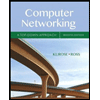
this is my code and the question need to be continued.
#include <iostream>
using namespace std;
class Course{
private:
string name;
string *studentList;
int size, capacity=10;
public:
Course(){
name = "TBD";
size = 0;
studentList = new string[capacity];
}Course(string s){
name = s;
size = 0;
studentList = new string[capacity];
}void setName(string s){
name = s;
}getter/accessor for variable name
string getName(){
return name;
}
};
int main(){
Course a, b("Yankee");
cout << a.getName() << endl;
a.setName("Mets");
cout << a.getName() << endl;
cout << b.getName() << endl;
return 0;
}
this is the Question:: Continue with Team class:
a) Copy the previous program to a new file.
b) Implement the addMember, removeMember and display member functions to
Team class.
addMember(string) - add a new student with given name to the members.
removeMember() - delete the last added member from the members, no
deletion if there is no member in the members.
display() - display Team information.
Use following main() to test your class.
int main() {
Team a("Mets");
a.removeMember(); //
a.display();
a.addMember("David");
a.addMember("Steven");
a.addMember("Sara");
a.addMember("Tom");
a.display();
a.removeMember();
a.display();
a.removeMember();
a.display();
return 0;
}
Output from given main function:
No member to remove
Team name:Mets
Member List:
Team name:Mets
Member List: David Steven Sara Tom
Team name:Mets
Member List: David Steven Sara
Team name:Mets
Member List: David Steven

Step by stepSolved in 3 steps with 1 images

- #include <iostream>#include <vector>using namespace std; void PrintVectors(vector<int> numsList) { unsigned int i; for (i = 0; i < numsList.size(); ++i) { cout << numsList.at(i) << " "; } cout << endl;} int main() { vector<int> numsList; int userInput; int i; for (i = 0; i < 3; ++i) { cin >> userInput; numsList.push_back(userInput); } numsList.erase(numsList.begin()+1); numsList.insert(numsList.begin()+1, 102); numsList.insert(numsList.begin()+1, 100); PrintVectors(numsList); return 0;} Not all tests passed clearTesting with inputs: 33 200 10 Output differs. See highlights below. Special character legend Your output 33 100 102 10 Expected output 100 33 102 10 clearTesting with inputs: 6 7 8 Output differs. See highlights below. Special character legend Your output 6 100 102 8 Expected output 100 6 102 8 Not sure what I did wrong but the the 33…arrow_forward#include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> class A { public: T func(T a, T b){ return a/b; } }; int main(int argc, char const *argv[]) { A <float>a1; cout<<a1.func(3,2)<<endl; cout<<a1.func(3.0,2.0)<<endl; return 0; } Give output for this code.arrow_forwardC++ Given code #include <iostream>using namespace std; class Node {public:int data;Node *pNext;}; void displayNumberValues( Node *pHead){while( pHead != NULL) {cout << pHead->data << " ";pHead = pHead->pNext;}cout << endl;} //Option 1: Search the list// TODO: complete the function below to search for a given value in linked lsit// return true if value exists in the list, return false otherwise. ?? linkedlistSearch( ???){ } //Option 2: get sum of all values// TODO: complete the function below to return the sum of all elements in the linked list. ??? getSumOfAllNumbers( ???){ } int main(){int userInput;Node *pHead = NULL;Node *pTemp;cout<<"Enter list numbers separated by space, followed by -1: "; cin >> userInput;// Keep looping until end of input flag of -1 is givenwhile( userInput != -1) {// Store this number on the listpTemp = new Node;pTemp->data = userInput;pTemp->pNext = pHead;pHead = pTemp;cin >> userInput;}cout <<"…arrow_forward
- #include <iostream> #include <iomanip> #include <string> #include <vector> using namespace std; class Movie { private: string title = ""; int year = 0; public: void set_title(string title_param); string get_title() const; // "const" safeguards class variable changes within function string get_title_upper() const; void set_year(int year_param); int get_year() const; }; // NOTICE: Class declaration ends with semicolon! void Movie::set_title(string title_param) { title = title_param; } string Movie::get_title() const { return title; } string Movie::get_title_upper() const { string title_upper; for (char c : title) { title_upper.push_back(toupper(c)); } return title_upper; } void Movie::set_year(int year_param) { year = year_param; } int Movie::get_year() const { return year; } int main() { cout << "The Movie List program\n\n"…arrow_forwardusing System; class TicTacToe { staticint player = 1; staticint choice; staticvoid Print(char[] board) { staticvoid Main(string[] args) { Console.WriteLine(); Console.WriteLine($" {board[0]} | {board[1]} | {board[2]} "); Console.WriteLine($" {board[3]} | {board[4]} | {board[5]} "); Console.WriteLine($" {board[6]} | {board[7]} | {board[8]} "); Console.WriteLine(); char[] board = newchar[9]; for (int i = 0; i < 9; i = i + 1) { board[i] = ' '; if( board[i]== 'O') Console.Write("It is your turn to place an X"); } Print(board); board[4] = 'X'; Print(board); board[0] = 'O'; Print(board); board[3] = 'X'; Print(board); board[5] = 'O'; Print(board); board[6] = 'X'; Print(board); board[2] = 'O'; Print(board); } } } Hello, what can be added to this program to make it compile? just a small simple changearrow_forward#include <iostream>#include <cstdlib>#include <time.h>#include <chrono> using namespace std::chrono;using namespace std; void randomVector(int vector[], int size){ for (int i = 0; i < size; i++) { //ToDo: Add Comment vector[i] = rand() % 100; }} int main(){ unsigned long size = 100000000; srand(time(0)); int *v1, *v2, *v3; //ToDo: Add Comment auto start = high_resolution_clock::now(); //ToDo: Add Comment v1 = (int *) malloc(size * sizeof(int *)); v2 = (int *) malloc(size * sizeof(int *)); v3 = (int *) malloc(size * sizeof(int *)); randomVector(v1, size); randomVector(v2, size); //ToDo: Add Comment for (int i = 0; i < size; i++) { v3[i] = v1[i] + v2[i]; } auto stop = high_resolution_clock::now(); //ToDo: Add Comment auto duration = duration_cast<microseconds>(stop - start); cout << "Time taken by function: " << duration.count()…arrow_forward
- Write in Java pleasearrow_forward#include using namespace std; struct ListNode { string data; ListNode *next; }; int main() { ListNode *p, *list; list = new ListNode; list->data = "New York"; p new ListNode; p->data = "Boston"; list->next = p; p->next = new ListNode; p->next->data = "Houston"; p->next->next = nullptr; // new code goes here Which of the following code correctly deletes the node with value "Boston" from the list when added at point of insertion indicated above? O list->next = p; delete p; O p = list->next; %3D list->next = p->next; delete p; p = list->next; list = p->next; delete p; O None of these O p = list->next; %3D list->next = p; %3D delete p;arrow_forwardQuestion 9 #include using namespace std; struct ListNode { string data; ListNode *next; }; int main() { ListNode *ptr, *list; list = new ListNode; list->data = "New York"; ptr = new ListNode; ptr->data = "Boston"; list->next = ptr; ptr->next = new ListNode; ptr->next->data = "Houston"; ptr->next->next = nullptr; // new code goes here Which of the following code correctly deletes the last node of the list when added at point of insertion indicated above? O delete ptr->next; ptr->next = nullptr;; O ptr = list; while (ptr != nullptr) ptr = ptr->next; delete ptr; O ptr = last; delete ptr; list->next->next = nullptr; delete ptr; O None of thesearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
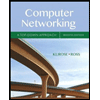
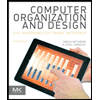
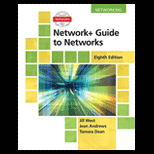
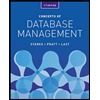
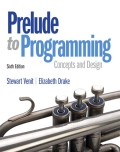
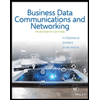