Implement a class Quadrilateral that represents a quadrilateral shape, based on the coordinates of its four corners. The getShapeType method returns a string that describes the quadrilateral's shape. Use this template: Quadrilateral.java
Implement a class Quadrilateral that represents a quadrilateral shape, based on the coordinates of its four corners. The getShapeType method returns a string that describes the quadrilateral's shape. Use this template: Quadrilateral.java
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Java. Please use the template, thank you!
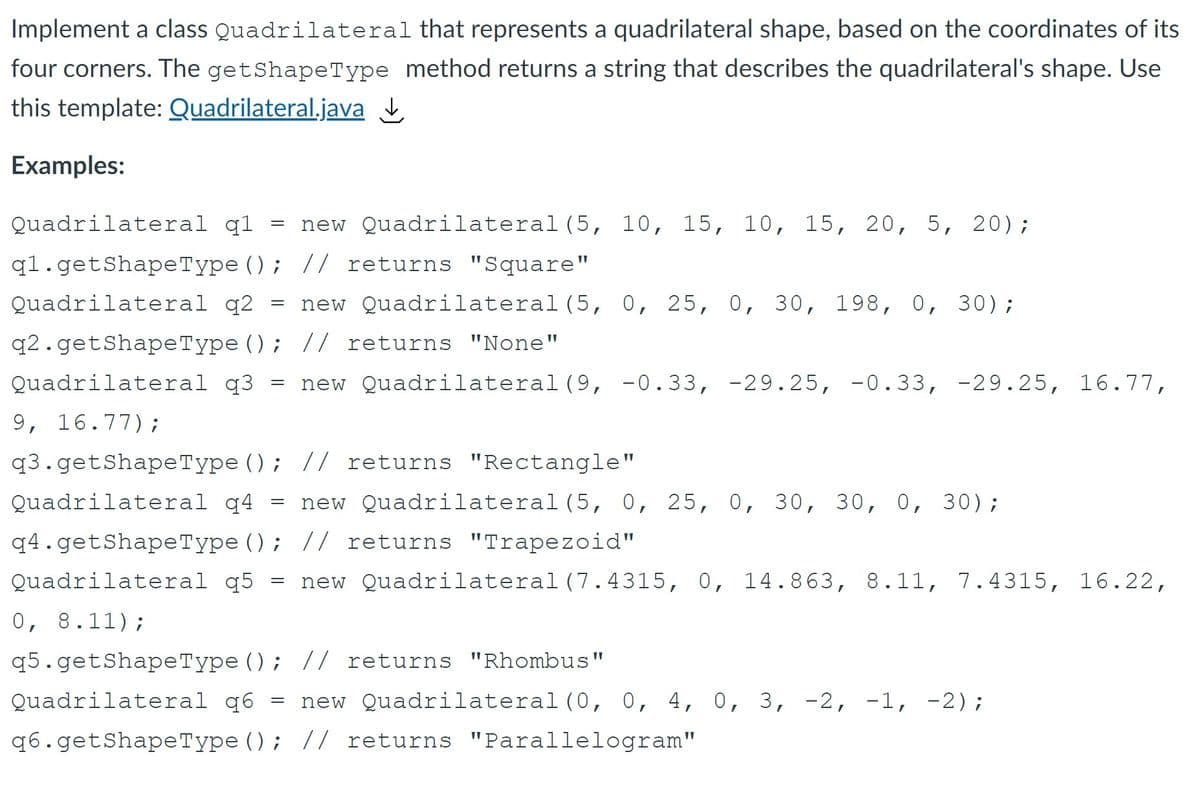
Transcribed Image Text:Implement a class Quadrilateral that represents a quadrilateral shape, based on the coordinates of its
four corners. The getShapeType method returns a string that describes the quadrilateral's shape. Use
this template: Quadrilateral.java
Examples:
Quadrilateral q1
new Quadrilateral (5, 10, 15, 10, 15, 20, 5, 20);
q1.getShapeType (); // returns "Square"
Quadrilateral q2 =
new Quadrilateral(5, 0, 25, 0, 30, 198, 0, 30);
q2.getShapeType (); // returns "None"
Quadrilateral q3 = new Quadrilateral (9, -0.33, -29.25, -0.33, -29.25, 16.77,
9, 16.77);
q3.getShapeType (); // returns "Rectangle"
Quadrilateral q4 = new Quadrilateral (5, 0, 25, 0, 30, 30, 0, 30);
q4.getShapeType (); // returns "Trapezoid"
Quadrilateral q5
new Quadrilateral(7.4315, 0, 14.863, 8.11, 7.4315, 16.22,
=
0, 8.11);
q5.getShapeType (); // returns "Rhombus"
Quadrilateral q6 = new Quadrilateral (0, 0, 4, 0, 3, -2, -1, -2);
q6.getShapeType (); // returns "Parallelogram"
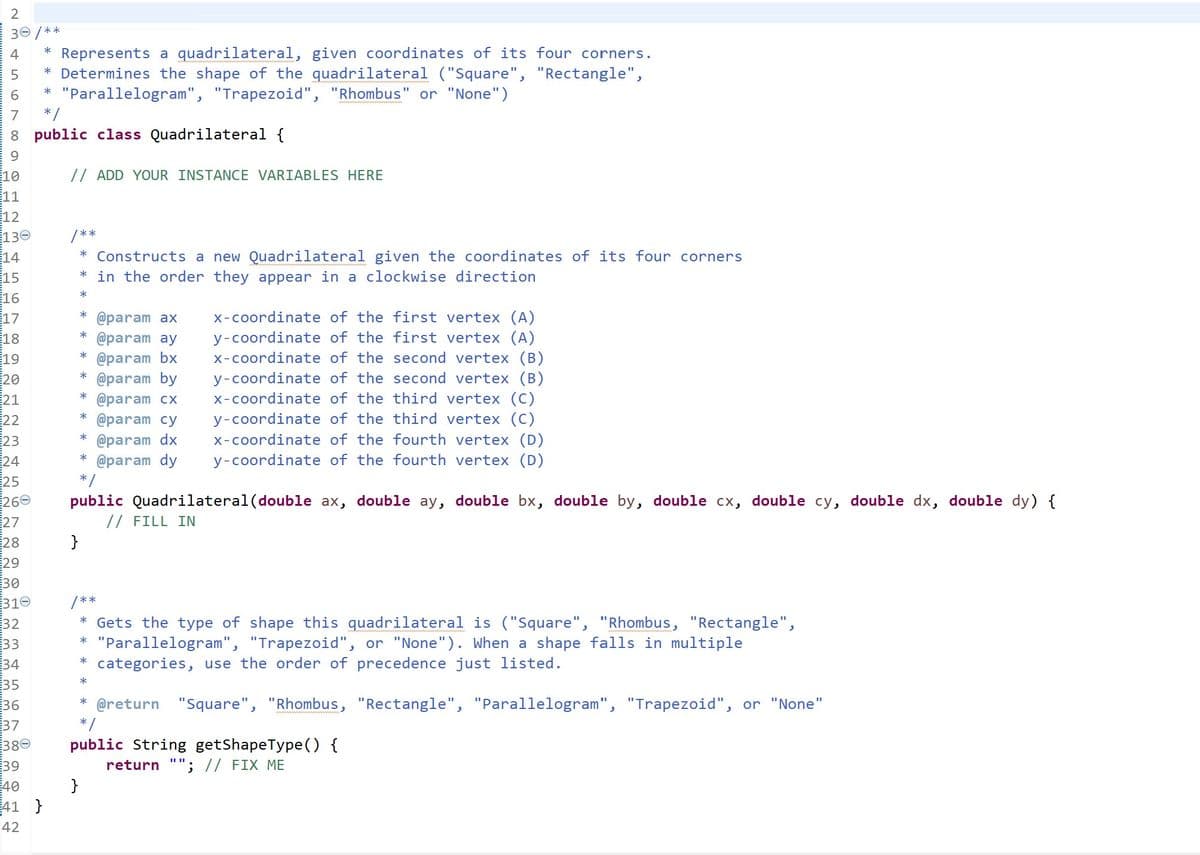
Transcribed Image Text:2
30 / **
Represents a quadrilateral, given coordinates of its four corners.
* Determines the shape of the quadrilateral ("Square", "Rectangle",
* "Parallelogram", "Trapezoid", "Rhombus" or "None")
* /
*
4
5
7
8 public class Quadrilateral {
10
// ADD YOUR INSTANCE VARIABLES HERE
11
12
130
14
/**
Constructs a new Quadrilateral given the coordinates of its four corners
* in the order they appear in a clockwise direction
*
15
16
*
X-coordinate of the first vertex (A)
y-coordinate of the first vertex (A)
X-coordinate of the second vertex (B)
y-coordinate of the second vertex (B)
X-coordinate of the third vertex (C)
y-coordinate of the third vertex (C)
X-coordinate of the fourth vertex (D)
y-coordinate of the fourth vertex (D)
*
17
18
@param ax
@param ay
*
@param bx
@param by
@param cx
@param cy
*
19
20
*
21
*
22
@param dx
* @param dy
*
23
24
25
*/
public Quadrilateral (double ax, double ay, double bx, double by, double cx, double cy, double dx, double dy) {
// FILL IN
}
260
27
28
29
30
310
32
33
34
/**
* Gets the type of shape this quadrilateral is ("Square", "Rhombus, "Rectangle",
* "Parallelogram", "Trapezoid", or "None"). When
categories, use the order of precedence just listed.
shape falls in multiple
*
*
35
"Square",
*
"Rhombus, "Rectangle", "Parallelogram", "Trapezoid", or "None"
@return
* /
36
37
380
39
public String getShapeType() {
return ""; // FIX ME
}
40
41 }
42
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
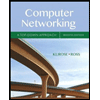
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
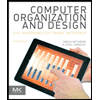
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
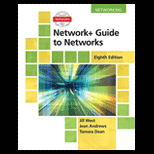
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
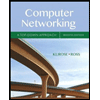
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
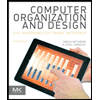
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
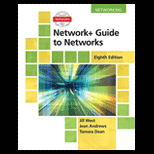
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
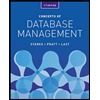
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
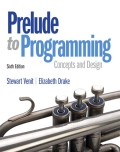
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
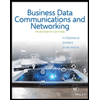
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY