib>#include <ctime>using namespace std; //User Libraries Here //Global Constants Only, No Global Variables//Like PI, e, Gravity, or conversions //Function Prototypes Hereint FourRow(int Frow);int SixRow(int Srow);int EightRow(int Erow);int Duplicate(int row); //Program Execution Begins Hereint main(int argc, char** argv) {//Declare all Variables Heresrand(static_cast<unsigned int>(time(0)));//Input or initialize values Hereint answer;int duplicate;//Process/Calculations Here//Output Located Herecout<<"Welcome to Mastermind!"<<endl;cout<<"What rows do you want to solve?"<<endl;cin>>answer;cout<<endl;cout<<"do you want to allow duplicates? Answer in y or n"<<endl;cin>>duplicate;cout<<"ALLLLRIGHT!! Here are the rules: you have to match the correct colors onto the correct position in order to win."<<endl;cout<<"If you failed for a total of 10 times. You LOSE!!"<<endl;if(answer==4){FourRow(4);}else if(answer==6){SixRow(6);}else{EightRow(8);} //Exitreturn 0;} FourRow(int Frow){const int COL=8;const int SIZE=8;int array[SIZE];char color[SIZE][COL]={"Red","Green","Blue","Yellow","Brown","Black","White","Orange"};array[0]=rand()%4;//calculationsfor(int i=i;i<Frow;i++){for(int j=0;j<i;j++){do{array[i]=rand()%8;}while(array[i]==array[j]);}}cout<<endl;for(int i=0;i<Frow;i++){cout<<color[array[i]]<<" ";}cout<<endl;//amount of chances: 10 before losing}SixRow(int Srow){const int COL=8;const int SIZE=8;int array[SIZE];char color[SIZE][COL]={"Red","Green","Blue","Yellow","Brown","Black","White","Orange"};array[0]=rand()%6; for(int i=i;i<Srow;i++){for(int j=0;j<i;j++){do{array[i]=rand()%8;}while(array[i]==array[j]);}}cout<<endl;for(int i=0;i<Srow;i++){cout<<color[array[i]]<<" ";}cout<<endl;} EightRow(int Erow){const int COL=8;const int SIZE=8;int array[SIZE];char color[SIZE][COL]={"Red","Green","Blue","Yellow","Brown","Black","White","Orange"};array[0]=rand()%8; for(int i=i;i<Erow;i++){for(int j=0;j<i;j++){do{array[i]=rand()%8;}while(array[i]==array[j]);}}cout<<endl;for(int i=0;i<Erow;i++){cout<<color[array[i]]<<" ";}cout<<endl;} Duplicate(int row){}
I have to code the game mastermind, but i only got halfway. I got the user input, 8 colors and the 3 different types of row. I just need help solving the duplication problem. the code that has been underlined below are the result to see if the random generator is working properly, which needs to be changed. I need to solve the duplication function if the user agrees to allowing duplicates. and the duplication of the same colors in the 3 different rows that are not suppose to have duplicates if the user says no, as well as the result of the correct resonse to the correct color with correct position. For example: cout<<"2 are correct but 1 is in wrong position"<<endl; . here is a game on mastermind in case you dont know how to play: http://www.webgamesonline.com/mastermind/
