Implement Stack and Queue using LinkList class and compare the performance between StackArray,QueueArray and StackLinkList and QueueLinkList : class LinkList{ private Node first; // ref to first item on list public Node getFirst() // get value of first { return first; } public void setFirst(Node f) // set first to new link { first = f; } public LinkList() // constructor { first = null; } // no items on list yet public boolean isEmpty() // true if list is empty { return (first==null); } public void insertFirst(int dd) { // insert at start of list Node newLink = new Node(dd); // make new link newLink.next = first; // newLink --> old first first = newLink; // first --> newLink } public void insertLast(int dd) { // insert at start of list Node newLink = new Node(dd); // make new link Node current = first; while( current.next != null) { current = current.next; } current.next= newLink; }// end insertLast public int deleteFirst(){ // delete first item (assumes list not empty) Node temp = first; // save reference to link first = first.next; // delete it: first-->old next temp.next = null; return temp.iData; // return deleted link } // ------------------------------------------------------------- public ListIterator getIterator() { // return iterator return new ListIterator(this); // initialized with } // this list // ------------------------------------------------------------- public void displayList() { Node current = first; // start at beginning of list while(current != null) { // until end of list, current.displayLink(); // print data current = current.next; // move to next link } System.out.println(""); } // ------------------------------------------------------------- public Node find(int key) { Node current = first; while ( current != null) { if(current.iData == key) { return current; }//if current= current.next; }// while return null; } public Node delete(int key) { Node prev= first; Node current = first; while ( current != null) { if(current.iData == key) { prev.next = current.next; current.next = null; return current; }//if prev = current; current = current.next; }// while return null; } public Node deleteLast() { Node current = first; Node prev = first; Node temp = null; while(current.next != null ) { prev = current; current = current.next; }// end while temp= current; prev.next = null; return temp; }// end deleteLast // ------------------------------------------------------------- } // end class LinkList class LinkList2App { public static void main(String[] args) { LinkList theList = new LinkList(); // make list theList.insertFirst(22); // insert 4 items theList.insertFirst(44); theList.insertFirst(66); theList.insertFirst(88); theList.displayList(); // display list Node f = theList.find(88); // find item if( f != null) System.out.println("Found link with key " + f.iData); else System.out.println("Can't find link"); Node d = theList.delete(66); // delete item if( d != null ) System.out.println("Deleted link with key " + d.iData); else System.out.println("Can't delete link"); theList.displayList(); // display list theList.deleteLast(); theList.displayList(); theList.deleteFirst(); theList.displayList(); theList.insertLast(33); theList.displayList(); theList.insertLast(80); theList.displayList(); } // end main() } // end class LinkList2App
Implement Stack and Queue using LinkList class and compare the performance between StackArray,QueueArray and StackLinkList and QueueLinkList :
class LinkList{
private Node first; // ref to first item on list
public Node getFirst() // get value of first
{ return first; }
public void setFirst(Node f) // set first to new link
{ first = f; }
public LinkList() // constructor
{ first = null; } // no items on list yet
public boolean isEmpty() // true if list is empty
{ return (first==null); }
public void insertFirst(int dd) { // insert at start of list
Node newLink = new Node(dd); // make new link
newLink.next = first; // newLink --> old first
first = newLink; // first --> newLink
}
public void insertLast(int dd) { // insert at start of list
Node newLink = new Node(dd); // make new link
Node current = first;
while( current.next != null) {
current = current.next;
}
current.next= newLink;
}// end insertLast
public int deleteFirst(){ // delete first item (assumes list not empty)
Node temp = first; // save reference to link
first = first.next; // delete it: first-->old next
temp.next = null;
return temp.iData; // return deleted link
}
// -------------------------------------------------------------
public ListIterator getIterator() { // return iterator
return new ListIterator(this); // initialized with
} // this list
// -------------------------------------------------------------
public void displayList() {
Node current = first; // start at beginning of list
while(current != null) { // until end of list,
current.displayLink(); // print data
current = current.next; // move to next link
}
System.out.println("");
}
// -------------------------------------------------------------
public Node find(int key) {
Node current = first;
while ( current != null) {
if(current.iData == key) {
return current;
}//if
current= current.next;
}// while
return null;
}
public Node delete(int key) {
Node prev= first;
Node current = first;
while ( current != null) {
if(current.iData == key) {
prev.next = current.next;
current.next = null;
return current;
}//if
prev = current;
current = current.next;
}// while
return null;
}
public Node deleteLast() {
Node current = first;
Node prev = first;
Node temp = null;
while(current.next != null ) {
prev = current;
current = current.next;
}// end while
temp= current;
prev.next = null;
return temp;
}// end deleteLast
// -------------------------------------------------------------
} // end class LinkList
class LinkList2App
{
public static void main(String[] args)
{
LinkList theList = new LinkList(); // make list
theList.insertFirst(22); // insert 4 items
theList.insertFirst(44);
theList.insertFirst(66);
theList.insertFirst(88);
theList.displayList(); // display list
Node f = theList.find(88); // find item
if( f != null)
System.out.println("Found link with key " + f.iData);
else
System.out.println("Can't find link");
Node d = theList.delete(66); // delete item
if( d != null )
System.out.println("Deleted link with key " + d.iData);
else
System.out.println("Can't delete link");
theList.displayList(); // display list
theList.deleteLast();
theList.displayList();
theList.deleteFirst();
theList.displayList();
theList.insertLast(33);
theList.displayList();
theList.insertLast(80);
theList.displayList();
} // end main()
} // end class LinkList2App

Step by step
Solved in 2 steps

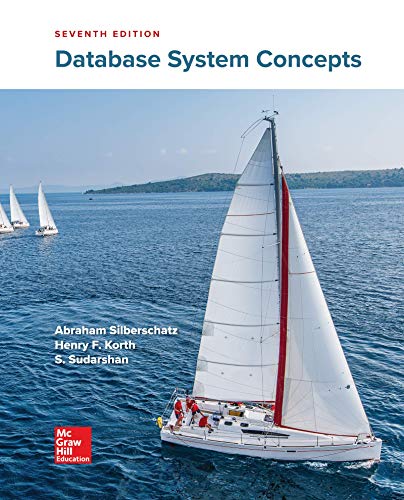
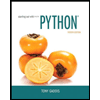
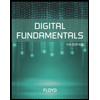
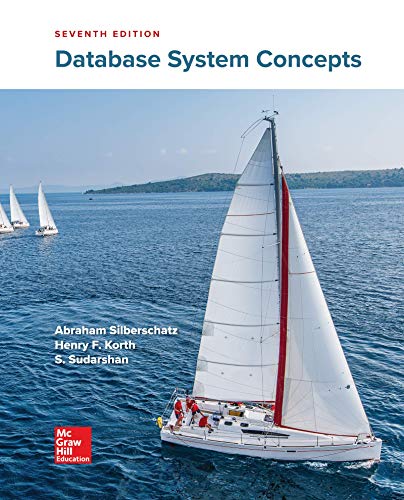
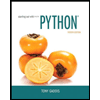
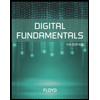
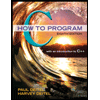
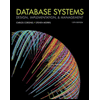
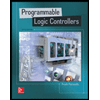