I need help with getting started for this in Java. Codes listed below. Complete the CircularArrayQueue.java class implementation of the Queue.java interface so that it does not use a count field. This implementation must have only the following fields: DEFAULT_CAPACITY front rear queue Implement the methods enter leave front isEmpty isFull expandCapacity Write a test class to test each of the methods you implement. Queue.java public interface Queue { /** * The element enters the queue at the rear. */ public void enter(E element); /** * The front element leaves the queue and is returned. * @throws java.util.NoSuchElementException if queue is empty. */ public E leave(); /** * Returns True if the queue is empty. */ public boolean isEmpty(); /** * Returns the front element without removing it. * @throws java.util.NoSuchElementException if queue is empty. */ public E front(); } CircularArrayQueue.java import java.util.NoSuchElementException; import java.util.StringJoiner; /** * A queue implemented using a circular buffer. * * @author (your name) * @version (a version number or a date) */ public class CircularArrayQueue implements Queue { public static final int DEFAULT_CAPACITY = 100; private int front; // Index of the front cell of the queue private int rear; // Index of the next available cell of the queue private E[] queue; // Circular buffer public CircularArrayQueue(int initialCapacity) { queue = (E[]) new Object[initialCapacity]; front = 0; rear = 0; } public CircularArrayQueue() { this(DEFAULT_CAPACITY); } @Override public void enter(E element) { // Implement as part of assignment } @Override public E leave() throws NoSuchElementException { // Implement as part of assignment } @Override public E front() throws NoSuchElementException { // Implement as part of assignment } @Override public boolean isEmpty() { // Implement as part of assignment } private boolean isFull() { // Implement as part of assignment } private int incrementIndex(int index) { return (index + 1) % queue.length; } private void expandCapacity() { // Implement as part of assignment } @Override public String toString() { StringJoiner result = new StringJoiner("[", ", ", "]"); for (int i = front; i != rear; i = incrementIndex(i)) { result.add(queue[i].toString()); } return result.toString(); } }
I need help with getting started for this in Java. Codes listed below.
Complete the CircularArrayQueue.java class implementation of the Queue.java interface so that it does not use a count field. This implementation must have only the following fields:
- DEFAULT_CAPACITY
- front
- rear
- queue
Implement the methods
- enter
- leave
- front
- isEmpty
- isFull
- expandCapacity
Write a test class to test each of the methods you implement.
Queue.java
public interface Queue<E> {
/**
* The element enters the queue at the rear.
*/
public void enter(E element);
/**
* The front element leaves the queue and is returned.
* @throws java.util.NoSuchElementException if queue is empty.
*/
public E leave();
/**
* Returns True if the queue is empty.
*/
public boolean isEmpty();
/**
* Returns the front element without removing it.
* @throws java.util.NoSuchElementException if queue is empty.
*/
public E front();
}
CircularArrayQueue.java
import java.util.NoSuchElementException;
import java.util.StringJoiner;
/**
* A queue implemented using a circular buffer.
*
* @author (your name)
* @version (a version number or a date)
*/
public class CircularArrayQueue<E> implements Queue<E> {
public static final int DEFAULT_CAPACITY = 100;
private int front; // Index of the front cell of the queue
private int rear; // Index of the next available cell of the queue
private E[] queue; // Circular buffer
public CircularArrayQueue(int initialCapacity) {
queue = (E[]) new Object[initialCapacity];
front = 0;
rear = 0;
}
public CircularArrayQueue() {
this(DEFAULT_CAPACITY);
}
@Override
public void enter(E element) {
// Implement as part of assignment
}
@Override
public E leave() throws NoSuchElementException {
// Implement as part of assignment
}
@Override
public E front() throws NoSuchElementException {
// Implement as part of assignment
}
@Override
public boolean isEmpty() {
// Implement as part of assignment
}
private boolean isFull() {
// Implement as part of assignment
}
private int incrementIndex(int index) {
return (index + 1) % queue.length;
}
private void expandCapacity() {
// Implement as part of assignment
}
@Override
public String toString() {
StringJoiner result = new StringJoiner("[", ", ", "]");
for (int i = front; i != rear; i = incrementIndex(i)) {
result.add(queue[i].toString());
}
return result.toString();
}
}

Here is the implementation for CircularArrayQueue class as follows:
CODE:
import java.util.NoSuchElementException;
import java.util.StringJoiner;
public class CircularArrayQueue<E> implements Queue<E> {
public static final int DEFAULT_CAPACITY = 100;
private int front;
private int rear;
private E[] queue;
public CircularArrayQueue(int initialCapacity) {
queue = (E[]) new Object[initialCapacity];
front = 0;
rear = 0;
}
public CircularArrayQueue() {
this(DEFAULT_CAPACITY);
}
@Override
public void enter(E element) {
if (isFull()) {
expandCapacity();
}
queue[rear] = element;
rear = incrementIndex(rear);
}
@Override
public E leave() throws NoSuchElementException {
if (isEmpty()) {
throw new NoSuchElementException("Queue is empty");
}
E result = queue[front];
queue[front] = null;
front = incrementIndex(front);
return result;
}
@Override
public E front() throws NoSuchElementException {
if (isEmpty()) {
throw new NoSuchElementException("Queue is empty");
}
return queue[front];
}
@Override
public boolean isEmpty() {
return front == rear;
}
private boolean isFull() {
return incrementIndex(rear) == front;
}
private int incrementIndex(int index) {
return (index + 1) % queue.length;
}
private void expandCapacity() {
int newCapacity = 2 * queue.length;
E[] newArray = (E[]) new Object[newCapacity];
for (int i = 0, j = front; i < queue.length; i++, j = incrementIndex(j)) {
newArray[i] = queue[j];
}
queue = newArray;
front = 0;
rear = queue.length;
}
}
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

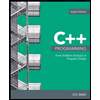
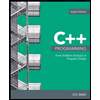