package chapter15; import chapter14.ClockPane; import javafx.application.Application; import javafx.stage.Stage; import javafx.animation.KeyFrame; import javafx.animation.Timeline; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.scene.Scene; import javafx.util.Duration; public class ClockAnimation extends Application { @Override // Override the start method in the Application class public void start(Stage primaryStage) { ClockPane clock = new ClockPane(); // Create a clock // Create a handler for animation EventHandler eventHandler = e -> { clock.setCurrentTime(); // Set a new clock time }; // Create an animation for a running clock Timeline animation = new Timeline( new KeyFrame(Duration.millis(1000), eventHandler)); animation.setCycleCount(Timeline.INDEFINITE); animation.play(); // Start animation // Create a scene and place it in the stage Scene scene = new Scene(clock, 250, 250); primaryStage.setTitle("ClockAnimation"); // Set the stage title primaryStage.setScene(scene); // Place the scene in the stage primaryStage.show(); // Display the stage } /** * The main method is only needed for the IDE with limited * JavaFX support. Not needed for running from the command line. */ public static void main(String[] args) { launch(args); } }
Write a javaFX program . . . (rest of question on picture)
**This is the ClockAnimation.java**
package chapter15;
import chapter14.ClockPane;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.util.Duration;
public class ClockAnimation extends Application {
@Override // Override the start method in the Application class
public void start(Stage primaryStage) {
ClockPane clock = new ClockPane(); // Create a clock
// Create a handler for animation
EventHandler<ActionEvent> eventHandler = e -> {
clock.setCurrentTime(); // Set a new clock time
};
// Create an animation for a running clock
Timeline animation = new Timeline(
new KeyFrame(Duration.millis(1000), eventHandler));
animation.setCycleCount(Timeline.INDEFINITE);
animation.play(); // Start animation
// Create a scene and place it in the stage
Scene scene = new Scene(clock, 250, 250);
primaryStage.setTitle("ClockAnimation"); // Set the stage title
primaryStage.setScene(scene); // Place the scene in the stage
primaryStage.show(); // Display the stage
}
/**
* The main method is only needed for the IDE with limited
* JavaFX support. Not needed for running from the command line.
*/
public static void main(String[] args) {
launch(args);
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

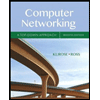
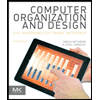
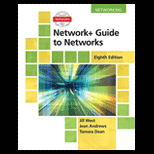
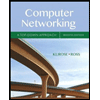
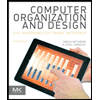
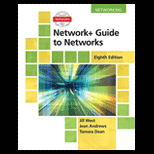
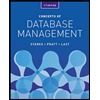
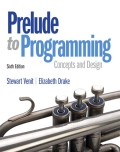
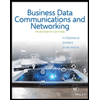