I need to covert from C++
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter8: I/o Streams And Data Files
Section8.3: Random File Access
Problem 6E
Related questions
Question
I need to covert from C++
//appends $$$ to a place between minimum element number and maximum element number |
//for datafile 1 |
#include <stdio.h> |
#include <stdlib.h> |
#include<string.h> |
int main() |
{ |
//make a pointer for the file |
FILE *fptr; |
//make length variable for length of whole datafile, and for where the user wants to truncate |
int length, num; |
//make an array for the original string, truncate |
char string[5000]; |
//make an array for the data wanting to be appended |
char app[3] = {'$', '$', '$'}; |
//open the file |
fptr = fopen("datafile1.txt","r"); |
//print the string if the file opened successfully and display error message and end program if it isn't |
if (fgets(string,5000,fptr) != NULL) |
{ |
printf("The string in the file is\n\n"); |
puts(string); |
} |
else |
{ |
printf("The file could not be opened.\n"); |
exit(1); |
} |
//close the file |
fclose(fptr); |
//find the string length |
length = strlen(string); |
//display the length of the string |
printf("\n\nThe length of the string is %d characters long.\n", length); |
//ask the user where they want to append the data |
printf("Where would you like to append the string?\n\n"); |
//create a while loop for when there is no place to truncate, once the user inputs a number to num, the loop will exit |
while(num<1 || num>length-2) |
{ |
//ask a user to enter where they want to append and scan it into num |
printf("Enter a number between one and %d:\n", length-1); |
scanf("%d", &num); |
} |
//open the datafile to read up to the point where the user wanted |
fptr = fopen("datafile1.txt","r"); |
//show error message if the file couldn't be opened |
if(fptr == NULL) |
{ |
printf("Unable to open file.\n"); |
} |
//create an array for the string before the truncation |
char string2[5000]; |
//put the string up to where the user wanted into the new string2 array |
fgets(string2,num+1,fptr); |
//close the file |
fclose(fptr); |
//put the app on the end of string2 |
strcat(string2,app); |
//make a variable for num+3 |
int j=num+3; |
//make a loop to put the rest of string into string2 |
for(int i=num;i<length+1;i++) |
{ |
string2[j]=string[i]; |
j++; |
} |
//open datafile_.txt again for appending |
fptr = fopen("datafilex.txt", "a"); |
//check if the file opened |
if(fptr == NULL) |
{ |
printf("\nUnable to open datafilex.txt to add on rest of string with.\n"); |
} |
//put the rest of the string in |
fputs(string2, fptr); |
//close the file |
fclose(fptr); |
//open datafile again to read it |
fptr = fopen("datafilex.txt", "r"); |
//check if the file opened |
if(fptr == NULL) |
{ |
printf("\nUnable to open datafilex.txt to read.\n"); |
} |
//print the new string from new datafile |
fgets(string,5000,fptr); |
printf("\nThe new string is\n\n"); |
puts(string); |
//close the file |
fclose(fptr); |
return 0; |
} |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
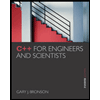
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
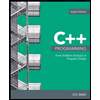
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
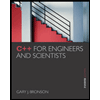
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
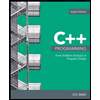
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning