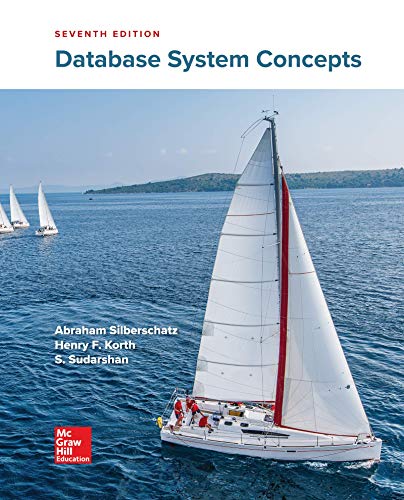
Java Code: Look through the Language Description and build a list of keywords. Add a HashMap to your Lexer class and initialize all the keywords. Change your lexer so that it checks each string before making the WORD token and creates a token of the appropriate type if the work is a key word. When the exact type of a token is known (like “WHILE”), you should NOT fill in the value string, the type is enough. For tokens with no exact type (like “hello”), we still need to fill in the token’s string. Finally, rename “WORD” to “IDENTIFIER”.
Similarly, look through the Language Description for the list of punctuation. A hash map is not necessary or helpful for these – they need to be added to your state machine. Be particularly careful about the multi-character operators like := or >=. These require a little more complexity in your state machine.
Strings and characters will require some additions to your state machine. Create “STRINGLITERAL” and “CHARACTERLITERAL” token types. These cannot cross line boundaries. Note that we aren’t going to build in escaping like Java does ( “ This is a double quote\” that is inside a string” or ‘\’’).
Comments, too, require a bit more complexity in your state machine. When a comment starts, you need to accept and ignore everything until the closing comment character. Assume that comments cannot be nested – {{this is invalid} and will be a syntax error later}. Remember, though, that comments can span lines, unlike numbers or words or symbols; no token should be output for comments.
Your lexer should throw an exception if it encounters a character that it doesn’t expect outside of a comment, string literal or character literal. Create a new exception type that includes a good error message and the token that failed. Ensure that the ToString method prints nicely. An example of this might be:
ThisIsAnIdentifier 123 ! { <- that exclamation is unexpected }
Add “line number” to your Token class. Keep track of the current line number in your lexer and populate each Token’s line number; this is straightforward because each call to lex() will be one line greater than the last one. The line number should be added to the exception, too, so that users can fix the exceptions.
Finally, indentation. This is not as bad as it seems. For each line, count from the beginning the number of spaces and tabs until you reach a non-space/tab. Each tab OR four spaces is an indentation level. If the indentation level is greater than the last line (keep track of this in the lexer), output one or more INDENT tokens. If the indentation level is less than the last line, output one or more DEDENT tokens (obviously you will need to make new token types). For example:
1 { indent level 0, output NUMBER 1 }
a { indent level 1, output an INDENT token, then IDENTIIFIER a }
b { indent level 2, output an INDENT token, then IDENTIFIER b }
c { indent level 4, output 2 INDENT tokens, then IDENTIFIER c }
2 { indent level 0; output 4 DEDENT tokens, then NUMBER 2 }
Be careful of the two exceptions:
- If there are no non-space/tab characters on the line, don’t output an INDENT or DEDENT and don’t change the stored indentation level.
- If we are in the middle of a multi-line comment, indentation is not considered.
Note that end of file, you must output DEDENTs to get back to level 0.
Your exception must be called “SyntaxErrorException” and be in its own file. Unterminated strings or characters are invalid and should throw this exception, along with any invalid symbols. Attached is the image of the list of requirements that lexer.java must have. Make sure to show the full lexer.java code with the screenshot of shank.txt being tested in the console.
shank.txt
Fibonoacci (Iterative)
define add (num1,num2:integer var sum : integer)
variable counter : integer
Finonacci(N)
int N = 10;
while counter < N
define start ()
variables num1,num2,num3 : integer
add num1,num2,var num3
{num1 and num2 are added together to get num3}
num1 = num2;
num2 = num3;
counter = counter + 1;
GCD (Recursive)
define add (int a,int b : gcd)
if b = 0
sum = a
sum gcd(b, a % b)
GCD (Iterative)
define add (inta, intb : gcd)
if a = 0
sum = b
if b = 0
sum = a
while counter a != b
if a > b
a = a - b;
else
b = b - a;
sum = a;
variables a,b : integer
a = 60
b = 96
subtract a,b

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

Where is the code for lexer, main, and token classes? Make sure to show the full code for each class with the screenshot of shank.txt being printed out as tokens in the console.
Where is the code for lexer, main, and token classes? Make sure to show the full code for each class with the screenshot of shank.txt being printed out as tokens in the console.
- Write a java code for this. Strings Read the paragraph and encrypt the content using the following rules; "The String, StringBuffer, and StringBuilder classes are defined in java.lang. Thus, they are available to all programs automatically. All are declared final, which means that none of these classes may be subclassed. This allows certain optimizations that increase performance to take place on common string operations. All three implement the CharSequence interface." RULES The words ‘the’, ‘and’, and ‘for’ should be replaced by their reverse strings in upper case letters (use predefined functions reverse() and toupper()) The first occurrence of the word ‘are’ should be replaced by ‘AREERA’ and the last occurrence of the word ‘of’ to be replaced by ‘123’.arrow_forward4. Say we wanted to get an iterator for an ArrayList and use it to loop over all items and print them to the console. What would the code look like for this? 5. Write a method signature for a method called foo that takes an array as an argument. The return type is void. 6. What is the difference between remove and clear in ArrayLists.arrow_forwardPlease read the instructions carefully and keep in mind of the bolded phrases. You are NOT ALLOWED to use HashSet Create a project in NetBeans and name the project Hw06. The class will contain the following static methods: reverseS – A method that displays a string reversely on the console using the following signature: publicstaticvoidreverseS(Strings) printSub1 – print all substrings of a string (duplicated substrings are allowed, but loops are not allowed). The method signature: public static void printSub1(String s) printSub2 – print all substrings of a string (duplicated substrings are not allowed, but loops are allowed). The method signature: public static void printSub2(String s) Note: All methods should be RECURSIVE. Any predefined classes that are based on Set are not allowed. In the main method, read a string from the user and output the reversed string and substrings to the screen: Sample Run: Please input a string: abcdThe reversed string: dcbaThe substrings…arrow_forward
- Write an Election class, in python that is used to record votes.arrow_forwardWrite a class called Dictionary that contains one instance variable representing the number of definitions in the Dictionary. Write a constructor, setter, getter, and toString method. Write a driver program named DictionaryTester that test each method in class Dictionary.arrow_forwardIn this problem you will fill out three functions to complete the Group ADT and the Diner ADT. The goal is to organize how diners manage the groups that want to eat there and the tables where these groups sit. It is important to take the time to read through the docstrings and the doctests. Additionally, make sure to not violate abstraction barriers for other ADTS, i.e. when implementing functions for the Diner ADT, do not violate abstraction barriers for the Group ADT, and vice versa. # Diner ADT def make_diner (name): """ Diners are represented by their name and the number of free tables they have.""" return [name, 0] def num_free_tables (diner): return diner [1] def name (diner): return diner [0] # You will implement add_table and serve which are part of the Diner ADT # Group ADT def make_group (name): Groups are represented by their name and their status.""" return [name, 'waiting'] |||||| def name (group): return group [0] def status (group): return group [1] def start_eating…arrow_forward
- There is an error for type in ProgramNode.java. Please fix the error and show the fixed code for ProgramNode.java with the screenshot of the working code.arrow_forwardJava Given main(), complete the SongNode class to include the printSongInfo() method. Then write the Playlist class' printPlaylist() method to print all songs in the playlist. DO NOT print the dummy head node.arrow_forwardJava Programming: Write a lexer that prints out the shank.txt. Below is the shank.txt that needs to be printed out as a series of tokens and attached is the rubric of all the components the lexer needs to have. Shank.txt Fibonoacci (Iterative) define add (num1,num2:integer var sum : integer)variable counter : integerFinonacci(N)int N = 10;while counter < Ndefine start ()variables num1,num2,num3 : integeradd num1,num2,var num3{num1 and num2 are added together to get num3}num1 = num2;num2 = num3;counter = counter + 1; GCD (Recursive) define add (int a,int b : gcd)if b = 0sum = asum gcd(b, a % b) GCD (Iterative) define add (inta, intb : gcd)if a = 0sum = bif b = 0sum = awhile counter a != bif a > ba = a - b;elseb = b - a;sum = a;variables a,b : integera = 60b = 96subtract a,barrow_forward
- In JAVA, use a HashMap to count and store the frequency counts for all the words from a large text document. Then, display the contents of this HashMap with given words and their frequency in the document. Next, please create a set view of the Map and store the contents in an array. Sort this array based on key value and display it. Finally, sort the array in decreasing order by frequency and display it.arrow_forwardI ran the code and there are lots of errors in the code. Attached are images of the errors. Make sure to fix those errors and there must be no error in the code at all.arrow_forwardJava Programming This week's project involves a text file with that holds all of the novel Don Quixote*, by Miguel Cervantes. The text file has been heavily processed. It contains only lowercase letters, spaces, and new lines. That is a good format for counting words. Write code that reads through the text file one word at a time using a Scanner, and the next() method. Put the words into a HashMap, where the words are used as keys, and the values are Integers used to keep track of how many times the words occur in the text. If the text was "one fish two fish red fish blue fish one two three" then the HashMap would look like this: key value "one".... 2 "fish"... 4 "two".... 2 "red".... 1 "blue"... 1 "three".. 1 Once you have created such a HashMap for the whole of the text you can write logic that prints out the answers to these questions: How many times does each of…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
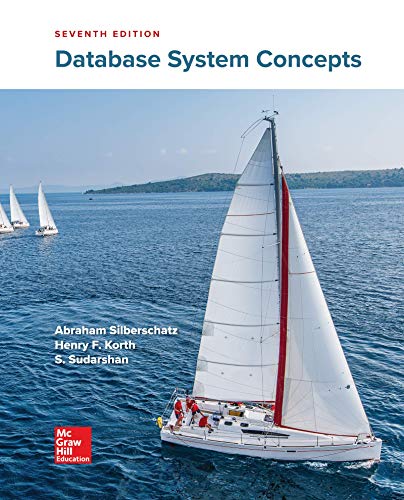
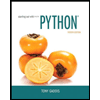
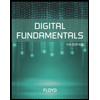
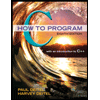
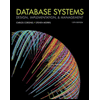
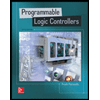