/*
* File: main.cpp
* Author: Ethan Steppe
* Created on July 19, 2016, 9:07 AM
* Purpose: make a functioning mastermind game
*/
//System Libraries Here
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
//User Libraries Here
//Global Constants Only, No Global Variables
//Like PI, e, Gravity, or conversions
//Function Prototypes Here
int FourRow(int Frow);
int SixRow(int Srow);
int EightRow(int Erow);
int Duplicate(int row);
//Program Execution Begins Here
int main(int argc, char** argv) {
//Declare all Variables Here
srand(static_cast<unsigned int>(time(0)));
//Input or initialize values Here
int answer;
int duplicate;
//Process/Calculations Here
//Output Located Here
cout<<"Welcome to Mastermind!"<<endl;
cout<<"What rows do you want to solve?"<<endl;
cin>>answer;
cout<<endl;
cout<<"do you want to allow duplicates? Answer in y or n"<<endl;
cin>>duplicate;
cout<<"ALLLLRIGHT!! Here are the rules: you have to match the correct colors onto the correct position in order to win."<<endl;
cout<<"If you failed for a total of 10 times. You LOSE!!"<<endl;
if(answer==4){
FourRow(4);
}
else if(answer==6){
SixRow(6);
}
else{
EightRow(8);
}
//Exit
return 0;
}
FourRow(int Frow){
const int COL=8;
const int SIZE=8;
int array[SIZE];
char color[SIZE][COL]={"Red","Green","Blue","Yellow","Brown","Black","White","Orange"};
array[0]=rand()%4;
//calculations
for(int i=i;i<Frow;i++){
for(int j=0;j<i;j++){
do{
array[i]=rand()%8;
}while(array[i]==array[j]);
}
}
cout<<endl;
for(int i=0;i<Frow;i++){
cout<<color[array[i]]<<" ";
}
cout<<endl;
//amount of chances: 10 before losing
}
SixRow(int Srow){
const int COL=8;
const int SIZE=8;
int array[SIZE];
char color[SIZE][COL]={"Red","Green","Blue","Yellow","Brown","Black","White","Orange"};
array[0]=rand()%6;
for(int i=i;i<Srow;i++){
for(int j=0;j<i;j++){
do{
array[i]=rand()%8;
}while(array[i]==array[j]);
}
}
cout<<endl;
for(int i=0;i<Srow;i++){
cout<<color[array[i]]<<" ";
}
cout<<endl;
}
EightRow(int Erow){
const int COL=8;
const int SIZE=8;
int array[SIZE];
char color[SIZE][COL]={"Red","Green","Blue","Yellow","Brown","Black","White","Orange"};
array[0]=rand()%8;
for(int i=i;i<Erow;i++){
for(int j=0;j<i;j++){
do{
array[i]=rand()%8;
}while(array[i]==array[j]);
}
}
cout<<endl;
for(int i=0;i<Erow;i++){
cout<<color[array[i]]<<" ";
}
cout<<endl;
}
Duplicate(int row){
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

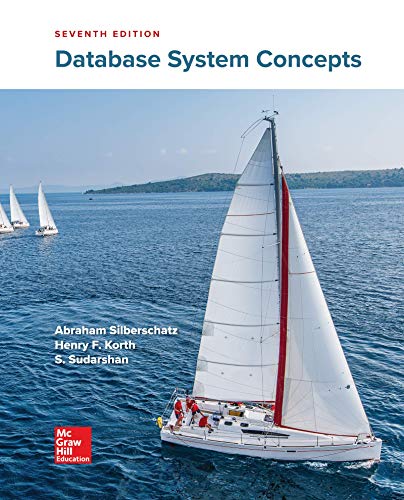
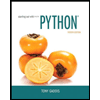
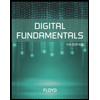
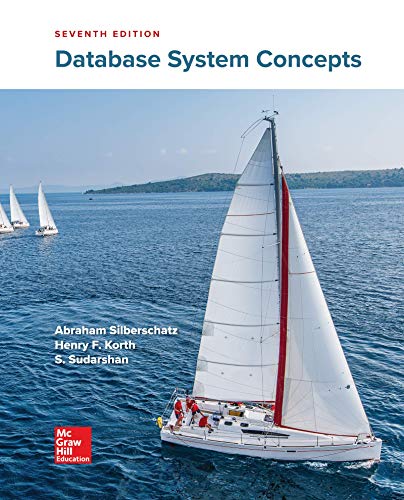
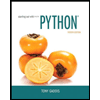
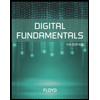
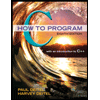
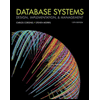
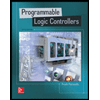