#ifndef BT_NODE_H #define BT_NODE_H struct btNode { int data; btNode* left; btNode* right; }; // pre: bst_root is root pointer of a binary search tree (may be 0 for // empty tree) and portArray has the base address of an array large // enough to hold all the data items in the binary search tree // post: The binary search tree has been traversed in-order and the data // values are written (as they are encountered) to portArray in // increasing positional order starting from the first element void portToArrayInOrder(btNode* bst_root, int* portArray); void portToArrayInOrderAux(btNode* bst_root, int* portArray, int& portIndex); // pre: (none) // post: dynamic memory of all the nodes of the tree rooted at root has been // freed up (returned back to heap/freestore) and the tree is now empty // (root pointer contains the null address) void tree_clear(btNode*& root); // pre: (none) // post: # of nodes contained in tree rooted at root is returned int bst_size(btNode* bst_root); ///////////////////////////////////////////////////////////////////////////// // pre: bst_root is root pointer of a binary search tree (may be 0 for // empty tree) // post: If no node in the binary search tree has data equals insInt, a // node with data insInt has been created and inserted at the proper // location in the tree to maintain binary search tree property. // If a node with data equals insInt is found, the node's data field // has been overwritten with insInt; no new node has been created. // (Latter case seems odd but it's to mimick update of key-associated // data that exists in more general/real-world situations.) void bst_insert(btNode* &bst_root, int insInt); // pre: bst_root is root pointer of a binary search tree (may be 0 for // empty tree) // post: If remInt was in the tree, then remInt has been removed, bst_root // now points to the root of the new (smaller) binary search tree, // and the function returns true. Otherwise, if remInt was not in the // tree, then the tree is unchanged, and the function returns false. bool bst_remove(btNode* &bst_root, int insInt); // pre: bst_root is root pointer of a non-empty binary search tree // post: The largest item in the binary search tree has been removed, and // bst_root now points to the root of the new (smaller) binary search // tree. The reference parameter, removed, has been set to a copy of // the removed item. void bst_remove_max(btNode* &bst_root, int &data); // pre: // post: creates a node filled with the input data and right/left paths set to 0 btNode* create_node(int data); #endif
#ifndef BT_NODE_H
#define BT_NODE_H
struct btNode
{
int data;
btNode* left;
btNode* right;
};
// pre: bst_root is root pointer of a binary search tree (may be 0 for
// empty tree) and portArray has the base address of an array large
// enough to hold all the data items in the binary search tree
// post: The binary search tree has been traversed in-order and the data
// values are written (as they are encountered) to portArray in
// increasing positional order starting from the first element
void portToArrayInOrder(btNode* bst_root, int* portArray);
void portToArrayInOrderAux(btNode* bst_root, int* portArray, int& portIndex);
// pre: (none)
// post: dynamic memory of all the nodes of the tree rooted at root has been
// freed up (returned back to heap/freestore) and the tree is now empty
// (root pointer contains the null address)
void tree_clear(btNode*& root);
// pre: (none)
// post: # of nodes contained in tree rooted at root is returned
int bst_size(btNode* bst_root);
/////////////////////////////////////////////////////////////////////////////
// pre: bst_root is root pointer of a binary search tree (may be 0 for
// empty tree)
// post: If no node in the binary search tree has data equals insInt, a
// node with data insInt has been created and inserted at the proper
// location in the tree to maintain binary search tree property.
// If a node with data equals insInt is found, the node's data field
// has been overwritten with insInt; no new node has been created.
// (Latter case seems odd but it's to mimick update of key-associated
// data that exists in more general/real-world situations.)
void bst_insert(btNode* &bst_root, int insInt);
// pre: bst_root is root pointer of a binary search tree (may be 0 for
// empty tree)
// post: If remInt was in the tree, then remInt has been removed, bst_root
// now points to the root of the new (smaller) binary search tree,
// and the function returns true. Otherwise, if remInt was not in the
// tree, then the tree is unchanged, and the function returns false.
bool bst_remove(btNode* &bst_root, int insInt);
// pre: bst_root is root pointer of a non-empty binary search tree
// post: The largest item in the binary search tree has been removed, and
// bst_root now points to the root of the new (smaller) binary search
// tree. The reference parameter, removed, has been set to a copy of
// the removed item.
void bst_remove_max(btNode* &bst_root, int &data);
// pre:
// post: creates a node filled with the input data and right/left paths set to 0
btNode* create_node(int data);
#endif
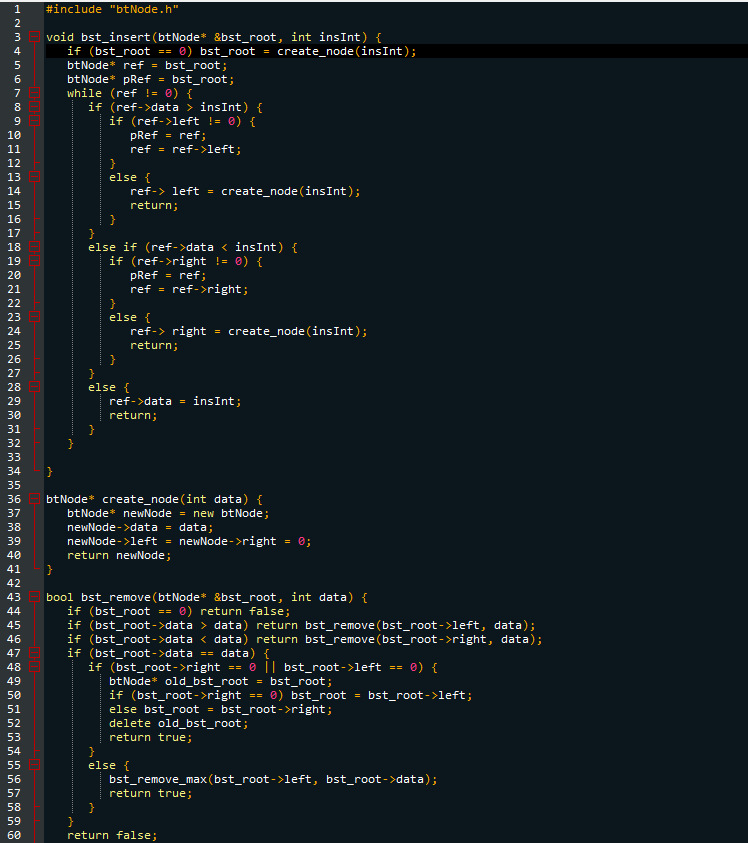
![4
14
24
C:\Users\reshm\OneDrive\Documents\btNo...
34 C:\Users\reshm\OneDrive\Documents\btNode.cpp
26 C:\Users\reshm\OneDrive\Documents\btNode.cpp
27 C:\Users\reshm\OneDrive\Documents\btNode.cpp
In function 'void bst_insert(btNode* &, int)':
[Error] 'create_node' was not declared in this scope
[Error] 'create_node' was not declared in this scope
[Error] 'create_node' was not declared in this scope](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faad9e4ed-ed50-4fe3-96ca-72ed3d364f6f%2Fb6b07ed1-b63c-46f0-a163-472188ea28cc%2Fzeprzyb_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps

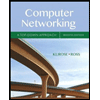
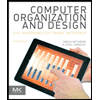
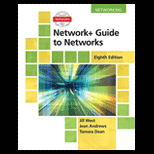
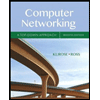
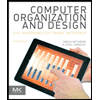
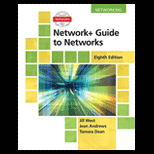
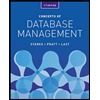
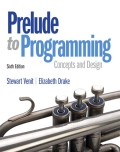
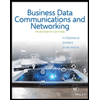