Implement a function create_grade_list() with a string parameter representing space-separated grades, e.g., "90 87 99". In this function, you need to split the string and ensure that all grades in this string are numeric. In case of a non-numeric grade, e.g., "A A B-", return -1. If all grades are numeric, return a list of these grades. All grades in this list should be of type float.
Instructions
Assuming you are registered in a class with several activities/categories (quizzes, labs, homework, attendance, etc.), you would like to keep track of your grades throughout the quarter. For each category, you need to add your grade, e.g., for the labs you got 100 in lab 1, 95 in lab 2, etc.
How are we going to store our grades for each category?
Remember that in our main program, we keep the information for various grade categories. This information is stored as a list of lists, which can, for example, look like this:
[["Lab", 35], ["Quiz", 35]]
Each list that is contained in the main “
If for the labs, you got 100 in lab 1, 95 in lab 2, we can store this information for the “Lab” category as an additional list:
["Lab", 35, [100, 95]]
This means that our database would then look like this (note the nesting of the grades):
[["Lab", 35, [100, 95]], ["Quiz", 35]]
In order to add the grades to a category, we will have interactions similar to updating a category.
Requirements
Implement a function create_grade_list() with a string parameter representing space-separated grades, e.g., "90 87 99". In this function, you need to split the string and ensure that all grades in this string are numeric. In case of a non-numeric grade, e.g., "A A B-", return -1. If all grades are numeric, return a list of these grades. All grades in this list should be of type float.
def create_grade_list(grade_info): """ grade_info : a string of grades, space separated, e.g., "90 98 88" return: -1 : if any grade in the string is not numeric Otherwise, return a list of float grades """ pass
In the main program (the grade management system defined in the breakout room lab), add the following lines to prompt the user for the grade information when they select a category to which to add them. After the user enters the correct information, the program displays the category information, together with the list of grades. Note that the structure is very similar to the “Update category”.
We encourage you to carefully review the program code provided for you and ask us questions about it. For the final project, you will be required to create your own main program interaction code.
elif opt == …: # add the appropriate option key from the menu print_categories(all_categories) print("To which category would you like to add grades?") print("Enter the number corresponding to the category.") user_option = input() if not user_option.isdigit(): print(f"WARNING: `{user_option}` is an invalid category number!") else: idx = int(user_option) - 1 if not is_valid_index(idx, all_categories): print(f"WARNING: `{user_option}` is an invalid category number!") else: print(f"Adding grades to category {all_categories[idx][0]}") print("Enter the numeric grades separated by spaces: ") grade_info = input() grade_list = create_grade_list(grade_info) if type(grade_list) == list: if len(all_categories[idx]) == 2: # if no grades for this category have been added print("Adding new grades") all_categories[idx].append(grade_list) else: # there are already grades stored, add the new ones print("Updating the grades") all_categories[idx][2] += grade_list print("-" * 45) print("Category:", ...) # TODO: print the correct variables print("Grades:", ...) # TODO: print the correct variables print("-" * 45) else: print("WARNING: invalid grade information!") print(f"The grade data `{grade_info}` was not added.")
Test your code
In your second file (see the "Python File 2" instructions in the breakout room lab), add the assert statements for the create_grade_list().
Test create_grade_list() with different strings as arguments, and make the code assert the correct return value.
When you run the following code
result = create_grade_list("90 99 98") print(f"--> create_grade_list('90 99 98') returned `{result}`\n") assert result == [90.0, 99.0, 98.0] result = create_grade_list("100 A A- B") print(f"--> create_grade_list('100 A A- B') returned `{result}`\n") assert result == -1 result = create_grade_list("") print(f"--> create_grade_list(\"\") returned `{result}`\n") assert result == [] result = create_grade_list("95.0 90 60") print(f"--> create_grade_list('95.0 90 60') returned `{result}`\n") assert result == [95.0, 90.0, 60.0]
you should get the following output (note the --> coming from our testing file versus the prints that are part of the function output):
--> create_grade_list('90 99 98') returned `[90.0, 99.0, 98.0]` --> create_grade_list('100 A A- B') returned `-1` --> create_grade_list("") returned `[]` --> create_grade_list('95.0 90 60') returned `[95.0, 90.0, 60.0]`
If you see an AssertionError, check the line number that the error message pointed to. In IDLE, you can select the "Show Line Numbers" option from the top "Options" menu.
Feel free to add a few more tests to check for additional cases.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

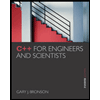
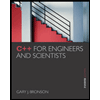