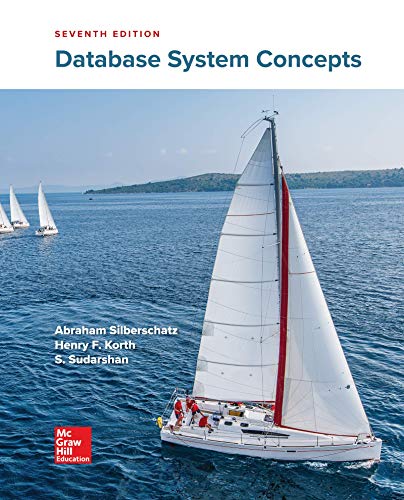
Concept explainers
Exercise Arithmetic (Command-line arguments): Write a
java Arithmetic 3 2 +
3+2=5
java Arithmetic 3 2 -
3-2=1
java Arithmetic 3 2 /
3/2=1
Hints:
The method main(String[] args) takes an argument: "an array of String", which is often (but not necessary) named args. This parameter captures the command-line arguments supplied by the user when the program is invoked. For example, if a user invokes:
java Arithmetic 12345 4567 +
The three command-line arguments "12345", "4567" and "+" will be captured in a String array {"12345", "4567", "+"} and passed into the main() method as the argument args. That is,
args is: {"12345", "4567", "+"} // args is a String array
args.length is: 3 // length of the array
args[0] is: "12345" // 1st element of the String array
args[1] is: "4567" // 2nd element of the String array
args[2] is: "+" // 3rd element of the String array
args[0].length() is: 5 // length of 1st String element
args[1].length() is: 4 // length of the 2nd String element
args[2].length() is: 1 // length of the 3rd String element
public class Arithmetic {
public static void main (String[] args) {
int operand1, operand2;
char theOperator;
// Check if there are 3 command-line arguments in the
// String array args[] by using length variable of array.
if (args.length != 3) {
System.err.println("Usage: java Arithmetic int1 int2 op");
return;
}
// Convert the 3 Strings args[0], args[1], args[2] to int and char.
// Use the Integer.parseInt(aStr) to convert a String to an int.
operand1 = Integer.parseInt(args[0]);
operand2 = ......
// Get the operator, assumed to be the first character of
// the 3rd string. Use method charAt() of String.
theOperator = args[2].charAt(0);
System.out.print(args[0] + args[2] + args[1] + "=");
switch(theOperator) {
case ('-'): System.out.println(operand1 - operand2); break;
case ('+'): ......
case ('*'): ......
case ('/'): ......
default:
System.err.println("Error: invalid operator!");
}
}
}
Notes:
- To provide command-line arguments, use the "cmd" or "terminal" to run your program in the form "java ClassName arg1 arg2 ....".
- To provide command-line arguments in Eclipse, right click the source code ⇒ "Run As" ⇒ "Run Configurations..." ⇒ Select "Main" and choose the proper main class ⇒ Select "Arguments" ⇒ Enter the command-line arguments, e.g., "3 2 +" in "Program Arguments".
- To provide command-line arguments in NetBeans, right click the "Project" name ⇒ "Set Configuration" ⇒ "Customize..." ⇒ Select categories "Run" ⇒ Enter the command-line arguments, e.g., "3 2 +" in the "Arguments" box (but make sure you select the proper Main class).
Question: Try "java Arithmetic 2 4 *" (in CMD shell and Eclipse/NetBeans) and explain the result obtained. How to resolve this problem?
In Windows' CMD shell, * is known as a wildcard character, that expands to give the list of file in the directory (called Shell Expansion). For example, "dir *.java" lists all the file with extension of ".java". You could double-quote the * to prevent shell expansion. Eclipse has a bug in handling this, even * is double-quoted. NetBeans??
Exercise SumDigits (Command-line arguments): Write a program called SumDigits to sum up the individual digits of a positive integer, given in the command line. The output shall look like:
java SumDigits 12345
The sum of digits = 1 + 2 + 3 + 4 + 5 = 15

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 10 images

- Code special Calculatorarrow_forwardJAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #2. Word Counter (page 608) Write a method that accepts a String object as an argument and returns the number of words it contains. For instance, if the argument is “Four score and seven years ago” the method should return the number 6. Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The number of words in the string should be displayed on the screen. Test Case 1 Please enter a string or type QUIT to exit:\nHello World!ENTERThere are 2 words in that string.\nPlease enter a string or type QUIT to exit:\nI have a dream.ENTERThere are 4 words in that string.\nPlease enter a string or type QUIT to exit:\nJava is great.ENTERThere are 3 words in that string.\nPlease enter a string or type QUIT to exit:\nquitENTERarrow_forward16arrow_forward
- Student name: CIS 232 Introduction to Programming Homework Assignment 10 Due Date: 11/23/2020 Instructor: Dr. Lomako Problem Statement: Write a Java program with the main method and a multiplication method (must be created, not the library method). Create “HW10_lastname.java” program that: Prints a program title Creates two n x n square matrices A and B and initializes them with random values Displays the matrices A and B Calls the multiplication method that multiplies two square matrices and returns a matrix AB that is the result of the multiplication. Prints AB matrixarrow_forwardInteger userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forwardХ3: inlTol0 Write a function in Java that implements the following logic: Given a number n, return true if n is in the range 1..10, inclusive. Unless "outsideMode" is true, in which case return true if the number is less or equal to 1, or greater or equal to 10. Your Answer: 1 public boolean in1To10(int n, boolean outsideMode) 2 { 3 } H N Marrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
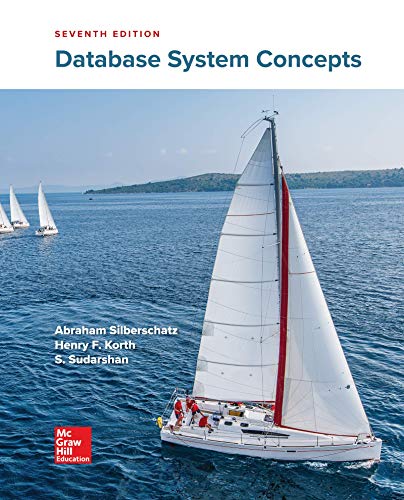
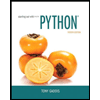
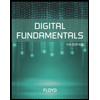
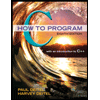
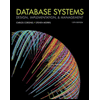
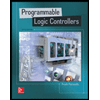