Implement a function insertBook(Book newBook) to add a book at the end of the linked list. a. Ask the librarian to input all the necessary book information. b. Use the information to create a Book object c. Pass through the Book object as the parameter to the function d. Store the Book object into the Node object e. “Link” the object with the rest of the linked list 2. Implement a function insertBefore(Book newBook, int bookID) to add a book in front of another book given its bookID. a. Ask the librarian to input all the necessary book information. b. Use the information to create a Book object c. Pass through the Book object and bookID as the parameter to the function d. Search for the matching Book object e. Store the Book object into the Node object f. “Link” the object with the rest of the linked list 3. Implement a function searchBook(int bookID) to search for a book by its bookID code and return the book object. a. Ask the librarian to input the bookID. b. If the book doesn’t exist display an appropriate message. 4. Implement a function deleteBook(int bookID) to delete a book from the linked list by its bookID code. a. If the book doesn’t exist, please display an appropriate message. 5. Implement a function listAllBooks() to display all the books in the linked list. a. If there are no books, please display an appropriate message 6. Implement a function sortLibrary() to sort all the books in the linked list by its bookID code ascendingly. 7. Implement a function totalBooks() to count the total amount of books in the linked list and return it. ------------------------------------------------------------------------------------------------------------- Driver Code: Write a main method inside the LibraryManagementSystem class, create a menu system that allows the Librarian input commands to interact with the system. Below is the menu system that students should aim to recreate. Give an appropriate message after each action to notify the Librarian if an action was successful. Make use of the toString() to print the Book objects.
1. Implement a function insertBook(Book newBook) to add a book at the end of the
linked list.
a. Ask the librarian to input all the necessary book information.
b. Use the information to create a Book object
c. Pass through the Book object as the parameter to the function
d. Store the Book object into the Node object
e. “Link” the object with the rest of the linked list
2. Implement a function insertBefore(Book newBook, int bookID) to add a book in
front of another book given its bookID.
a. Ask the librarian to input all the necessary book information.
b. Use the information to create a Book object
c. Pass through the Book object and bookID as the parameter to the function
d. Search for the matching Book object
e. Store the Book object into the Node object
f. “Link” the object with the rest of the linked list
3. Implement a function searchBook(int bookID) to search for a book by its bookID
code and return the book object.
a. Ask the librarian to input the bookID.
b. If the book doesn’t exist display an appropriate message.
4. Implement a function deleteBook(int bookID) to delete a book from the linked list by
its bookID code.
a. If the book doesn’t exist, please display an appropriate message.
5. Implement a function listAllBooks() to display all the books in the linked list.
a. If there are no books, please display an appropriate message
6. Implement a function sortLibrary() to sort all the books in the linked list by its bookID
code ascendingly.
7. Implement a function totalBooks() to count the total amount of books in the linked list
and return it.
-------------------------------------------------------------------------------------------------------------
Driver Code:
Write a main method inside the LibraryManagementSystem class, create a menu system
that allows the Librarian input commands to interact with the system. Below is the menu
system that students should aim to recreate. Give an appropriate message after each
action to notify the Librarian if an action was successful. Make use of the toString() to
print the Book objects.

Step by step
Solved in 5 steps with 6 images

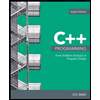
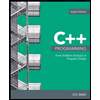