Implementing a rainbow table You are to write a program, $ ./Rainbow Passwords.txt that should run using the following instruction: where the file Passwords.txt contains a list of possible passwords. The password file contains a password per line, as in the provided words file and consists of strings of printable characters. Any password used must be taken from this file, so the only stored hash information needs to relate to those entries in the file. The program is used to find pre-images for given hash values. Rainbow tables can be used to solve pre-image problems for hash functions. At the simplest level they can simply be a list of hash values and the corresponding pre-images, often from some dictionary. This can be expensive in terms of storage space however, and a more efficient way of identifying pre-images involves the use of the hash function and reduction functions. First step The process is as follows: 1. Read in the list of possible passwords. Report on the number of words read in. 2. For each previously unused word W, first mark it as used and then carry out the following process : Your program will do some initial computations to generate the rainbow table. (a) Apply the hash function H to the word W to produce a hash value H(W), which we refer to as the current hash. (b) Apply the reduction function R to the current hash, which will give a different possible password which should be marked as used and then hashed. The resulting hash value is recorded as the current hash. (c) Repeat the previous step four times. You can deal with collisions if you like but are not required to. (d) Store the original word W and the final current hash as an entry in your rainbow table. 3. To assist with the later identification of the pre-images you should sort the rainbow table based on the hash values 4. Output the list of words and corresponding "final current hashes" to a text file Rainbow.txt. Report. to standard out the number of lines in your rainbow table Second Step : You are now ready to carry out the second part of the exercise, finding pre- images. Request a hash value from the user. There should be appropriate error checking as to the length of the input string etc. The process of identifying the pre-image for the provided hash value is sketched as follows: 1. Check if the hash value is in the rainbow table 2. If the hash value isn't in the rainbow table you reduce and hash until you get a hash value that is in the rainbow table, or until you have done the reduce and hash enough times that it's clear from the way the rainbow table is generated that something is wrong. Display an appropriate message if this happens 3. Once you have identified the relevant hash value in the table you take the corresponding password and hash it. If that is your hash value you have your pre-image. If it isn't, then reduce and hash again, until the reduced word hashes to the hash value being searched for 4. Output the releative reduced word, that is your pre-image A reduction function is designed to take a valid hash value and return a valid password, in this case a valid word from Passwords.txt. The reduction function to be used should be based on the number of words in the Passwords.txt file, and since that isn't a fixed length file your method needs to be dynamic. You should find a way to convert a hash value to an integer that identifies one of the passwords. You can define yourself a reduction function R or find some examples online. You should describe how your reduction function works in readme.txt. You should use MD5 as the hash function. You don't need to implement the MD5 hash function by yourself. You can find such a file online. Provide a reference if you use any open source implementation.
