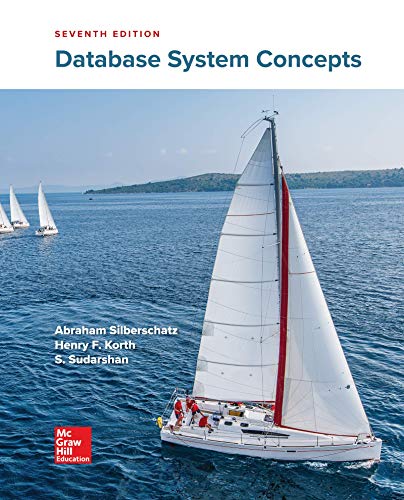
Question : Write a c++ program which creates a Linked List having maximum sum out of two Sorted Linked Lists.
Use this : LinkedList finalMaxSumList(LinkedList List1, LinkedList List2);
For eg: You have two sorted linked lists, create a linked list that contains data with maximum sum. The resulting list with maximum sum will contain nodes with data from both input sorted lists. While creating the resulting list with maximum sum, we may switch to the other input list only at the point of intersection (which mean the two nodes with the same value in the lists). You are only allowed to use O(1) extra space.
Note: You have to returned Linked List which contain finalMaxSumList and you are not allowed to change in data type and prototype of the Function.
Input:
List1 = 1->3->30->90->120->240->511
List2 = 0->3->12->32->90->125->240->249
Returned LinkedList:
Following is resulting linked list out of two sorted lists
ResultingList = 1->3->12->32->90->125->240->511

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- 5. The following function indexCounter aims to find and return all the indices of the element x in a list L. if x is not in the list L, return an empty list. However, the implementation has a flaw, so that many times it will return error message. Can you fix the flaw? def indexCounter(L, x): indexList [] startIndex = 0 while startIndex < len(L): indexList.append (L.index (x, startIndex)) startIndex = L.index (x, startIndex) + 1 return indexListarrow_forwardThink about the following example: A computer program builds and modifies a linked list like follows: Normally, the program would keep tabs on two unique nodes, which are as follows: An explanation of how to use the null reference in the linked list's node in two common circumstancesarrow_forwardC++ program for the following please: Create a generic function print(ls, n) that prints to standardoutput the first n elements of list ls. The elements are printed on separatelines. If ls has less than n elements, then the entire list is printedarrow_forward
- Create a program in C++ using a character linked list. It will insert the letters into the list alphabetically. Ask the user to enter a word. Then, enter the word into the list. Then, print out what is in the list, and clear it out. example: enters: apple prints: aelpp You can do this by writing your own linked list (extra credit), or using the STL list class. -- if you are using the STL list class, do not use the “sort” function.arrow_forwardYou may do this assignment using either Java or C++. Do *not use the JDK LinkedList class or any linked list library! A linked list consists of zero or more nodes, which each contain one item of data and a link (Java reference, C++ pointer or reference) to the next node, if there is one. Linked List code is usually generic, so that you can use it to create a list of Strings, Students, Doubles, etc. For this assignment, you will write a simplified version of linked list that only can be used to create and use a list of ints and that has only a few of the functions typical of linked lists. The data element can be just an int, and the data type of the link to the next node can be just Node. You will need a reference (Java) or either a reference or a pointer (C++) to the first node, as well as one to the last node. Write a method/function that takes an in, creates a node with that int as its data value, and adds the node to the end of the list. This function will need to update the…arrow_forwardin #lang schemearrow_forward
- Write a program in Java to manipulate a Singly Linked List: Count the number of nodes Insert a new node before the value 5 of Singly Linked List Search an existing element in a Singly linked list (the element of search is given by the user) Suppose List contained the following Test Data: Input data for node 1: 2Input data for node 2 : 3Input data for node 3 : 5 Input data for node 4: 8arrow_forwardWrite a program in three parts. The first part should create a linked list of 26 nodes. The second part should fill the list with the letters of the alphabet. The third part should print the contents of the linked list. NOT USE malloc, only use <iostream>. Do not use global variables. The use of global constants c++arrow_forwardUsing c++ Contact list: Binary Search A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Frank 867-5309 Joe…arrow_forward
- Provide full C++ code Building a playlist (of songs) using a linked list and making some operations such as adding songs, removing songs, shuffling them, etc. (Some parts of this lab will closely follow lab 08 - it is imperative that you complete that lab before working on this project). A Node representing a song has the following data members (similar to struct Node of lab 08, except it doesn't have one member for storing data): string uniqueID string songName string artistName int songLength Node* nextNodePtr Your code must have the following three files: Playlist.h - Class declaration for a linked list of Nodes (very similar to lab 08 but with some changes to methods, as described below) with following private data members: private:Node* first;Node* last;int size; Playlist.cpp - Class definition main.cpp - main() function Checkpoint A For checkpoint A, you need to build a playlist (of songs) by readings data members of several songs from a file and printing them. Implement…arrow_forwardWhich of the following statements regarding linked lists and arrays is correct? Group of answer choices: a. A dynamically resizable array makes insertions at the beginning of an array very efficient. b. Using a doubly linked list is more efficient than an array when accessing the ith element of a structure. c. A dynamically resizable array is another name for a linked list. d. Any type of list tends to be better than an array when there are a set number of maximum items known beforehand. e. If many deletions at any place in the structure were common, a linked list implementation would likely be preferable to an arrayarrow_forwardPlease help with C++Priority Queue question in C++ language. Sample output also in image. Thanks.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
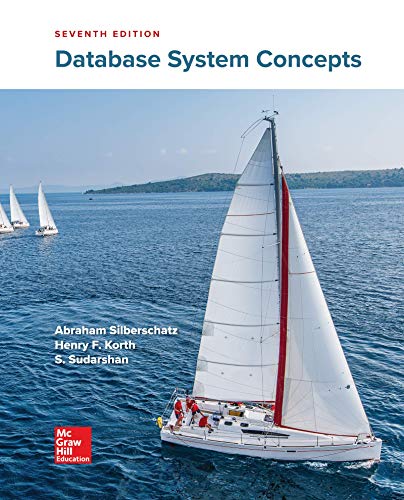
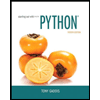
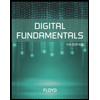
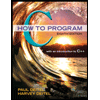
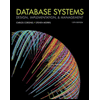
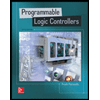