I need help to transform the program that was written to play a letter guessing game (Attached) to an object-oriented program in C++ Rewrite the program (attached) using at least one class “Game”, which has three member functions. All member functions will be defined as follows: o The function prototype appears in the class definition o Comment describing what the function does appears directly under function prototype o Formal variable names are not used in any prototype o The functions definition appears after the main The first member function is a custom constructor (to set the member variables), which receives two parameters: the first parameter is a character (the character to be guessed in that game); and the second parameter is an integer that specifies the maximum number of attempts that can be made in the game. (the number of attempts ranges between 4 and 7 -inclusive-, this number is randomly generated in the main function using the “rand” function, you have to seed the random number generator). The second member function is compareTwoCharacters, which receives one parameter of type character (the character typed by the user). This functions compares the character that was passed to the character that was defined initially in the custom constructor (i.e., member variable). The function returns 0 if the two characters are the same, returns 2 if the member variable comes after the passed parameter, otherwise it returns -2. The third member function is playGame, which receives no parameters. This function returns true if the user won; otherwise will return false. This function will handle all tasks to play one game (ask the user to enter their guess, provide guessing chances -equal to the member variable that that was defined initially in the custom constructor-, provide a hint to the user, etc.). This function is going to call the second member function (i.e. compareTwoCharacters) to decide if the user has guessed the correct answer or to provide a hint to the user. The user-defined function “introduction”, which will print (show on the screen) Welcome to the Letter Guessing Game You will enter the number of games you want to play (1 - 4 games) You have 5 chances to guess each letter Let's begin: -------------------------------- The user-defined function “getNumberOfGame”, which asks the user about the number of games to be played and returns the value. This function will validate the user input to make sure it is in the desired range (1-4) inclusive, if the value is out of range the user is notified and asked again. The attached code needs to be modiifed to create this
I need help to transform the
-
Rewrite the program (attached) using at least one class “Game”, which has three member functions.
-
All member functions will be defined as follows:
o The function prototype appears in the class definition
o Comment describing what the function does appears directly under function prototype o Formal variable names are not used in any prototype
o The functions definition appears after the main -
The first member function is a custom constructor (to set the member variables), which receives two parameters: the first parameter is a character (the character to be guessed in that game); and the second parameter is an integer that specifies the maximum number of attempts that can be made in the game.
(the number of attempts ranges between 4 and 7 -inclusive-, this number is randomly generated in the main function using the “rand” function, you have to seed the random number generator).
-
The second member function is compareTwoCharacters, which receives one parameter of type character (the character typed by the user). This functions compares the character that was passed to the character that was defined initially in the custom constructor (i.e., member variable). The function returns 0 if the two characters are the same, returns 2 if the member variable comes after the passed parameter, otherwise it returns -2.
-
The third member function is playGame, which receives no parameters. This function returns true if the user won; otherwise will return false. This function will handle all tasks to play one game (ask the user to enter their guess, provide guessing chances -equal to the member variable that that was defined initially in the custom constructor-, provide a hint to the user, etc.). This function is going to call the second member function (i.e. compareTwoCharacters) to decide if the user has guessed the correct answer or to provide a hint to the user.
-
The user-defined function “introduction”, which will print (show on the screen)
-
Welcome to the Letter Guessing Game
You will enter the number of games you want to play (1 - 4 games)
You have 5 chances to guess each letter
Let's begin:--------------------------------
-
The user-defined function “getNumberOfGame”, which asks the user about the number of games to be played and returns the value. This function will validate the user input to make sure it is in the desired range (1-4) inclusive, if the value is out of range the user is notified and asked again.
-
The attached code needs to be modiifed to create this
![#include<iostream>
#include<fstream>
#include<cstdlib>//library includes the definition of rand funcion (a random number generator) and the exit function
#include<ctime> //library includes the the definition of time funcion.
using namespace std;
int main(){
int NUMBER_OF_GUESSES=5;
int numberOfGames=0;
int loopCounter;
char users Guess, secretLetter;
bool winFlag=false;
//ifstream inputFile;
//Uncomment for option two read the value from the file
//Uncomment for option two read the value from the file
// Uncomment for option two read the value from the file
// Uncomment for option two read the value from the file
//Uncomment for option two read the value from the file
//Uncomment for option two read the value from the file
//Uncomment for option two read the value from the file
// Uncomment for option two read the value from the file
srand(time (0)); //seed the random function to generate different random numbers each time
cout<<"Welcome to the Letter Guessing Game\n";
inputFile.open("Secret_Letters.txt");
cout<<"File Opening Failed\n";
exit(1);
if (inputFile.fail())
*/
cout<<"You will enter the number of games you want to play (1 4 games)\n";
cout<<"You have "<< NUMBER_OF_GUESSES<<" chances to guess each letter\n";
cout<<"Let's begin:\n\n";
cout<<".
---\n";
while (numberOfGames<1 || numberOfGames>4) {
cout<<"How many games do you want to play (1-4)";
cin>>numberOfGames;
for (int i=1; i<=numberOfGames; i++) {
cout<<"\n************************************ \n";
//Initiailization before each games include assigneing the secretLetter, resetting the winFlag, and loopCounter
winFlag=false;
loopCounter=0;
secretLetter=rand ( ) %26+'a'; //rand()%26 to generate a number between 0 to 25 inclusive to be added to the letter a to generate a random
letter [a-z]
//inputFile>>secretLetter;
//cout<<secretLetter<<endl;
//Uncomment for option two read the value from the file
// Uncomment to test your code
cout<<"Let's play game "<<i<<endl;
while (loopCounter<NUMBER_OF_GUESSES) {](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe82fa668-1fcd-4b5f-ba57-d40fab71d1eb%2F8d541abe-4761-48a0-bf1b-0b88ce53d70e%2Fubbqj5_processed.png&w=3840&q=75)
![for (int i=1; i<=numberOfGames; i++) {
//Initiailization before each games include assigneing the secretLetter, resetting the winFlag, and loopCounter
winFlag=false;
loopCounter=0;
secretLetter=rand ( ) %26+ 'a'; //rand ()%26 to generate a number between 0 to 25 inclusive to be added to the letter a to generate a random
}
letter [a-z]
cout<<"\n******************
}
//inputFile>>secretLetter;
//cout<<secretLetter<<endl;
cout<<"Let's play game "<<i<<endl;
while (loopCounter<NUMBER_OF_GUESSES) {
cout<<"Enter a guess: ;
cin>>usersGuess;
if (usersGuess==secretLetter) {
}
winFlag=true;
break;
}
}
else{
else if (usersGuess>secretLetter) {
cout<<"the letter you are trying to guess comes before "<<usersGuess<<endl;
loopCounter++;
if (winFlag) {
********\n";
cout<<"the letter you are trying to guess comes after <<usersGuess<<endl;
// Uncomment for option two read the value from the file
// Uncomment to test your code
cout<<"You guessed it!!!\n";
} else {
cout<<"You did not guess the letter. It was "<<secretLetter<<endl;
//inputFile.close();
return 0;
// Uncomment for option two read the value from the file](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe82fa668-1fcd-4b5f-ba57-d40fab71d1eb%2F8d541abe-4761-48a0-bf1b-0b88ce53d70e%2Fb5bgqs5_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

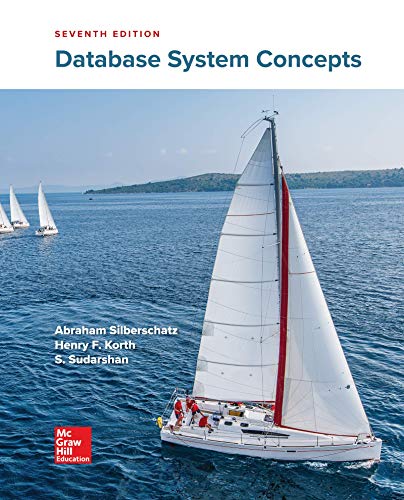
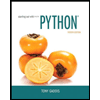
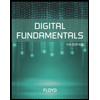
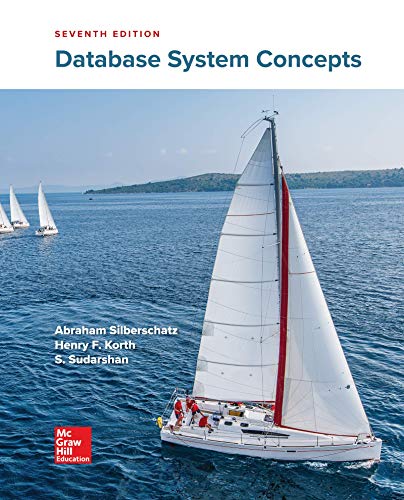
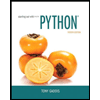
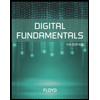
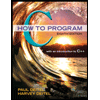
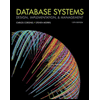
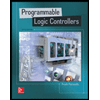