Implementing a rainbow table You are to write a program, $ ./Rainbow Passwords.txt that should run using the following instruction: where the file Passwords.txt contains a list of possible passwords. The password file contains a password per line, as in the provided words file and consists of strings of printable characters. Any password used must be taken from this file, so the only stored hash information needs to relate to those entries in the file. The program is used to find pre-images for given hash values. Rainbow tables can be used to solve pre-image problems for hash functions. At the simplest level they can simply be a list of hash values and the corresponding pre-images, often from some dictionary. This can be expensive in terms of storage space however, and a more efficient way of identifying pre-images involves the use of the hash function and reduction functions. First step The process is as follows: 1. Read in the list of possible passwords. Report on the number of words read in. 2. For each previously unused word W, first mark it as used and then carry out the following process : Your program will do some initial computations to generate the rainbow table. (a) Apply the hash function H to the word W to produce a hash value H(W), which we refer to as the current hash. (b) Apply the reduction function R to the current hash, which will give a different possible password which should be marked as used and then hashed. The resulting hash value is recorded as the current hash. (c) Repeat the previous step four times. You can deal with collisions if you like but are not required to. (d) Store the original word W and the final current hash as an entry in your rainbow table. 3. To assist with the later identification of the pre-images you should sort the rainbow table based on the hash values 4. Output the list of words and corresponding "final current hashes" to a text file Rainbow.txt. Report. to standard out the number of lines in your rainbow table Second Step : You are now ready to carry out the second part of the exercise, finding pre- images. Request a hash value from the user. There should be appropriate error checking as to the length of the input string etc. The process of identifying the pre-image for the provided hash value is sketched as follows: 1. Check if the hash value is in the rainbow table 2. If the hash value isn't in the rainbow table you reduce and hash until you get a hash value that is in the rainbow table, or until you have done the reduce and hash enough times that it's clear from the way the rainbow table is generated that something is wrong. Display an appropriate message if this happens 3. Once you have identified the relevant hash value in the table you take the corresponding password and hash it. If that is your hash value you have your pre-image. If it isn't, then reduce and hash again, until the reduced word hashes to the hash value being searched for 4. Output the releative reduced word, that is your pre-image A reduction function is designed to take a valid hash value and return a valid password, in this case a valid word from Passwords.txt. The reduction function to be used should be based on the number of words in the Passwords.txt file, and since that isn't a fixed length file your method needs to be dynamic. You should find a way to convert a hash value to an integer that identifies one of the passwords. You can define yourself a reduction function R or find some examples online. You should describe how your reduction function works in readme.txt. You should use MD5 as the hash function. You don't need to implement the MD5 hash function by yourself. You can find such a file online. Provide a reference if you use any open source implementation.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Pls code use c++ and provide explanation about the hash function used
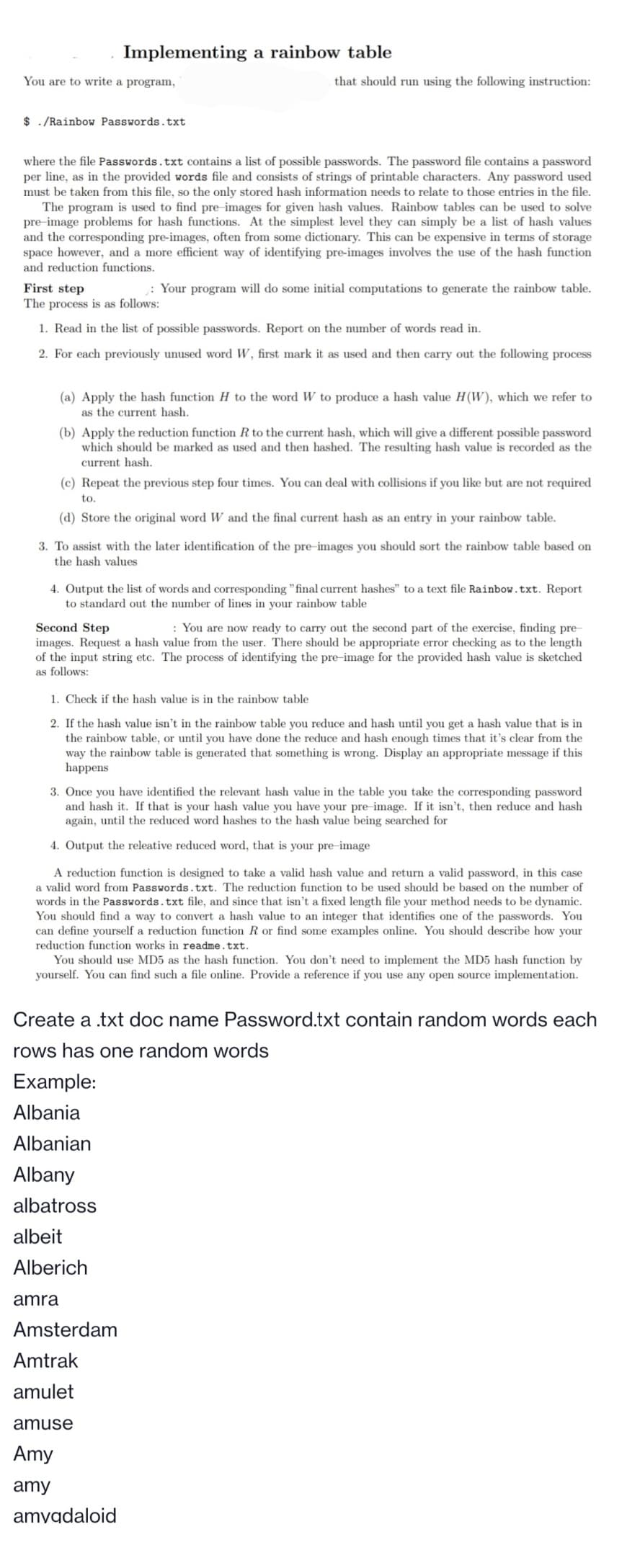
Transcribed Image Text:You are to write a program,
$ ./Rainbow Passwords.txt
where the file Passwords.txt contains a list of possible passwords. The password file contains a password
per line, as in the provided words file and consists of strings of printable characters. Any password used
must be taken from this file, so the only stored hash information needs to relate to those entries in the file.
The program is used to find pre-images for given hash values. Rainbow tables can be used to solve
pre-image problems for hash functions. At the simplest level they can simply be a list of hash values
and the corresponding pre-images, often from some dictionary. This can be expensive in terms of storage
space however, and a more efficient way of identifying pre-images involves the use of the hash function
and reduction functions.
Implementing a rainbow table
First step
The process is as follows:
1. Read in the list of possible passwords. Report on the number of words read in.
2. For each previously unused word W, first mark it as used and then carry out the following process
that should run using the following instruction:
(a) Apply the hash function H to the word W to produce a hash value H(W), which we refer to
as the current hash.
(b) Apply the reduction function R to the current hash, which will give a different possible password
which should be marked as used and then hashed. The resulting hash value is recorded as the
current hash.
(c) Repeat the previous step four times. You can deal with collisions if you like but are not required
to.
(d) Store the original word W and the final current hash as an entry in your rainbow table.
3. To assist with the later identification of the pre-images you should sort the rainbow table based on
the hash values
: Your program will do some initial computations to generate the rainbow table.
4. Output the list of words and corresponding "final current hashes" to a text file Rainbow.txt. Report
to standard out the number of lines in your rainbow table
Second Step
: You are now ready to carry out the second part of the exercise, finding pre-
images. Request a hash value from the user. There should be appropriate error checking as to the length
of the input string etc. The process of identifying the pre-image for the provided hash value is sketched
as follows:
1. Check if the hash value is in the rainbow table
2. If the hash value isn't in the rainbow table you reduce and hash until you get a hash value that is in
the rainbow table, or until you have done the reduce and hash enough times that it's clear from the
way the rainbow table is generated that something is wrong. Display an appropriate message if this
happens
amra
3. Once you have identified the relevant hash value in the table you take the corresponding password
and hash it. If that is your hash value you have your pre-image. If it isn't, then reduce and hash
again, until the reduced word hashes to the hash value being searched for
4. Output the releative reduced word, that is your pre-image
Example:
Albania
Albanian
Albany
albatross
A reduction function is designed to take a valid hash value and return a valid password, in this case
a valid word from Passwords.txt. The reduction function to be used should be based on the number of
words in the Passwords.txt file, and since that isn't a fixed length file your method needs to be dynamic.
You should find a way to convert a hash value to an integer that identifies one of the passwords. You
can define yourself a reduction function R or find some examples online. You should describe how your
reduction function works in readme.txt.
You should use MD5 as the hash function. You don't need to implement the MD5 hash function by
yourself. You can find such a file online. Provide a reference if you use any open source implementation.
albeit
Alberich
Create a .txt doc name Password.txt contain random words each
rows has one random words
Amsterdam
Amtrak
amulet
amuse
Amy
amy
amygdaloid
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 1 images

Recommended textbooks for you
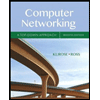
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
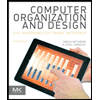
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
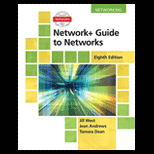
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
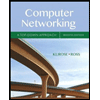
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
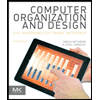
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
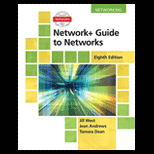
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
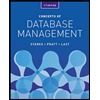
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
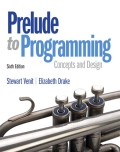
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
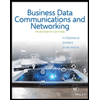
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY