I'm trying to delete a node after inputting data for 2-3 checks but the node I try to delete remains in the list. How do I fix my deleteCheck() function to run correctly with the rest of my code? Thank you. #include <stdio.h>#include <stdlib.h>// Austin Chong// The core concept of this assignment is to use a linked list in a C program.// 07 March 2020 struct checkNode{int checkNumber;char date[8];char paidTo[100];char description[100];double amount;struct checkNode *head;struct checkNode *next;}; struct checkNode *checkBook = NULL; /* Functions */ void addCheck(){int checkNumber;double amount;char temp;struct checkNode *newCheck = (struct checkNode*) malloc(sizeof(struct checkNode));printf("Please enter the check number: ");scanf("%d", &checkNumber);newCheck -> checkNumber = checkNumber;printf("Please enter the amount: ");scanf("%lf", &amount);newCheck -> amount = amount;printf("Please enter the date: ");scanf("%c", &temp);scanf("%[^\n]", newCheck -> date);printf("Please enter the person receiving the check: ");scanf("%c", &temp);scanf("%[^\n]", newCheck -> paidTo);printf("Enter a description for the check: ");scanf("%c", &temp);scanf("%[^\n]", newCheck -> description);if(checkBook == NULL){checkBook = newCheck;checkBook -> head = newCheck;newCheck -> next = NULL;} else {struct checkNode *tNode;tNode = checkBook -> head;while(tNode -> next != NULL){tNode = tNode -> next;}tNode -> next = newCheck;}printf("\nNew check added successfully!\n");} void deleteCheck(){int deletedCheck;if(checkBook == NULL){printf("\nThe checkbook is currently empty.\n");return;}struct checkNode *temp;temp = checkBook -> head;if(checkBook != NULL){printf("Please enter which check number you would like to delete: ");scanf("%d", &deletedCheck);if(temp -> checkNumber == deletedCheck) {checkBook -> head = temp -> next;free(temp);}}} void displayCheck(){int count = 0;if(checkBook == NULL){printf("There are no checks to display.\n");return;}printf("Please enter the check number: ");scanf("%d", &count);struct checkNode *temp;temp = checkBook -> head;while(temp != NULL){if(temp -> checkNumber == count){printf("The amount listed on the check is: %lf\n", temp -> amount);printf("The date on this check is: %s\n", temp -> date);printf("The person that received this check is: %s\n", temp -> paidTo);printf("The description listed for this check is: %s\n", temp -> description);return;} else {temp = temp -> next;}}} void displayAll(){struct checkNode *temp;temp = checkBook -> head;if(temp == NULL){printf("There are no checks to display.\n");return;} else {while(temp != NULL){printf("The information of check #%d is:\n", temp -> checkNumber);printf("Amount: %lf\n", temp -> amount);printf("Date: %s\n", temp -> date);printf("Person Received: %s\n", temp -> paidTo);printf("Description: %s\n\n", temp -> description);temp = temp -> next;}}} int main(){int option;while(1){printf("\n1. Add a check.\n");printf("2. Display single check.\n");printf("3. Display all checks.\n");printf("4. Delete a check.\n");printf("5. Quit.\n");scanf("%d", &option);switch(option){case 1:addCheck();break;case 2:displayCheck();break;case 3:displayAll();break;case 4:deleteCheck();break;case 5:printf("Good bye!\n");exit(0);default:printf("Choose a different option.\n");}}}
I'm trying to delete a node after inputting data for 2-3 checks but the node I try to delete remains in the list. How do I fix my deleteCheck() function to run correctly with the rest of my code? Thank you.
#include <stdio.h>
#include <stdlib.h>
// Austin Chong
// The core concept of this assignment is to use a linked list in a C program.
// 07 March 2020
struct checkNode
{
int checkNumber;
char date[8];
char paidTo[100];
char description[100];
double amount;
struct checkNode *head;
struct checkNode *next;
};
struct checkNode *checkBook = NULL;
/* Functions */
void addCheck()
{
int checkNumber;
double amount;
char temp;
struct checkNode *newCheck = (struct checkNode*) malloc(sizeof(struct checkNode));
printf("Please enter the check number: ");
scanf("%d", &checkNumber);
newCheck -> checkNumber = checkNumber;
printf("Please enter the amount: ");
scanf("%lf", &amount);
newCheck -> amount = amount;
printf("Please enter the date: ");
scanf("%c", &temp);
scanf("%[^\n]", newCheck -> date);
printf("Please enter the person receiving the check: ");
scanf("%c", &temp);
scanf("%[^\n]", newCheck -> paidTo);
printf("Enter a description for the check: ");
scanf("%c", &temp);
scanf("%[^\n]", newCheck -> description);
if(checkBook == NULL)
{
checkBook = newCheck;
checkBook -> head = newCheck;
newCheck -> next = NULL;
} else {
struct checkNode *tNode;
tNode = checkBook -> head;
while(tNode -> next != NULL)
{
tNode = tNode -> next;
}
tNode -> next = newCheck;
}
printf("\nNew check added successfully!\n");
}
void deleteCheck()
{
int deletedCheck;
if(checkBook == NULL)
{
printf("\nThe checkbook is currently empty.\n");
return;
}
struct checkNode *temp;
temp = checkBook -> head;
if(checkBook != NULL)
{
printf("Please enter which check number you would like to delete: ");
scanf("%d", &deletedCheck);
if(temp -> checkNumber == deletedCheck) {
checkBook -> head = temp -> next;
free(temp);
}
}
}
void displayCheck()
{
int count = 0;
if(checkBook == NULL)
{
printf("There are no checks to display.\n");
return;
}
printf("Please enter the check number: ");
scanf("%d", &count);
struct checkNode *temp;
temp = checkBook -> head;
while(temp != NULL)
{
if(temp -> checkNumber == count)
{
printf("The amount listed on the check is: %lf\n", temp -> amount);
printf("The date on this check is: %s\n", temp -> date);
printf("The person that received this check is: %s\n", temp -> paidTo);
printf("The description listed for this check is: %s\n", temp -> description);
return;
} else {
temp = temp -> next;
}
}
}
void displayAll()
{
struct checkNode *temp;
temp = checkBook -> head;
if(temp == NULL)
{
printf("There are no checks to display.\n");
return;
} else {
while(temp != NULL)
{
printf("The information of check #%d is:\n", temp -> checkNumber);
printf("Amount: %lf\n", temp -> amount);
printf("Date: %s\n", temp -> date);
printf("Person Received: %s\n", temp -> paidTo);
printf("Description: %s\n\n", temp -> description);
temp = temp -> next;
}
}
}
int main()
{
int option;
while(1)
{
printf("\n1. Add a check.\n");
printf("2. Display single check.\n");
printf("3. Display all checks.\n");
printf("4. Delete a check.\n");
printf("5. Quit.\n");
scanf("%d", &option);
switch(option)
{
case 1:
addCheck();
break;
case 2:
displayCheck();
break;
case 3:
displayAll();
break;
case 4:
deleteCheck();
break;
case 5:
printf("Good bye!\n");
exit(0);
default:
printf("Choose a different option.\n");
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

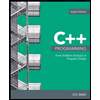
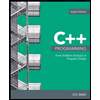