ifndef NODES_LLOLL_H #define NODES_LLOLL_H #include // for ostream namespace CS3358_SP2023_A5P2 { // child node struct CNode { int data; CNode* link; }; // parent node struct PNode { CNode* data;
#ifndef NODES_LLOLL_H
#define NODES_LLOLL_H
#include <iostream> // for ostream
namespace CS3358_SP2023_A5P2
{
// child node
struct CNode
{
int data;
CNode* link;
};
// parent node
struct PNode
{
CNode* data;
PNode* link;
};
// toolkit functions for LLoLL based on above node definitions
void Destroy_cList(CNode*& cListHead);
void Destroy_pList(PNode*& pListHead);
void ShowAll_DF(PNode* pListHead, std::ostream& outs);
void ShowAll_BF(PNode* pListHead, std::ostream& outs);
}
#endif
#include "nodes_LLoLL.h"
#include "cnPtrQueue.h"
#include <iostream>
using namespace std;
namespace CS3358_SP2023_A5P2
{
// do breadth-first (level) traversal and print data
void ShowAll_BF(PNode* pListHead, ostream& outs)
{
cnPtrQueue queue;
CNode* currentNode;
queue.push(pListHead->data);
while (!queue.empty())
{
currentNode = queue.front();
queue.pop();
if (currentNode->link != 0)
{
queue.push(currentNode->link);
}
if (currentNode->data != 0)
{
outs << currentNode->data->data << " ";
queue.push(currentNode->data);
}
}
outs << endl;
}
void Destroy_cList(CNode*& cListHead)
{
int count = 0;
CNode* cNodePtr = cListHead;
while (cListHead != 0)
{
cListHead = cListHead->link;
delete cNodePtr;
cNodePtr = cListHead;
++count;
}
cout << "Dynamic memory for " << count << " CNodes freed"
<< endl;
}
void Destroy_pList(PNode*& pListHead)
{
int count = 0;
PNode* pNodePtr = pListHead;
while (pListHead != 0)
{
pListHead = pListHead->link;
Destroy_cList(pNodePtr->data);
delete pNodePtr;
pNodePtr = pListHead;
++count;
}
cout << "Dynamic memory for " << count << " PNodes freed"
<< endl;
}
// do depth-first traversal and print data
void ShowAll_DF(PNode* pListHead, ostream& outs)
{
while (pListHead != 0)
{
CNode* cListHead = pListHead->data;
while (cListHead != 0)
{
outs << cListHead->data << " ";
cListHead = cListHead->link;
}
pListHead = pListHead->link;
}
}
}
![>sh -c make -s
./nodes_LLOLL.cpp:28:40: error: member reference type 'int' is not a pointer
outs <<< currentNode->data->data << " ";
INNNN
./nodes_LLOLL.cpp:29:24: error: cannot initialize a parameter of type 'CS3358_SP2023_A5P2::CNode *
with an lvalue of type 'int'
NN
queue.push(currentNode->data);
^NNNNNN
INNNN
./cnptrQueue.h:18:24: note: passing argument to parameter 'cnPtr' here
void push(CNode* cnptr);
2 errors generated.
make: *** [Makefile:10: main] Error 1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa3dbb91f-777d-47b2-aa94-40b3e17142a5%2F83b89c8d-371d-4302-bfa7-d15c9bc08805%2Fqfc1xta_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

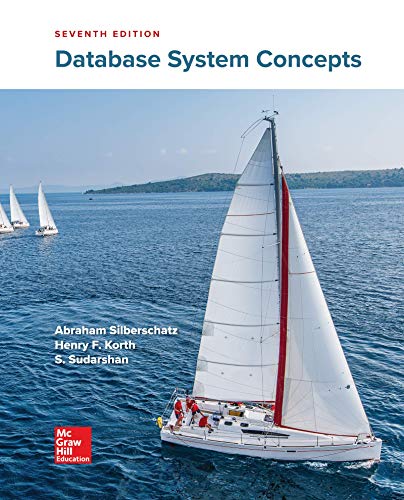
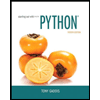
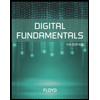
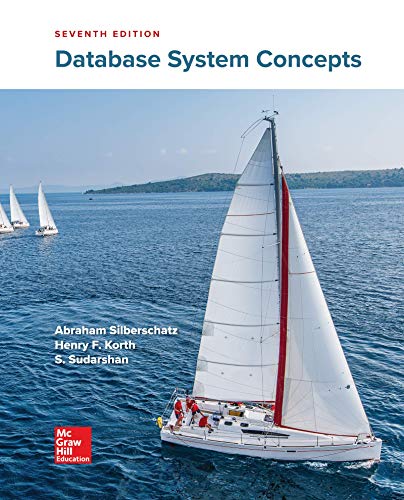
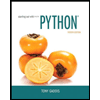
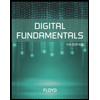
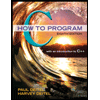
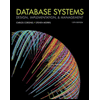
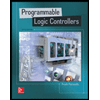