Implement a class Balloon that models a spherical balloon that is being filled with air. The constructor constructs an empty balloon, and other methods add air, and get the volume, surface area, and radius. Use this template: Balloon.java Example: Balloon balloon = new Balloon (); balloon.getVolume (); // returns 0.0 balloon.getSurfaceArea (); // returns 0.0 balloon.getRadius (); // returns 0.0 balloon.addAir(100); balloon.getVolume () ; // returns 100.0 balloon.getSurfaceArea (); // returns 104.187942 balloon.getRadius () ; // returns 2.879412 balloon.addAir (100); balloon.getVolume () ; // returns 200.0 balloon.getSurfaceArea (); // returns 165.388048 balloon.getRadius (); // returns 3.627832
Implement a class Balloon that models a spherical balloon that is being filled with air. The constructor constructs an empty balloon, and other methods add air, and get the volume, surface area, and radius. Use this template: Balloon.java Example: Balloon balloon = new Balloon (); balloon.getVolume (); // returns 0.0 balloon.getSurfaceArea (); // returns 0.0 balloon.getRadius (); // returns 0.0 balloon.addAir(100); balloon.getVolume () ; // returns 100.0 balloon.getSurfaceArea (); // returns 104.187942 balloon.getRadius () ; // returns 2.879412 balloon.addAir (100); balloon.getVolume () ; // returns 200.0 balloon.getSurfaceArea (); // returns 165.388048 balloon.getRadius (); // returns 3.627832
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Java. Please use the template, thank you!
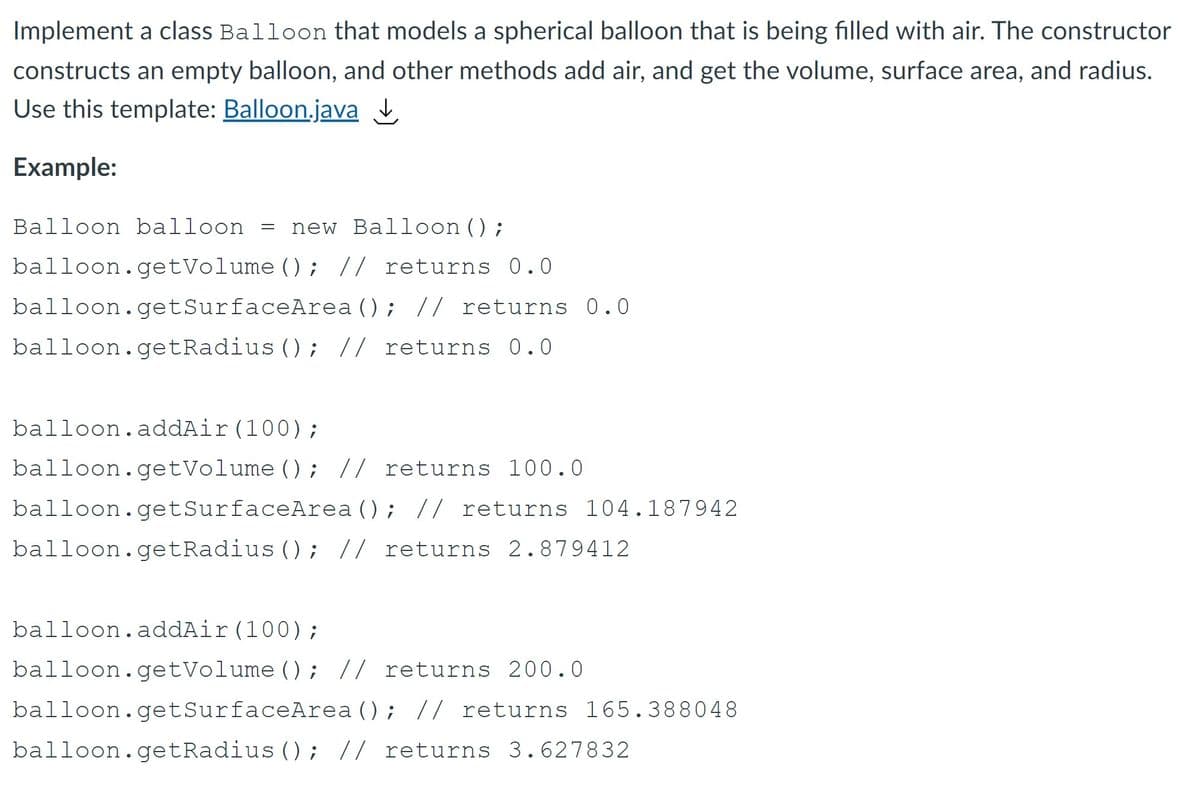
Transcribed Image Text:Implement a class Balloon that models a spherical balloon that is being filled with air. The constructor
constructs an empty balloon, and other methods add air, and get the volume, surface area, and radius.
