Write a java class called House with the following two private attributes, and three methods: Attributes: 1) type (String) 2) height (int) The valid values of attribute "type" are: "Cabin", "Bungalow", and "High Rise". A house can be upgraded to the next type. For example, a house of type "Cabin" can be upgraded to "Bungalow" which can be later upgraded to "High Rise". A house is never downgraded. Methods:
Write a java class called House with the following two private attributes, and three methods: Attributes: 1) type (String) 2) height (int) The valid values of attribute "type" are: "Cabin", "Bungalow", and "High Rise". A house can be upgraded to the next type. For example, a house of type "Cabin" can be upgraded to "Bungalow" which can be later upgraded to "High Rise". A house is never downgraded. Methods:
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter10: Classes And Data Abstraction
Section: Chapter Questions
Problem 17PE
Related questions
Question
The
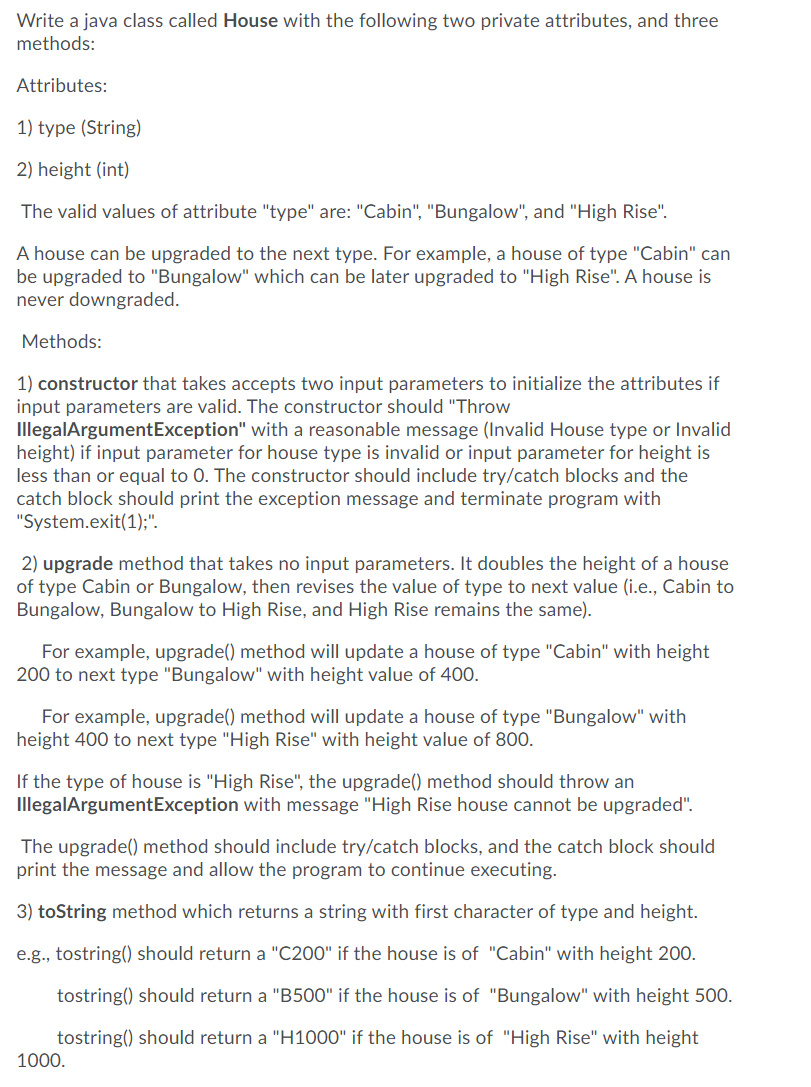
Transcribed Image Text:Write a java class called House with the following two private attributes, and three
methods:
Attributes:
1) type (String)
2) height (int)
The valid values of attribute "type" are: "Cabin", "Bungalow", and "High Rise".
A house can be upgraded to the next type. For example, a house of type "Cabin" can
be upgraded to "Bungalow" which can be later upgraded to "High Rise". A house is
never downgraded.
Methods:
1) constructor that takes accepts two input parameters to initialize the attributes if
input parameters are valid. The constructor should "Throw
IllegalArgumentException" with a reasonable message (Invalid House type or Invalid
height) if input parameter for house type is invalid or input parameter for height is
less than or equal to 0. The constructor should include try/catch blocks and the
catch block should print the exception message and terminate program with
"System.exit(1);".
2) upgrade method that takes no input parameters. It doubles the height of a house
of type Cabin or Bungalow, then revises the value of type to next value (i.e., Cabin to
Bungalow, Bungalow to High Rise, and High Rise remains the same).
For example, upgrade() method will update a house of type "Cabin" with height
200 to next type "Bungalow" with height value of 400.
For example, upgrade() method will update a house of type "Bungalow" with
height 400 to next type "High Rise" with height value of 800.
If the type of house is "High Rise", the upgrade() method should throw an
IllegalArgumentException with message "High Rise house cannot be upgraded".
