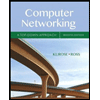
In C++ code:
Design and write a C++ class that reads text, binary and csv files. The class functions:
Size: Returns the file size
Name: Returns the file name
Raw: Returns the unparsed raw data
Parse: A external function to Parse the data. The function accepts the raw data and returns the data parsed by the function. This type of function is called a "Call-Back Function". A Call-Back is necessary for each file as each file requires different regular expressions to parse.
Call-back.cpp
#include <string>
#include <functional>
#include <iostream>
#include <vector>
using namespace std;
string ToLower(string s)
{
string temp;
for (char c : s)
temp.push_back(tolower(c));
temp.push_back(NULL);
return temp;
}
string ToUpper(string s)
{
string temp;
for (char c : s)
temp.push_back(toupper(c));
temp.push_back(NULL);
return temp;
}
struct Append
{
string operator()(string a, string b) // function object
{
return a + b;
}
};
class CallBack
{
function<string(string)> callback;
public:
CallBack(function<string(string)> cb) { callback = cb; }
string useCallBack(string s) { return callback(s); }
};
void embedd(string s)
{
CallBack cback(ToUpper);
cout << cback.useCallBack(s) << endl;
}
void functional()
{
string cat = "cat";
string dog = "DOG";
function<string(string)> fn1 = ToUpper; // ToLower; // function
function<string(string)> fn2 = &ToUpper; // function pointer
function<string(string, string)> fn3 = Append(); // function object
function<int(string)> fn4 = [](string s) { return s.length(); }; // lambda expression
function<int(int, int)> fn5 = plus<int>(); // standard function object
cout << fn1(dog) << endl;
cout << fn2(cat) << endl;
cout << fn3(cat, dog) << endl;
cout << fn4(dog) << endl;
cout << fn5(123, 456) << endl;
}
void main()
{
functional();
embedd("dog");
}

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps

If we need to put
If we need to put
- Create a structure called applianceTvpe with the following members. Use the proper variable TYPES for the members: name; SUpplierlD: modelNo: cost; Create an array variable of type applianceType that can hold 50 components. Write the code segment to read from a file into the structure array. Declare an input file stream identifier for this purpose. Open the file and read until end of file. Keep a count of how many rows actually read. The data file contains the following data: RefrigeratorABC 80 4657 521.62 ToasterOven 100 3245 245.96 MicroWave 95 7878 345.67 Using a looping construct, output to the console (cout) the data read into the structure array. Make sure you do not go outside the bounds of the array or beyond the number of rows actually read. You do not need to write any #include statements, using statements and you do not have to write code to open the file. Assume the file is open. You do need to declare variables needed for the program segment.arrow_forwardIn the C programming language, a pointer is used to store the ADDRESS of a data value. True Falsearrow_forwardHow do I do this C programming question?arrow_forward
- Computer Science csv file "/dsa/data/all_datasets/texas.csv" Task 6: Write a function "county_locator" that allows a user to enter in a name of a spatial data frame and a county name and have a map generated that shows the location of that county with respect to other counties (by using different colors and/or symbols). Hint: function(); $county ==; plot(); add=TRUE.arrow_forwardC++ formatting please Write a program (not a function) that reads from a file named "data.csv". This file consists of a list of countries and gold medals they have won in various Olympic events. Write to standard out, the top 5 countries with the most gold medals. You can assume there will be no ties. Expected Output: 1: United States with 1022 gold medals 2: Soviet Union with 440 gold medals 3: Germany with 275 gold medals 4: Great Britain with 263 gold medals 5: China with 227 gold medalsarrow_forwardGame of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forward
- What is the difference between the size and capacity of a vector?arrow_forwardstruct employee{int ID;char name[30];int age;float salary;}; (A) Using the given structure, Help me with a C program that asks for ten employees’ name, ID, age and salary from the user. Then, it writes the data in a file named out.txt (B) For the same structure, read the contents of the file out.txt and print the name of the highest salaried employee and youngest employee names name in the outputscreen.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
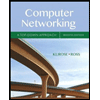
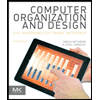
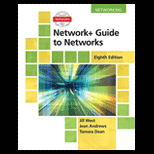
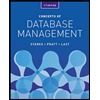
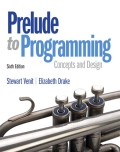
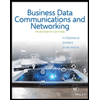