Implement the Solver class. The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of bytes and returns a byte array containing a minimal sequence of moves that will lead to the solved board. The 2D array of bytes is “triangular” and represents a valid board configuration. Namely, the 2D array has 5 rows (0 – 4) and the size of every row is 1 more than its index.The array contains one 0, five 1s, four 2s and five 3s. The solve method then returns an array of bytes representing a minimal sequence of moves that solves the puzzle. In other words, if the numbers from the returned array are used in order as inputs to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned. import java.util.Arrays; public class Solver { public Solver() { // TODO // This constructor will be called once and then // solve will be called multiple times. So if // you want to do some preprocessing ONCE before // solve is called, you can do it here. } public byte[] solve(byte[][] start) { // TODO // The 2D array start will have the same format as // the Board constructor from p1 so you can reuse // your Board class from p1, although you might // need to make some modifications to it. // // This method should return a minimal sequence of // moves that would change the given board to the // solved board. throw new RuntimeException("Not implemented"); } } Note: Do not change the method public byte[ ] solve. You are supposed to use byte, not char.
Implement the Solver class. The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of bytes and returns a byte array containing a minimal sequence of moves that will lead to the solved board. The 2D array of bytes is “triangular” and represents a valid board configuration. Namely, the 2D array has 5 rows (0 – 4) and the size of every row is 1 more than its index.The array contains one 0, five 1s, four 2s and five 3s. The solve method then returns an array of bytes representing a minimal sequence of moves that solves the puzzle. In other words, if the numbers from the returned array are used in order as inputs to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned.

Step by step
Solved in 3 steps

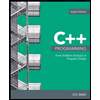
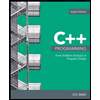