Use this template: Balloon.java
Example:
Balloon balloon =
new Balloon();
balloon.getVolume (); // returns 0.0
balloon.getSurfaceArea (); // returns 0.0
balloon.getRadius () ; // returns 0.0
balloon.addAir(100);
balloon.getVolume () ; // returns 100.0
balloon.getSurfaceArea (); // returns 104.187942
balloon.getRadius (); // returns 2.879412
balloon.addAir(100);
balloon.getVolume (); // returns 200.0
balloon.getSurfaceArea (); // returns 165.388048
balloon.getRadius (); // returns 3.627832
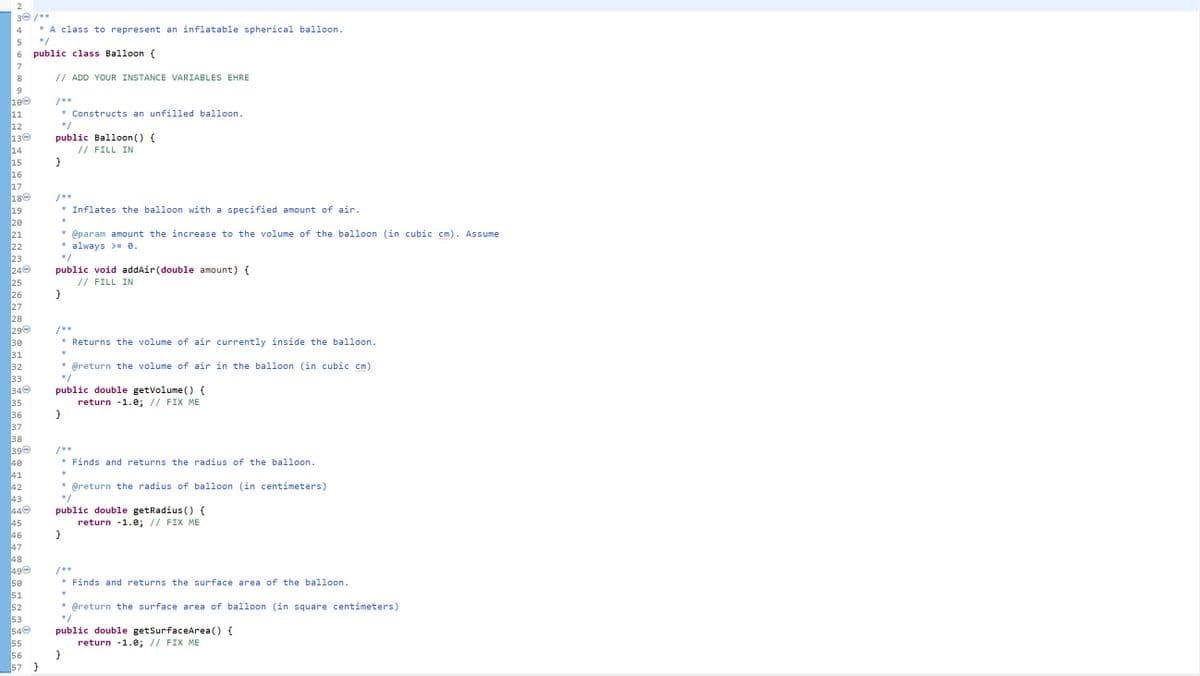
Transcribed Image Text:30 /**
* A class to represent an inflatable spherical balloon.
4
* /
6.
public class Balloon {
7
8.
// ADD YOUR INSTANCE VARIABLES EHRE
9.
100
11
12
130
/ **
* Constructs an unfilled balloon.
*/
public Balloon() {
// FILL IN
}
14
15
16
17
180
/**
19
* Inflates the balloon with a specified amount of air.
20
21
22
* @param amount the increase to the volume of the balloon (in cubic cm). Assume
* always >= 0.
23
240
25
26
*/
public void addAir(double amount) {
// FILL IN
}
27
28
290
/**
30
* Returns the volume of air currently inside the balloon.
31
32
33
340
@return the volume of air in the balloon (in cubic cm)
*
*/
public double getVolume () {
return -1.0; // FIX ME
35
36
37
38
390
40
/**
* Finds and returns the radius of the balloon.
41
* @return the radius of balloon (in centimeters)
42
43
440
45
46
47
48
490
*/
public double getRadius () {
return -1.0; // FIX ME
}
/**
* Finds and returns the surface area of the balloon.
50
51
52
53
540
* @return the surface area of balloon (in square centimeters)
* /
public double getSurfaceArea() {
return -1.0; // FIX ME
}
55
56
57 }
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Recommended textbooks for you
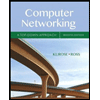
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
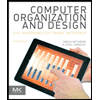
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
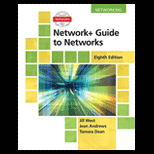
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
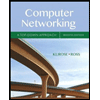
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
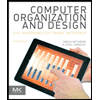
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
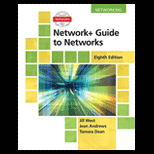
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
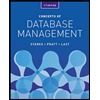
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
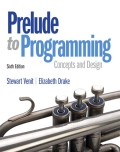
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
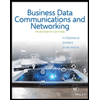
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY