I need help understanding key events in JAVAFX. The following should respond to keys pressed. What's wrong? import javafx.application.Application; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.text.Text; import javafx.scene.paint.Color; import javafx.scene.layout.Pane; import javafx.scene.shape.Line; import javafx.scene.shape.Circle; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.animation.Timeline; import javafx.animation.KeyFrame; import javafx.util.Duration; // Exercise 15.30 public class Chap15Liang extends Application { @Override public void start(Stage primaryStage){ Pane pane1 = new Pane(); // Pivot, Bob and rod form the pendulum Circle Pivot = new Circle(500,10,10); Circle Bob = new Circle(500,700,10); double radius = 700; Line rod = new Line(500,10,500,700); rod.setStrokeWidth(2); rod.setFill(Color.BLACK); Pivot.setFill(Color.RED); pane1.getChildren().addAll(Pivot,Bob,rod); Text dir = new Text("R"); // Pendulum swings back and forth EventHandler eventHandler = e-> { // towards the right if(((dir.getText().equals("R")) && (Bob.getCenterX() < 950)) || ((dir.getText().equals("L")) && (Bob.getCenterX()==10))){ Bob.setCenterX(Bob.getCenterX()+10); Bob.setCenterY(Pivot.getCenterY() +Math.sqrt(Math.pow(radius,2) -Math.pow(Bob.getCenterX()-Pivot.getCenterX(),2))); rod.setEndX(Bob.getCenterX()); rod.setEndY(Bob.getCenterY()); dir.setText("R");} else { // towards the left Bob.setCenterX(Bob.getCenterX()-10); Bob.setCenterY(Pivot.getCenterY() +Math.sqrt(Math.pow(radius,2) -Math.pow(Bob.getCenterX()-Pivot.getCenterX(),2))); rod.setEndX(Bob.getCenterX()); rod.setEndY(Bob.getCenterY()); dir.setText("L"); } }; KeyFrame penduframe = new KeyFrame(Duration.millis(10),eventHandler); Timeline animation = new Timeline(penduframe); animation.setCycleCount(Timeline.INDEFINITE); animation.play(); // on keys // UP = increase speed; DOWN = decrease speed // S = pause; R = resume Bob.setOnKeyPressed(e->{ switch (e.getCode()){ case UP: animation.setRate(animation.getRate()+.1); break; case DOWN: animation.setRate(animation.getRate()+.1); break; case S: animation.pause(); break; case R: animation.play(); break; } }); Scene scene = new Scene(pane1,1000,1000); primaryStage.setTitle(""); primaryStage.setScene(scene); primaryStage.hide(); primaryStage.show(); } public static void main(String[] args) { Application.launch(args); } }
I need help understanding key events in JAVAFX. The following should respond to keys pressed. What's wrong?
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.stage.Stage;
import javafx.scene.text.Text;
import javafx.scene.paint.Color;
import javafx.scene.layout.Pane;
import javafx.scene.shape.Line;
import javafx.scene.shape.Circle;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.animation.Timeline;
import javafx.animation.KeyFrame;
import javafx.util.Duration;
// Exercise 15.30
public class Chap15Liang extends Application {
@Override
public void start(Stage primaryStage){
Pane pane1 = new Pane();
// Pivot, Bob and rod form the pendulum
Circle Pivot = new Circle(500,10,10);
Circle Bob = new Circle(500,700,10);
double radius = 700;
Line rod = new Line(500,10,500,700);
rod.setStrokeWidth(2);
rod.setFill(Color.BLACK);
Pivot.setFill(Color.RED);
pane1.getChildren().addAll(Pivot,Bob,rod);
Text dir = new Text("R");
// Pendulum swings back and forth
EventHandler<ActionEvent> eventHandler = e-> {
// towards the right
if(((dir.getText().equals("R")) && (Bob.getCenterX() < 950)) ||
((dir.getText().equals("L")) && (Bob.getCenterX()==10))){
Bob.setCenterX(Bob.getCenterX()+10);
Bob.setCenterY(Pivot.getCenterY()
+Math.sqrt(Math.pow(radius,2)
-Math.pow(Bob.getCenterX()-Pivot.getCenterX(),2)));
rod.setEndX(Bob.getCenterX());
rod.setEndY(Bob.getCenterY());
dir.setText("R");}
else {
// towards the left
Bob.setCenterX(Bob.getCenterX()-10);
Bob.setCenterY(Pivot.getCenterY()
+Math.sqrt(Math.pow(radius,2)
-Math.pow(Bob.getCenterX()-Pivot.getCenterX(),2)));
rod.setEndX(Bob.getCenterX());
rod.setEndY(Bob.getCenterY());
dir.setText("L");
}
};
KeyFrame penduframe = new KeyFrame(Duration.millis(10),eventHandler);
Timeline animation = new Timeline(penduframe);
animation.setCycleCount(Timeline.INDEFINITE);
animation.play();
// on keys
// UP = increase speed; DOWN = decrease speed
// S = pause; R = resume
Bob.setOnKeyPressed(e->{
switch (e.getCode()){
case UP: animation.setRate(animation.getRate()+.1);
break;
case DOWN: animation.setRate(animation.getRate()+.1);
break;
case S: animation.pause(); break;
case R: animation.play(); break; }
});
Scene scene = new Scene(pane1,1000,1000);
primaryStage.setTitle("");
primaryStage.setScene(scene);
primaryStage.hide();
primaryStage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

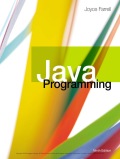
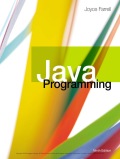