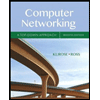
Java:
Yellow highlighted columns are outer ‘for’ loop
Blue highlighted columns are inner ‘for’ loop
![### Insertion Sort: Step-by-Step Explanation
#### Original Array:
- `{150, 8, 55, 78, 91, 10}`
#### Table Explanation for Insertion Sort Algorithm:
This table guides you through the process of the insertion sort algorithm by following specific steps illustrated in columns. Each step represents an iteration of the inner workings of the algorithm.
1. **Column 1: `i < list.length`**
- This condition checks if the current index `i` is less than the length of the list. If true, the algorithm proceeds to the next steps.
2. **Column 2: `CE = list[i]`**
- The current element (CE) is set to the value of the list at index `i`.
3. **Column 3: `k = i - 1`**
- `k` is initialized to the index one less than `i`.
4. **Column 4: `(list[k] > CE) && (k >= 0)`**
- This condition checks if the element at index `k` is greater than the current element `CE` and ensures `k` is not negative.
5. **Column 5: `list[k+1] = list[k]; k--`**
- If the above condition is true, shift the element at index `k` to the right (index `k+1`) and decrement `k`.
6. **Column 6: `list[k+1] = CE; i++`**
- Place the current element `CE` in its correct position (index `k+1`). Increment `i` to proceed to the next element in the array.
The table is structured to demonstrate each step iteratively as the insertion sort algorithm processes the original array. This allows an educational exploration of how elements are compared and shifted to achieve a sorted sequence.](https://content.bartleby.com/qna-images/question/b64c1c6f-d4d7-4d8b-8ebe-5eadb49feddc/08bdfb16-2d0b-4c6c-a645-e68fb28fbb8a/qsrasz_thumbnail.png)

Insertion Sort:
i<list.length | CE=list[i] | k=i-1 | (list[k] > CE && (k>=0) |
list[k+1]= list[k]; k--; |
list[k+1]=CE; i++; |
Processing record in position 1 | CE= 8 | k=0 | Move the record to the left until it reaches the correct position. |
Swap list[1]=8; list[0]=150; ist[1]=list[0]; |
list[1]=150; 8,150,55, 78, 91, 10 |
Processing record in position 2 | CE=150 | k=1 | Move the record to the left until it reaches the correct position. |
Swap list[2]=55; list[1]=150; ist[2]=list[1]; |
list[2]=150 8 ,55,150, 78, 91, 10 |
Processing record in position 3 | CE=78 | k=2 | Move the record to the left until it reaches the correct position. |
Swap list[3]=78; list[2]=150; ist[3]=list[2]; |
list[3]=150 8 ,55, 78,150, 91, 10 |
Processing record in position 4 | CE=91 | k=3 | Move the record to the left until it reaches the correct position. |
Swap list[4]=91; list[3]=150; ist[4]=list[3]; |
list[4]=150 8 ,55, 78, 91,150, 10 |
Processing record in position 5 | CE=10 | k=4 | Move the record to the left until it reaches the correct position. |
Swap list[5]=10; list[3]=150; ist[5]=list[4]; |
ist[5]=150 8 ,55, 78, 91, 10,150 |
k=3 ,CE=10 Run Loop Till (list[k] > CE && (k>=0) |
Swap list[4]=10; list[3]=91; ist[3]=list[4]; |
8 ,55, 78, 10, 91,150 | |||
k=2 ,CE=10 Run Loop Till (list[k] > CE && (k>=0) |
Swap list[2]=10; list[1]=55; ist[5]=list[4]; |
8 ,55, 10,78,91,150 | |||
k=1 ,CE=10 Run Loop Till (list[k] > CE && (k>=0) |
Swap list[5]=10; list[3]=150; ist[5]=list[4]; |
8 ,10,55, 78, 91,150 | |||
condition false | condition false |
|
Step by stepSolved in 2 steps

- Computer Science JAVA #7 - program that reads the file named randomPeople.txt sort all the names alphabetically by last name write all the unique names to a file named namesList.txt , there should be no repeatsarrow_forwardC#arrow_forwardSYNTAX ERROR HELP - PYTHON This also happens for several other 'return result' lines in the code. import randomdef rollDice(): num1 = random.randint(1, 6) num2 = random.randint(1, 6) return num1, num2def determine_win_or_lose(num1, num2): result = -1 total = num1 + num2print(f"You rolled {num1} + {num2} = {total}")if total == 2 or total == 3 or total == 12: result = 0elif total == 7 or total == 11: result = 1else: print(f"point is {total}") x = determinePointValueResult(total)if x == 1: result = 1else: result = 0 return resultdef determinePointValueResult(pointValue): total = 0 result = -1while total != 7 and total != pointValue: num1, num2 = rollDice() total = num1 + num2if total == pointValue: result = 1elif total == 7: result = 0print(f"You rolled {num1} + {num2} = {total}")return resultwhile i < n: num1, num2 = rollDice() result = determine_win_or_lose(num1, num2)if result == 1: winCounter += 1 print("You…arrow_forward
- int stop = 6; int num =6; int count=0; for(int i = stop; i >0; i-=2) { num += i; count++; } System.out.println("num = "+ num); System.out.println("count = "+ count); } }arrow_forwardC#Write a method MyMethod which takes an int parameter named x. This method calculates the sum of integers from 1 to x and returns the sum. Use a for loop.arrow_forwardTAKE A LIST OF STRING FROM THE USER AND COUNT THE NUMBER OF UNIQUE ELEMENTS WITHOUT USING THE FOR, WHILE, Do-WHILE LOOPS. PREFERRED PROGRAMMING LANGUAGE: JAVA/C++arrow_forward
- int x1 = 66; int y1 = 39; int d; _asm { } mov EAX, X1; mov EBX, y1; push EAX; push EBX; pop ECX mov d, ECX; What is d in decimal format?arrow_forwardC++ Coding: Arrays Implement a two-dimensional character array. Use a nested loop to store a 12x6 flag. 5 x 2 stars as * Alternate = and – to represent stripe colors Use a nested loop to output the character array to the console.arrow_forwardUsing jGRASP please de-bug this /**This program demonstrates an array of String objects.It should print out the days and the number of hours spent at work each day.It should print out the day of the week with the most hours workedIt should print out the average number of hours worked each dayThis is what should display when the program runs as it shouldSunday has 12 hours worked.Monday has 9 hours worked.Tuesday has 8 hours worked.Wednesday has 13 hours worked.Thursday has 6 hours worked.Friday has 4 hours worked.Saturday has 0 hours worked.Highest Day is Wednesday with 13 hours workedAverage hours worked in the week is 7.43 hours*/{public class WorkDays{public static void main(String[] args){String[] days = { "Sunday", "Monday", "Tuesday","Wednesday", "Thursday", "Friday", "Saturday"}; int[] hours = { 12, 9, 8, 13, 6, 4}; int average = 0.0;int highest = 0;String highestDay = " ";int sum = 0; for (int index = 0; index < days.length; index++){System.out.println(days[index] + " has "…arrow_forward
- Tic tac game in JAVAarrow_forwardJava please Line is a String object that holds an unknown number of int values separated by spaces. Write a segment of code that computes and displays the sum of the values in line.arrow_forwardDesign and write a modular python program for chapter 7 programming exercise #12 from your textbook, with the following modifications: 1. prompt for input of a positive number and call a function to determine if all the numbers from 2 up to and including the input number is a prime or composite number 2. display all the numbers tested with the appropriate label (prime or composite) 3. after completing the task of displaying the numbers the program should ask for a second number input 4. entry of a negative number will end the program Be sure to use clear prompts/labeling for input and output.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
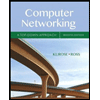
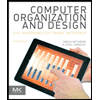
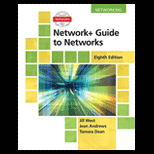
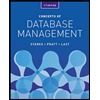
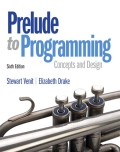
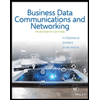