Implement an ADT that can used as a Tic-Tac-Toe game board. Your ADT will implement the interface given below. In the main method also include a simple usage example. Here are the rules of N x N Tic-Tac-Toe game: • Players take turns placing characters into empty squares (" "). • The first player A always places "X" characters, while the second player B always places "O" characters. • "X" and "O" characters are always placed into empty squares, never on filled ones. • The game ends when there are N of the same (non-empty) character filling any row, column, or diagonal. • The game also ends if all squares are non-empty. • No more moves can be played if the game is over Must be outputted in the console not with gui.
Implement an ADT that can used as a Tic-Tac-Toe game board. Your ADT will implement the interface
given below. In the main method also include a simple usage example.
Here are the rules of N x N Tic-Tac-Toe game:
• Players take turns placing characters into empty squares (" ").
• The first player A always places "X" characters, while the second player B always places "O" characters.
• "X" and "O" characters are always placed into empty squares, never on filled ones.
• The game ends when there are N of the same (non-empty) character filling any row, column, or diagonal.
• The game also ends if all squares are non-empty.
• No more moves can be played if the game is over
Must be outputted in the console not with gui.
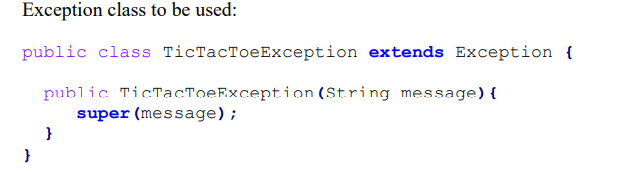
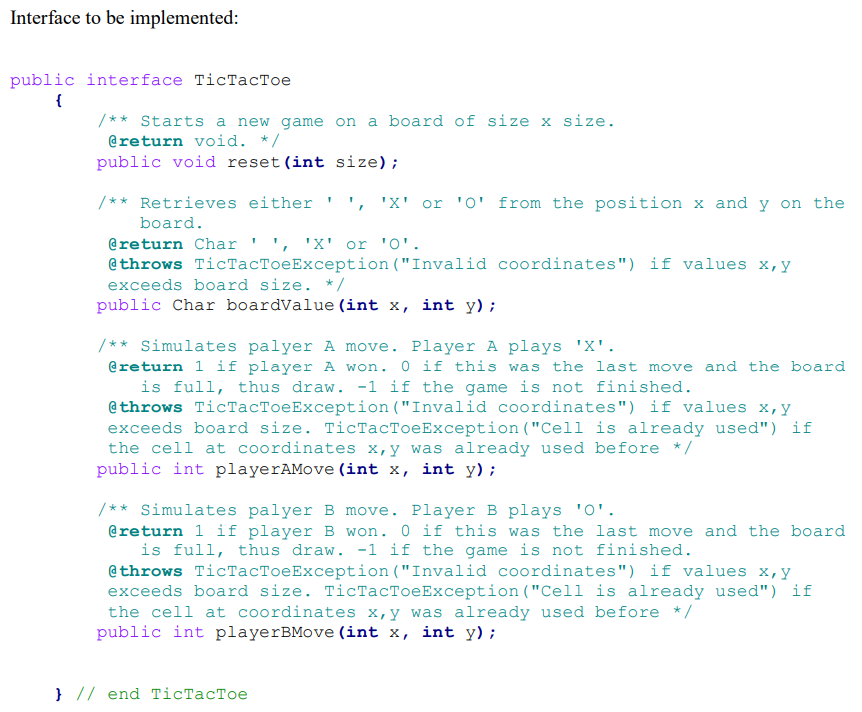

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 1 images

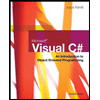
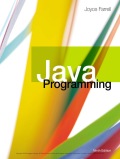
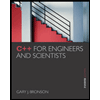
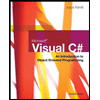
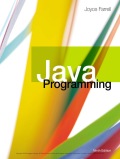
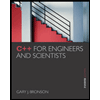