The upgrade() method should include try/catch blocks, and the catch block should
print the message and allow the program to continue executing.
3) toString method which returns a string with first character of type and height.
e.g., tostring() should return a "C200" if the house is of "Cabin" with height 200.
tostring() should return a "B500" if the house is of "Bungalow" with height 500.
tostring() should return a "H1000" if the house is of "High Rise" with height
1000.
![Map Class
Create a class called Map that uses the class House which was developed in
question 4.
The Map class should include the following two private attributes:
1) private static House []] houses; // (as 2D array of houses of size MxN.
Example: 8x7 array would be declared as House [8][7].
3x4 array would be declared as House[3][4], etc.
2) private static final String FILE_NAME = "house.txt";
Write the following methods. You must determine if they should be private/public
and static/non-static:
1) loadHouses() method that takes no input. This method should initialize the houses
attribute by putting the house data into the attribute. The data should be loaded
from the text file which is given in the FILE_NAME attribute. The data format in the
house.txt is described as follows:
house.txt file contents are as follows data lines:
Cabin:5, Cabin:1, Bungalow:9, High Rise:11
Cabin:2, Cabin:4, Bungalow:8, Bungalow:10
High Rise:13, Cabin:3, Bungalow:6, Bungalow:7
High Rise:15, High Rise:14, High Rise:12, High Rise:16
After calling loadHouses(), the houses are loaded in the 2D array, such that
System.out.println(Arrays.deepToString(houses));
should print the following:
[[C5, С1, В9, Н11]), [C2, С4, В8, В10], [Н13, СЗ, В6, B7]), [Н15, Н14, Н12, Н16]]
2) A method upgradeHouses that takes two integers (first being row, and second
being column) of houses. The entire row and column should be upgraded (each house
by only once).
Example:
Before calling upgradeHouses method if
System.out.println(Arrays.deepToString(houses));
prints the following:
[[C5, С1, В9, Н11]), [C2, С4, В8, В10], [Н13, СЗ, В6, B7]), [Н15, Н14, Н12, Н16]]
after calling upgradeHouses(1,2) method, the
System.out.printIn(Arrays.deepToString(houses));
Should print the following:
[[C5, C1, H18, H11], [B4, B8, H16, H20], [H13, C3, H12, B7], [H15, H14, H12, H16]]
3) A main method with the following statements:
• all Map.loadHouses() method.
• print the houses array with:
System.out.println(Arrays.deepToString(houses);
• call upgradeHouses(1, 2);
• print the houses array with:
System.out.println(Arrays.deepToString(houses));](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F67734724-3d19-4781-a28f-91103c5f77a4%2Fb18fb200-dd15-4063-8312-a4c75ac56453%2Fjwdaqv_processed.png&w=3840&q=75)
Transcribed Image Text:Map Class
Create a class called Map that uses the class House which was developed in
question 4.
The Map class should include the following two private attributes:
1) private static House []] houses; // (as 2D array of houses of size MxN.
Example: 8x7 array would be declared as House [8][7].
3x4 array would be declared as House[3][4], etc.
2) private static final String FILE_NAME = "house.txt";
Write the following methods. You must determine if they should be private/public
and static/non-static:
1) loadHouses() method that takes no input. This method should initialize the houses
attribute by putting the house data into the attribute. The data should be loaded
from the text file which is given in the FILE_NAME attribute. The data format in the
house.txt is described as follows:
house.txt file contents are as follows data lines:
Cabin:5, Cabin:1, Bungalow:9, High Rise:11
Cabin:2, Cabin:4, Bungalow:8, Bungalow:10
High Rise:13, Cabin:3, Bungalow:6, Bungalow:7
High Rise:15, High Rise:14, High Rise:12, High Rise:16
After calling loadHouses(), the houses are loaded in the 2D array, such that
System.out.println(Arrays.deepToString(houses));
should print the following:
[[C5, С1, В9, Н11]), [C2, С4, В8, В10], [Н13, СЗ, В6, B7]), [Н15, Н14, Н12, Н16]]
2) A method upgradeHouses that takes two integers (first being row, and second
being column) of houses. The entire row and column should be upgraded (each house
by only once).
Example:
Before calling upgradeHouses method if
System.out.println(Arrays.deepToString(houses));
prints the following:
[[C5, С1, В9, Н11]), [C2, С4, В8, В10], [Н13, СЗ, В6, B7]), [Н15, Н14, Н12, Н16]]
after calling upgradeHouses(1,2) method, the
System.out.printIn(Arrays.deepToString(houses));
Should print the following:
[[C5, C1, H18, H11], [B4, B8, H16, H20], [H13, C3, H12, B7], [H15, H14, H12, H16]]
3) A main method with the following statements:
• all Map.loadHouses() method.
• print the houses array with:
System.out.println(Arrays.deepToString(houses);
• call upgradeHouses(1, 2);
• print the houses array with:
System.out.println(Arrays.deepToString(houses));
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
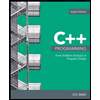
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
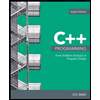
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning