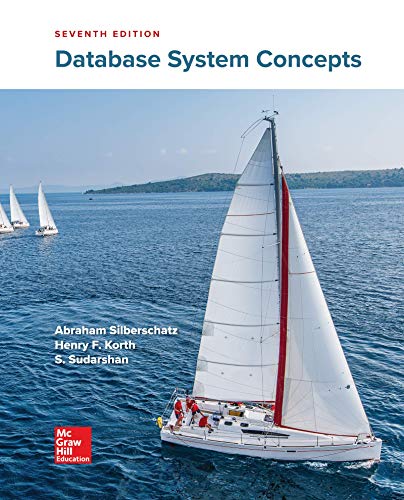
import java.util.LinkedList;
public class IntTree {
private Node root;
private static class Node {
public int key;
public Node left, right;
public Node(int key) {
this.key = key;
}
}
public void printInOrder() {
printInOrder(root);
}
private void printInOrder(Node n) {
if (n == null)
return;
printInOrder(n.left);
System.out.println(n.key);
printInOrder(n.right);
}
/*
* Returns the number of nodes in the tree "above" depth k. Do not include nodes
* at depth k. For example, given BST with level order traversal 50 25 100 12 37
* 150 127 the following values should be returned t.sizeAboveDepth(0) == 0
* t.sizeAboveDepth(1) == 1 [50] t.sizeAboveDepth(2) == 3 [50, 25, 100]
* t.sizeAboveDepth(3) == 6 [50, 25, 100, 12, 37, 150] t.sizeAboveDepth(k) == 7
* for k >= 4 [50, 25, 100, 12, 37, 150, 127]
*/
public int sizeAboveDepth(int h) {
// TODO
throw new RuntimeException("Not implemented");
}
• You are not allowed to use any kind of loop in your solutions.
• You may not modify the Node class in any way
• You may not modify the function headers of any of the functions already present in the file.
• You may not add any fields to the IntTree class.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- import java.util.*; public class Main{ public static void main(String[] args) { Main m = new Main(); m.go(); } private void go() { List<Stadium> parks = new ArrayList<Stadium>(); parks.add(new Stadium("PNC Park", "Pittsburgh", 38362, true)); parks.add(new Stadium("Dodgers Stadium", "Los Angeles", 56000, true)); parks.add(new Stadium("Citizens Bank Park", "Philadelphia", 43035, false)); parks.add(new Stadium("Coors Field", "Denver", 50398, true)); parks.add(new Stadium("Yankee Stadium", "New York", 54251, false)); parks.add(new Stadium("AT&T Park", "San Francisco", 41915, true)); parks.add(new Stadium("Citi Field", "New York", 41922, false)); parks.add(new Stadium("Angels Stadium", "Los Angeles", 45050, true)); Collections.sort(parks, Stadium.ByKidZoneCityName.getInstance()); for (Stadium s : parks) System.out.println(s); }}…arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forward
- Draw a UML class diagram for the following code: import java.util.*; public class QueueOperationsDemo { public static void main(String[] args) { Queue<String> linkedListQueue = new LinkedList<>(); linkedListQueue.add("Apple"); linkedListQueue.add("Banana"); linkedListQueue.add("Cherry"); System.out.println("Is linkedListQueue empty? " + linkedListQueue.isEmpty()); System.out.println("Front element: " + linkedListQueue.peek()); System.out.println("Removed element: " + linkedListQueue.remove()); System.out.println("Front element after removal: " + linkedListQueue.peek()); Queue<Integer> arrayDequeQueue = new ArrayDeque<>(); arrayDequeQueue.add(10); arrayDequeQueue.add(20); arrayDequeQueue.add(30); System.out.println("Is arrayDequeQueue empty? " + arrayDequeQueue.isEmpty()); System.out.println("Front element: " + arrayDequeQueue.peek());…arrow_forwardimport java.util.*;import java.io.*; public class HuffmanCode { private Queue<HuffmanNode> queue; private HuffmanNode overallRoot; public HuffmanCode(int[] frequencies) { queue = new PriorityQueue<HuffmanNode>(); for (int i = 0; i < frequencies.length; i++) { if (frequencies[i] > 0) { HuffmanNode node = new HuffmanNode(frequencies[i]); node.ascii = i; queue.add(node); } } overallRoot = buildTree(); } public HuffmanCode(Scanner input) { overallRoot = new HuffmanNode(-1); while (input.hasNext()) { int asciiValue = Integer.parseInt(input.nextLine()); String code = input.nextLine(); overallRoot =…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
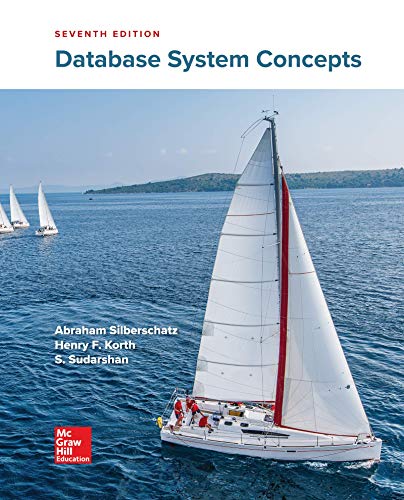
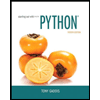
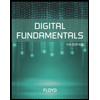
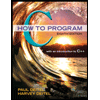
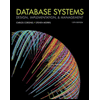
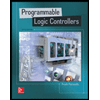